Search Blogs
15 Advanced REST API Interview Questions for Developers
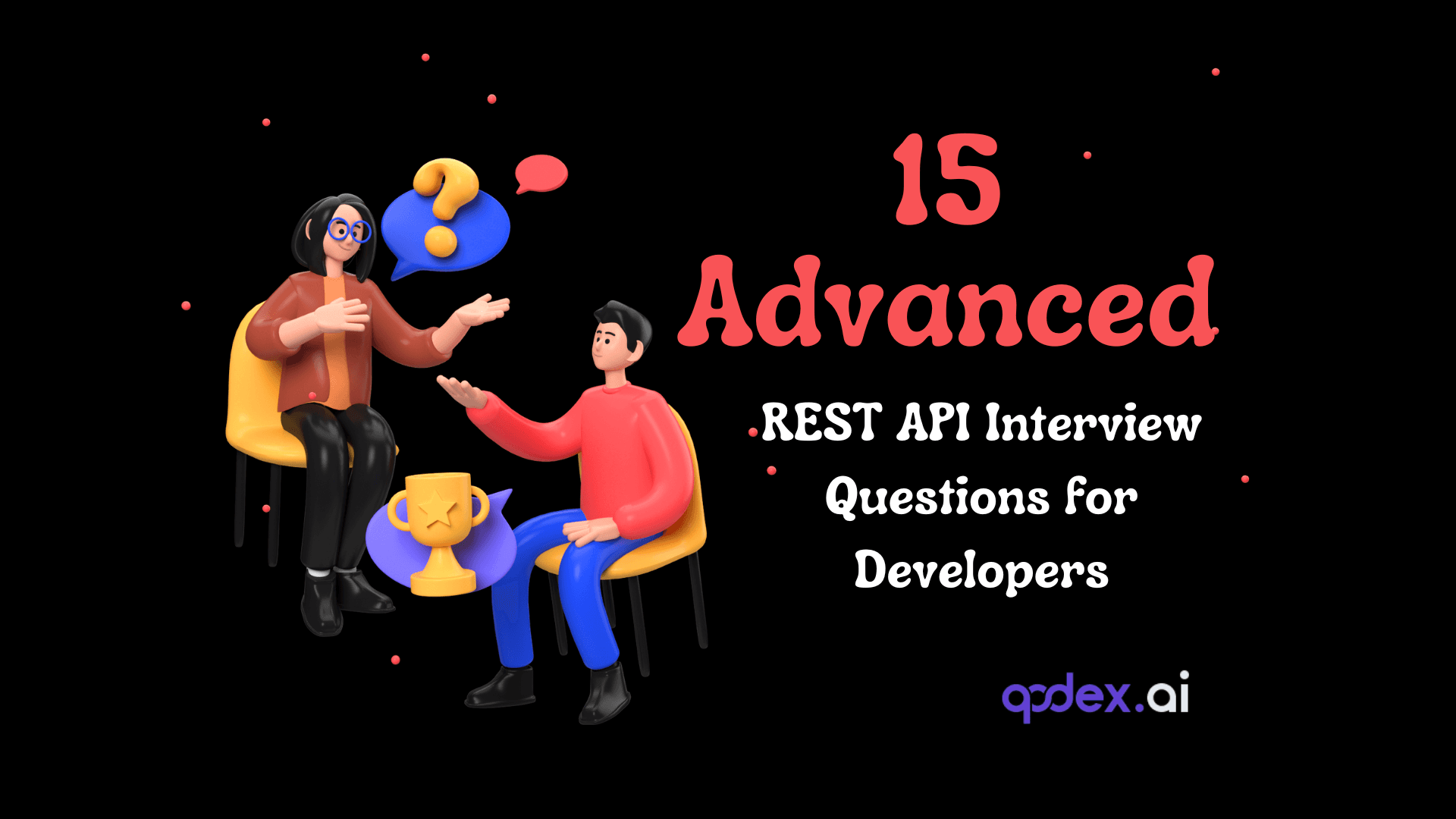
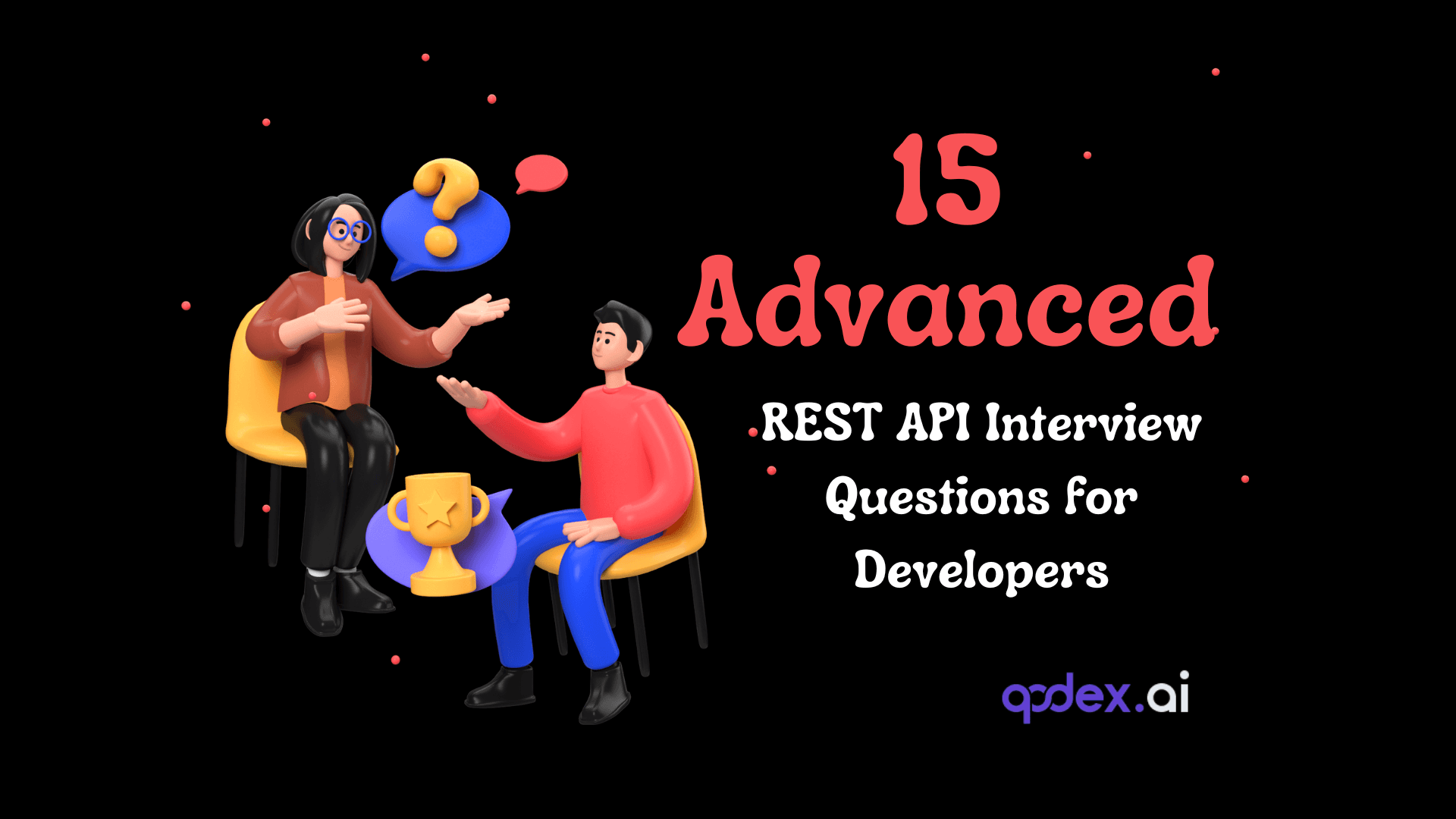
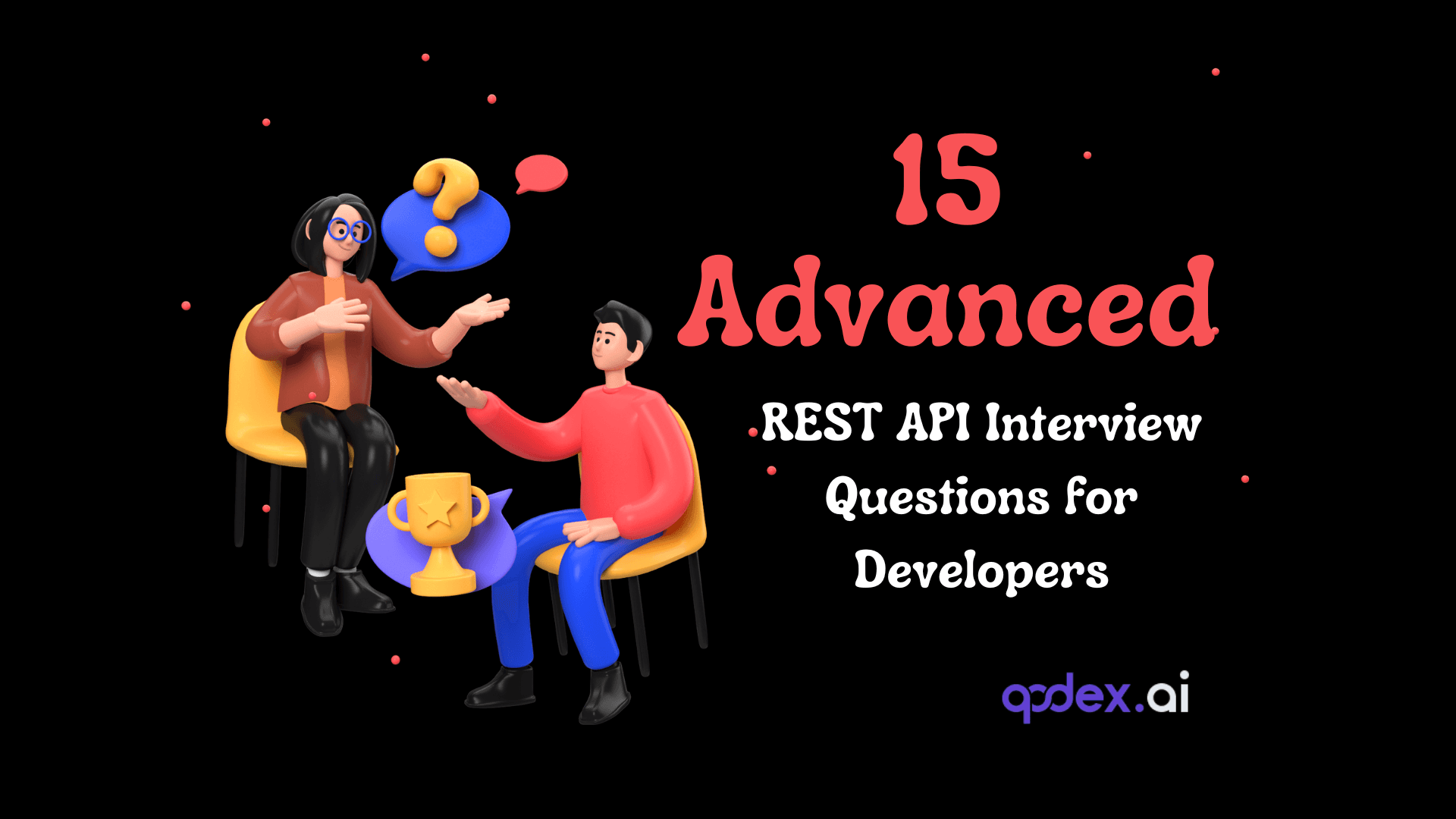
Experienced Interview Questions
Differentiate between SOAP and REST?
SOAP (Simple Object Access Protocol):Protocol: SOAP is a protocol, providing a set of rules for structuring messages, defining endpoints, and describing operations.
Message Format: SOAP messages are typically XML-based, with a rigid structure defined by XML schemas.
Communication Style: SOAP relies on a contract-based communication style, where clients and servers agree on the format and structure of messages beforehand.
Stateful: SOAP supports stateful communication, allowing interactions that maintain session state between client and server.
Complexity: SOAP is considered more complex due to its strict message format and contract-based communication.
REST (Representational State Transfer):
Architectural Style: REST is an architectural style, emphasizing stateless communication and resource-based interactions.
Message Format: REST messages are typically represented in formats like JSON or XML, but the structure is flexible and not mandated by the protocol.
3. Communication Style: RESTful services follow a resource-based communication style, where clients interact with resources using standard HTTP methods.
4. Stateless: REST is stateless, meaning each request from a client to the server contains all the information needed to understand and fulfill the request.
5. Simplicity: REST is considered simpler than SOAP due to its lightweight communication style and flexible message format.
2. While creating URI for web services, what are the best practices that needs to be followed?
List of the best practices that need to be considered with designing URI for web services:
While defining resources, use plural nouns. (Example: To identify user resource, use the name “users” for that resource.)
While using the long name for resources, use underscore or hyphen. Avoid using spaces between words. (Example, to define authorized users resource, the name can be “authorized_users” or “authorized-users”.)
The URI is case-insensitive, but as part of best practice, it is recommended to use lower case only.
While developing URI, the backward compatibility must be maintained once it gets published.
When the URI is updated, the older URI must be redirected to the new one using the HTTP status code 300.
Use appropriate HTTP methods like GET, PUT, DELETE, PATCH, etc. It is not needed or recommended to use these method names in the URI. (Example: To get user details of a particular ID, use /users/{id} instead of /getUser)
Use the technique of forward slashing to indicate the hierarchy between the resources and the collections. (Example: To get the address of the user of a particular id, we can use: /users/{id}/address)
3. What are the best practices to develop RESTful web services?
Best practices for developing RESTful web services include:
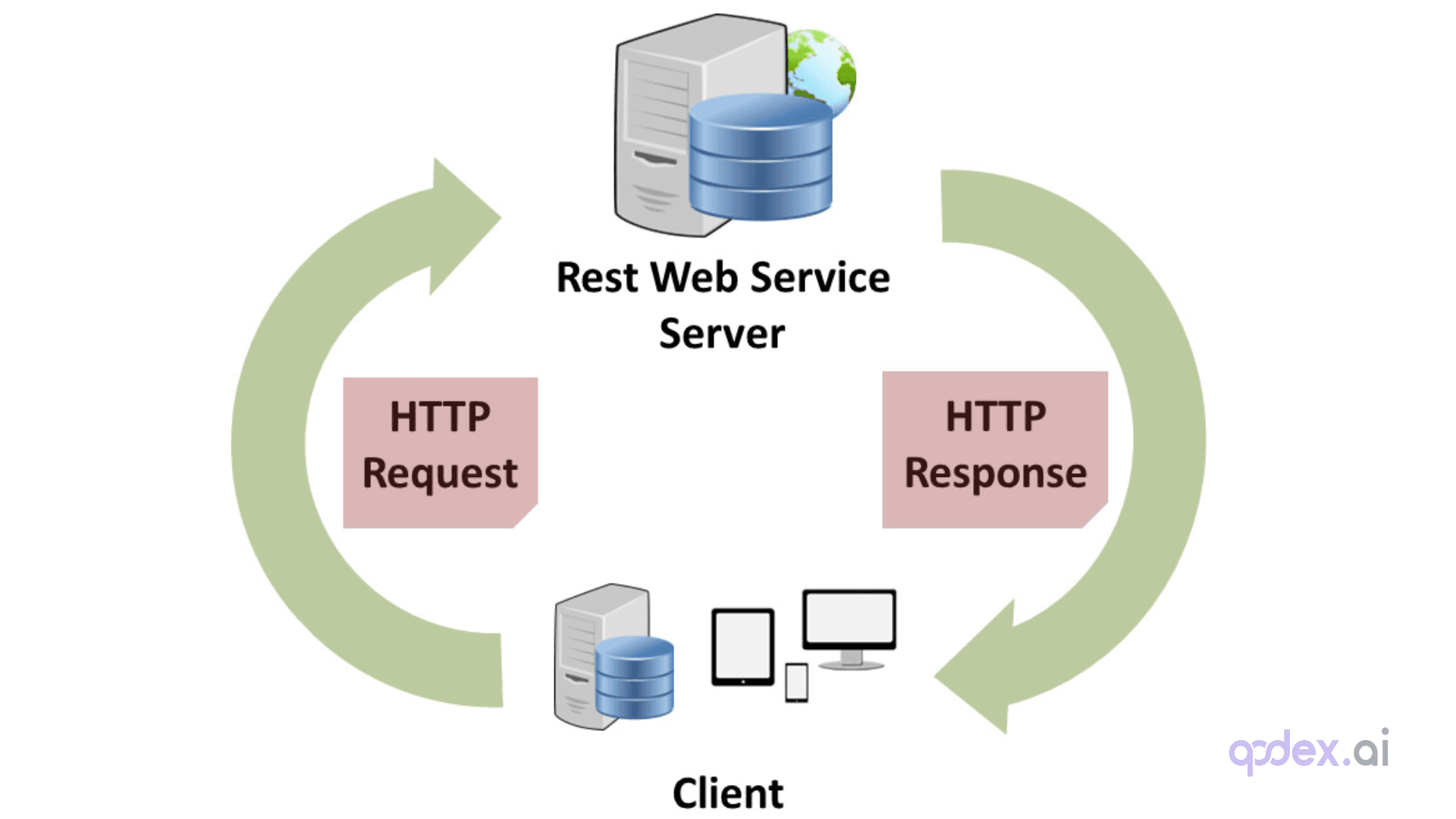
1. Use Descriptive URIs: URIs should be meaningful and descriptive of the resource they represent.
2. Follow HTTP Methods: Use appropriate HTTP methods (GET, POST, PUT, DELETE) to perform CRUD operations on resources.
3. Use HTTP Status Codes: Return relevant HTTP status codes to indicate the outcome of requests (e.g., 200 for success, 404 for not found).
4. Versioning: Implement versioning in URIs or headers to manage changes in APIs over time.
5. Input Validation: Validate input data to ensure security and prevent errors.
6. Error Handling: Provide informative error messages and handle errors gracefully.
7. Use Content Negotiation: Support multiple data formats (JSON, XML) through content negotiation.
8. Security: Implement authentication and authorization mechanisms to secure your APIs.
9. Caching: Use caching mechanisms to improve performance and reduce server load.
10. Documentation: Provide comprehensive documentation for your APIs, including usage examples and explanations of resources and endpoints.
4. What are Idempotent methods? How is it relevant in RESTful web services domain?
Idempotent methods are HTTP methods that can be safely repeated multiple times without causing different outcomes. In other words, performing the same idempotent operation multiple times produces the same result as performing it once.
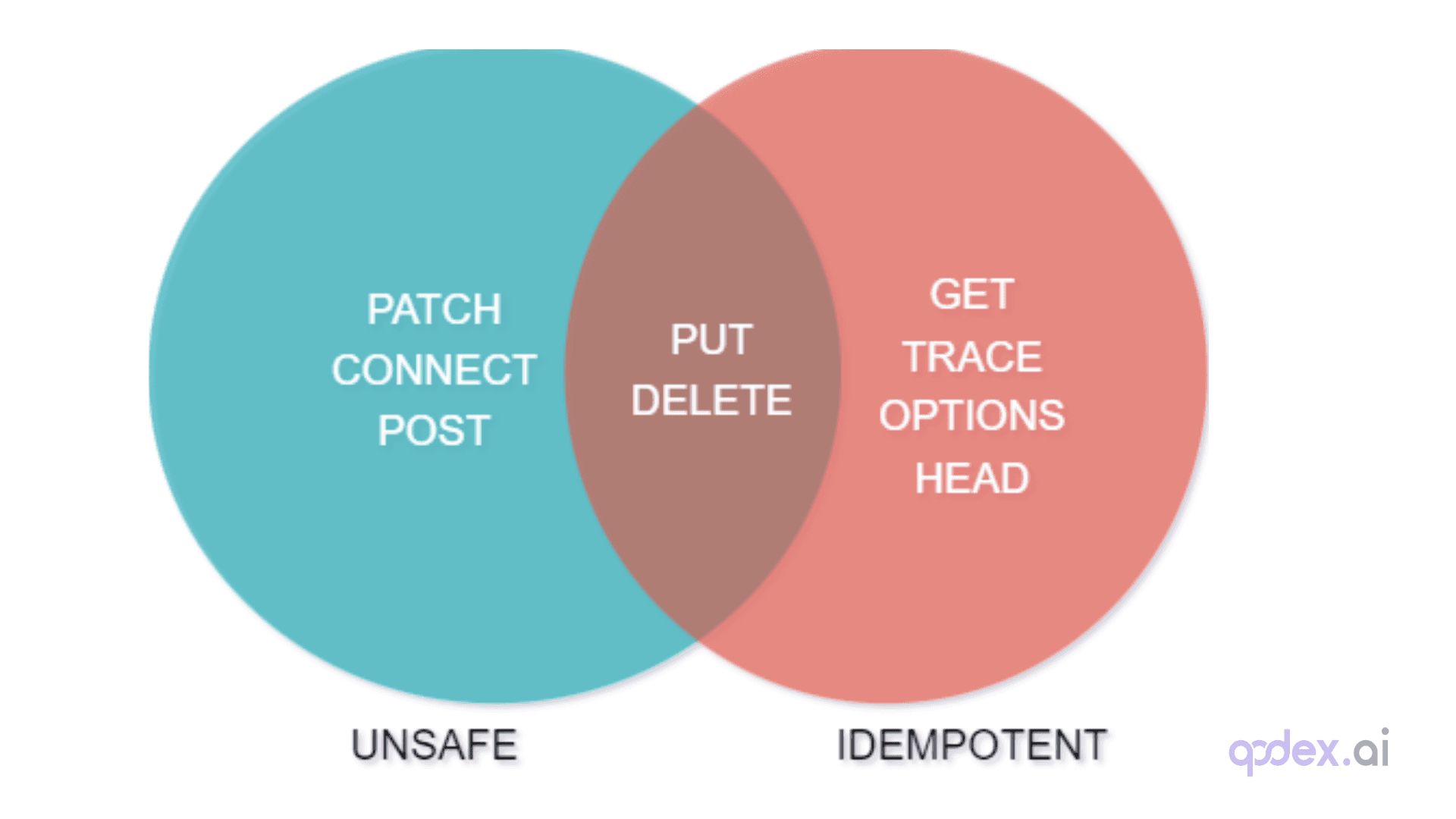
In the context of RESTful web services:
- Idempotent Methods: GET, PUT, and DELETE are considered idempotent methods in HTTP.
- Relevance in RESTful Web Services: Idempotent methods are crucial in RESTful web services because they ensure that repeating requests for resource retrieval (GET), creation or update (PUT), and deletion (DELETE) don't produce unintended side effects. This property simplifies error handling, enhances reliability, and improves scalability in distributed systems.
5. What are the differences between REST and AJAX?
REST vs AJAX:
1. Definition:
- REST (Representational State Transfer) is an architectural style for designing networked applications, emphasising stateless client-server communication via standardised HTTP methods.
- AJAX (Asynchronous JavaScript and XML) is a technique used in web development to create interactive and dynamic user interfaces, allowing asynchronous data retrieval from a server without refreshing the entire page.
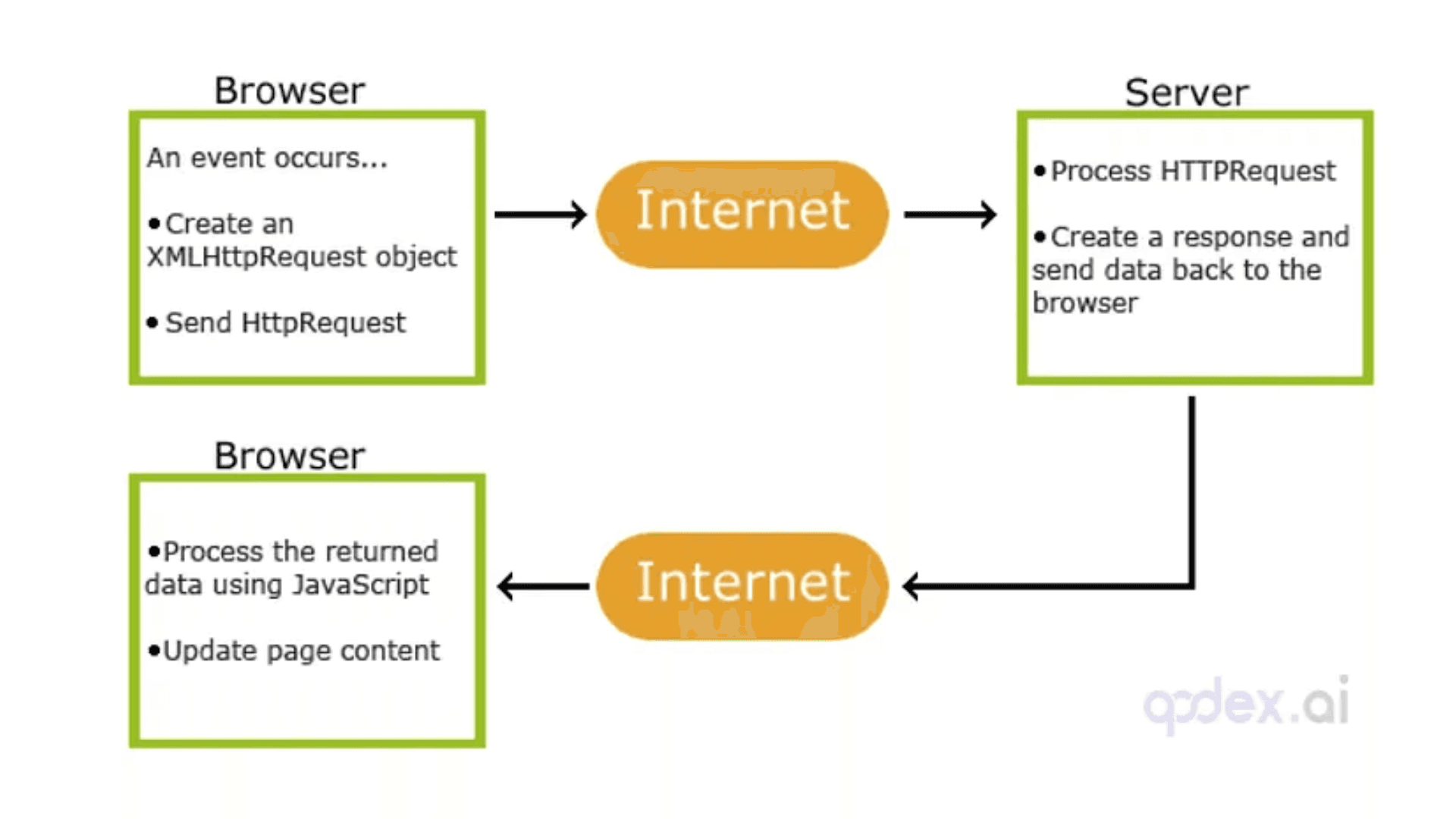
2. Purpose:
- REST is primarily used for designing web services and APIs that enable communication between client and server, typically for accessing and manipulating resources.
- AJAX is used for enhancing user experience by enabling seamless data retrieval and updating within a web page, without requiring a full page reload.
3. Communication Style:
- REST follows a stateless, resource-based communication style, where clients interact with resources via standardised HTTP methods (GET, POST, PUT, DELETE).
- AJAX allows asynchronous communication between client and server, typically using JavaScript to make HTTP requests in the background and update parts of a web page dynamically.
4. Scope:
- REST is more focused on defining the architecture and communication protocols for web services and APIs.
- AJAX is focused on the client-side implementation of web applications, particularly for handling dynamic content and user interactions.
5. Usage:
- REST is commonly used in web development for building APIs and web services that enable interoperability and data exchange between different systems.
- AJAX is widely used in web development for creating responsive and interactive user interfaces, such as updating content without page reloads, form validation, and real-time data fetching.
6. Can you tell what constitutes the core components of HTTP Request?
In REST, any HTTP Request has 5 main components, they are:
Method/Verb − This part tells what methods the request operation represents. Methods like GET, PUT, POST, DELETE, etc are some examples.
URI − This part is used for uniquely identifying the resources on the server.HTTP Version − This part indicates what version of HTTP protocol you are using. An example can be HTTP v1.1.
Request Header − This part has the details of the request metadata such as client type, the content format supported, message format, cache settings, etc.
Request Body − This part represents the actual message content to be sent to the server.
7. What constitutes the core components of HTTP Response?
HTTP Response has 4 components:
Response Status Code − This represents the server response status code for the requested resource. Example- 400 represents a client-side error, 200 represents a successful response.
HTTP Version − Indicates the HTTP protocol version.
Response Header − This part has the metadata of the response message. Data can describe what is the content length, content type, response date, what is server type, etc.
Response Body − This part contains what is the actual resource/message returned from the server.
8. Define Addressing in terms of RESTful Web Services.
Addressing is the process of locating a single/multiple resources that are present on the server. This task is accomplished by making use of URI (Uniform Resource Identifier). The general format of URI is <protocol>://<application-name>/<type-of-resource>/<id-of-resource>
9. What are the differences between PUT and POST in REST?
PUT:
- Purpose: Used to update or replace an existing resource or create a new resource if it doesn't exist.
- Idempotent: PUT requests are idempotent, meaning multiple identical requests have the same effect as a single request.
- Usage: Typically used when the client knows the exact URI of the resource it wants to update or create.
POST:
- Purpose: Used to submit data to be processed to a specified resource, often resulting in the creation of a new resource.
- Non-idempotent: POST requests are not idempotent, meaning multiple identical requests may have different effects.
- Usage: Often used for creating new resources when the server assigns the URI of the resource.
In short, PUT is used for updating or replacing existing resources, while POST is used for creating new resources or submitting data for processing.
10. What makes REST services to be easily scalable?
REST services follow the concept of statelessness which essentially means no storing of any data across the requests on the server. This makes it easier to scale horizontally because the servers need not communicate much with each other while serving requests.
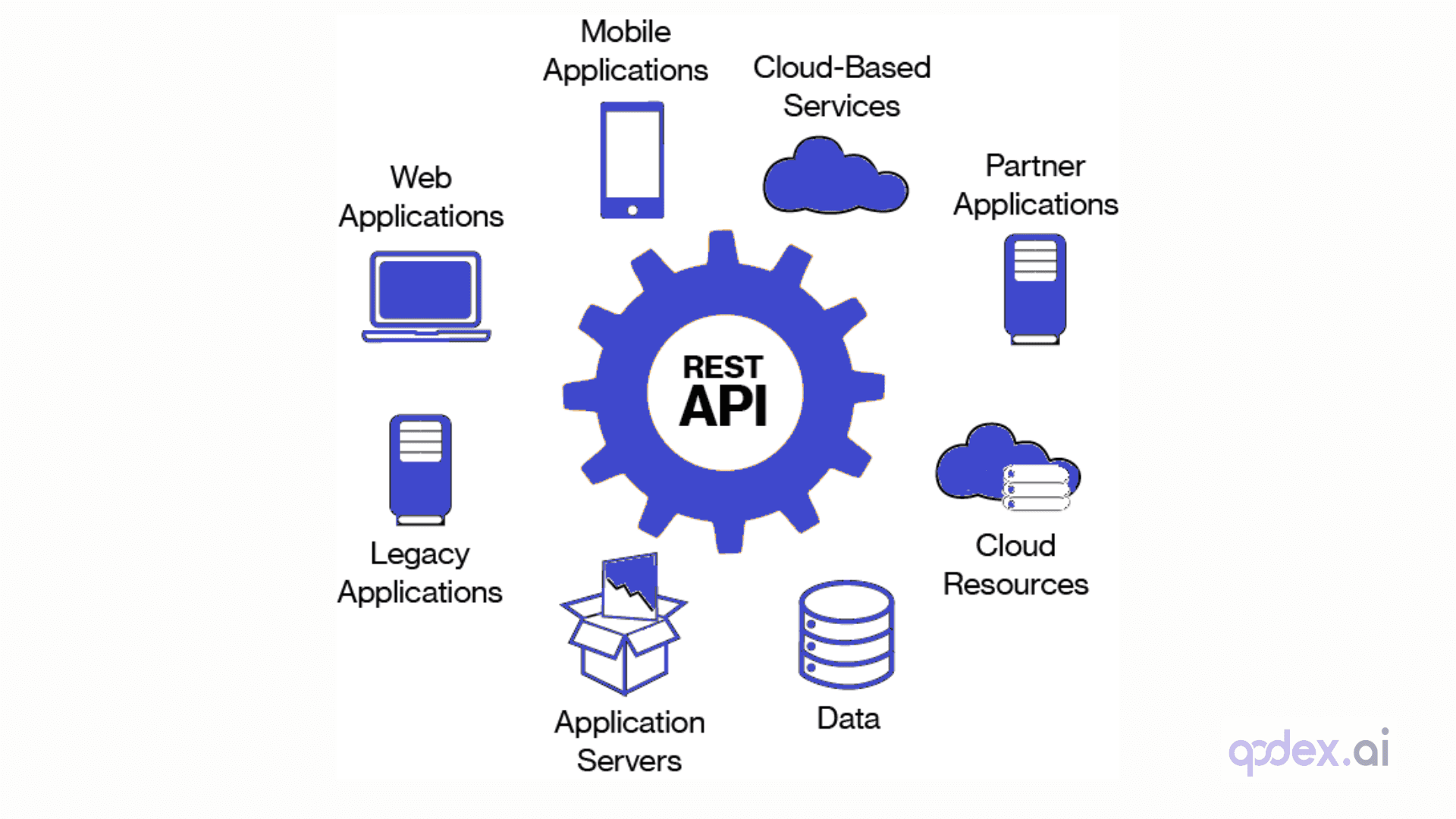
11. What is Payload in terms of RESTful web services?
Payload refers to the data passes in the request body. In RESTful web services, the term "payload" refers to the data that is sent as part of a request or response. It is the actual content of the message being sent.
Request Payload: In a request, the payload typically includes data that a client wants to send to the server, such as JSON or XML data. This payload is included in the body of the HTTP request.
Response Payload: In a response, the payload includes the data that the server sends back to the client in response to a request. This can also be JSON, XML, HTML, or any other format based on what the client requested.
In essence, the payload is the essential content that is transmitted between the client and server in a RESTful interaction.
12. Is it possible to send payload in the GET and DELETE methods?
While it's technically possible to include a payload in GET and DELETE requests according to the HTTP specification, it's generally discouraged due to issues with caching, security risks, and semantic clarity. It's considered best practice to use other HTTP methods like POST, PUT, or PATCH for requests requiring a payload.
13. What is the maximum payload size that can be sent in POST methods?
Theoretically, there is no restriction on the size of the payload that can be sent. But one must remember that the greater the size of the payload, the larger would be the bandwidth consumption and time taken to process the request that can impact the server performance.
14. How does HTTP Basic Authentication work?
While implementing Basic Authentication as part of APIs, the user must provide the username and password which is then concatenated by the browser in the form of “username: password” and then perform base64 encoding on it. The encoded value is then sent as the value for the “Authorization” header on every HTTP request from the browser. Since the credentials are only encoded, it is advised to use this form when requests are sent over HTTPS as they are not secure and can be intercepted by anyone if secure protocols are not used.
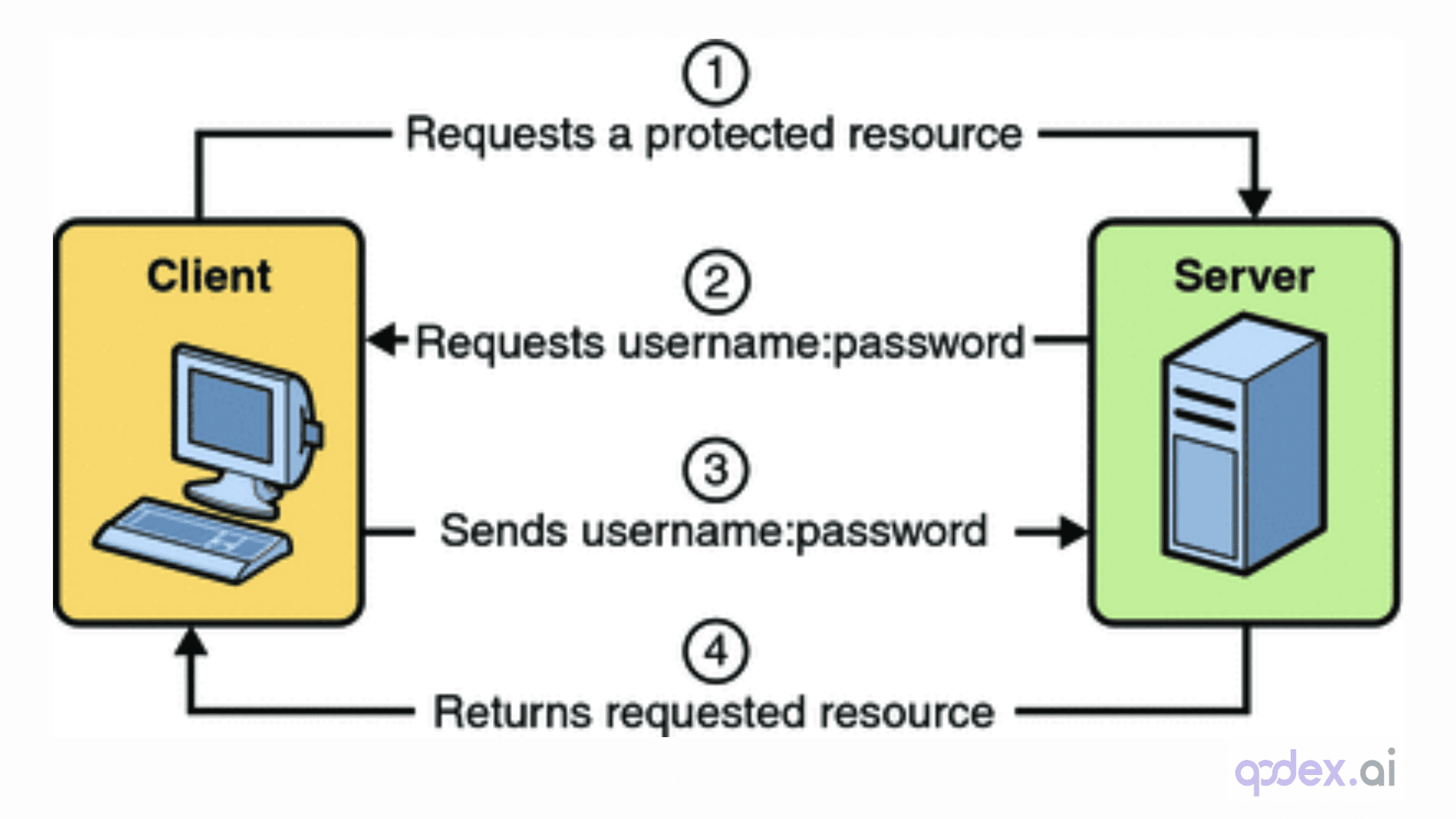
15. What is the difference between idempotent and safe HTTP methods?
Safe methods are those that do not change any resources internally. These methods can be cached and can be retrieved without any effects on the resource.
Idempotent methods are those methods that do not change the responses to the resources externally. They can be called multiple times without any change in the responses.
In the context of HTTP methods, the difference between idempotent and safe methods is as follows:
Safe Methods:
Definition: Safe methods are HTTP methods that do not modify resources on the server.
Characteristics: They are read-only operations that do not change the state of the server. Safe methods can be repeated without causing any additional side effects.
Examples include GET, HEAD, and OPTIONS.
Idempotent Methods:
Definition: Idempotent methods are HTTP methods that can be applied multiple times without changing the result beyond the first application.
Characteristics: They can be repeated multiple times with the same outcome.They do not cause unintended side effects even if the operation is repeated.
Examples include GET, PUT, DELETE, and certain uses of POST.
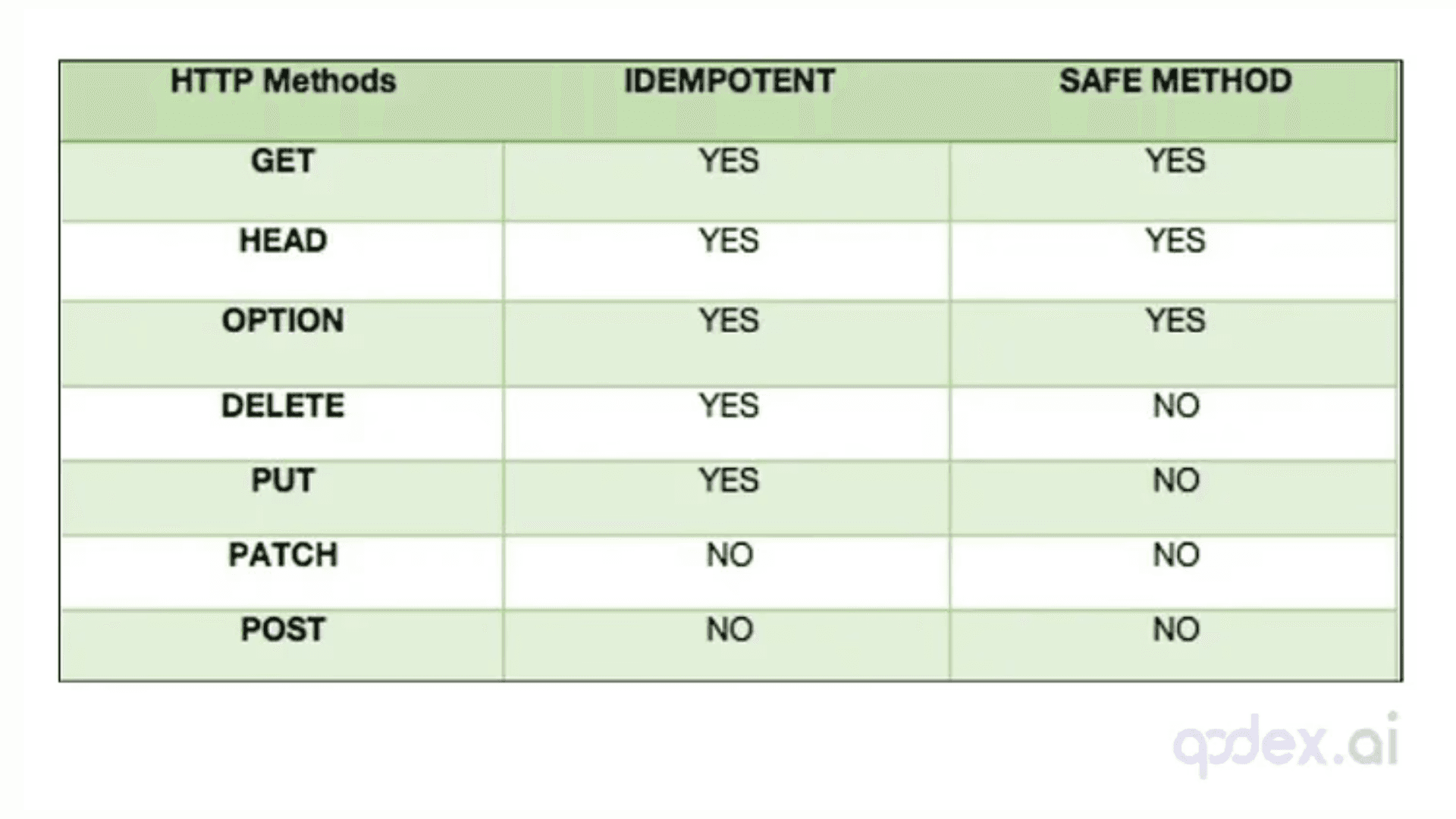
"Stay connected with us for the latest updates, insights, and exciting content! 🚀 Follow us on X and LinkedIn. Hit the 'Like' button, give us a 'Follow,' and don't forget to 'Share' to spread the knowledge and inspiration."
Differentiate between SOAP and REST?
SOAP (Simple Object Access Protocol):Protocol: SOAP is a protocol, providing a set of rules for structuring messages, defining endpoints, and describing operations.
Message Format: SOAP messages are typically XML-based, with a rigid structure defined by XML schemas.
Communication Style: SOAP relies on a contract-based communication style, where clients and servers agree on the format and structure of messages beforehand.
Stateful: SOAP supports stateful communication, allowing interactions that maintain session state between client and server.
Complexity: SOAP is considered more complex due to its strict message format and contract-based communication.
REST (Representational State Transfer):
Architectural Style: REST is an architectural style, emphasizing stateless communication and resource-based interactions.
Message Format: REST messages are typically represented in formats like JSON or XML, but the structure is flexible and not mandated by the protocol.
3. Communication Style: RESTful services follow a resource-based communication style, where clients interact with resources using standard HTTP methods.
4. Stateless: REST is stateless, meaning each request from a client to the server contains all the information needed to understand and fulfill the request.
5. Simplicity: REST is considered simpler than SOAP due to its lightweight communication style and flexible message format.
2. While creating URI for web services, what are the best practices that needs to be followed?
List of the best practices that need to be considered with designing URI for web services:
While defining resources, use plural nouns. (Example: To identify user resource, use the name “users” for that resource.)
While using the long name for resources, use underscore or hyphen. Avoid using spaces between words. (Example, to define authorized users resource, the name can be “authorized_users” or “authorized-users”.)
The URI is case-insensitive, but as part of best practice, it is recommended to use lower case only.
While developing URI, the backward compatibility must be maintained once it gets published.
When the URI is updated, the older URI must be redirected to the new one using the HTTP status code 300.
Use appropriate HTTP methods like GET, PUT, DELETE, PATCH, etc. It is not needed or recommended to use these method names in the URI. (Example: To get user details of a particular ID, use /users/{id} instead of /getUser)
Use the technique of forward slashing to indicate the hierarchy between the resources and the collections. (Example: To get the address of the user of a particular id, we can use: /users/{id}/address)
3. What are the best practices to develop RESTful web services?
Best practices for developing RESTful web services include:
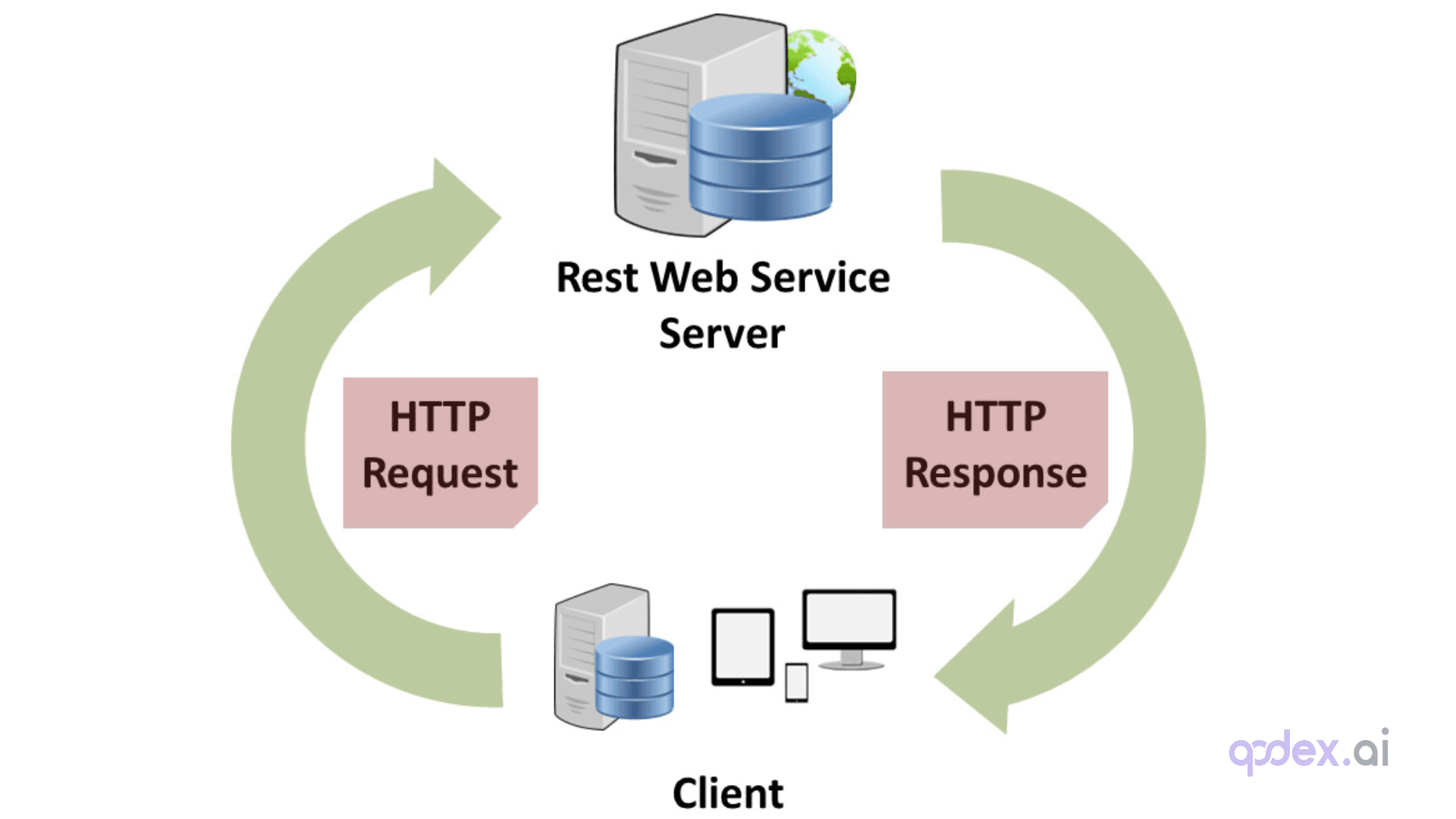
1. Use Descriptive URIs: URIs should be meaningful and descriptive of the resource they represent.
2. Follow HTTP Methods: Use appropriate HTTP methods (GET, POST, PUT, DELETE) to perform CRUD operations on resources.
3. Use HTTP Status Codes: Return relevant HTTP status codes to indicate the outcome of requests (e.g., 200 for success, 404 for not found).
4. Versioning: Implement versioning in URIs or headers to manage changes in APIs over time.
5. Input Validation: Validate input data to ensure security and prevent errors.
6. Error Handling: Provide informative error messages and handle errors gracefully.
7. Use Content Negotiation: Support multiple data formats (JSON, XML) through content negotiation.
8. Security: Implement authentication and authorization mechanisms to secure your APIs.
9. Caching: Use caching mechanisms to improve performance and reduce server load.
10. Documentation: Provide comprehensive documentation for your APIs, including usage examples and explanations of resources and endpoints.
4. What are Idempotent methods? How is it relevant in RESTful web services domain?
Idempotent methods are HTTP methods that can be safely repeated multiple times without causing different outcomes. In other words, performing the same idempotent operation multiple times produces the same result as performing it once.
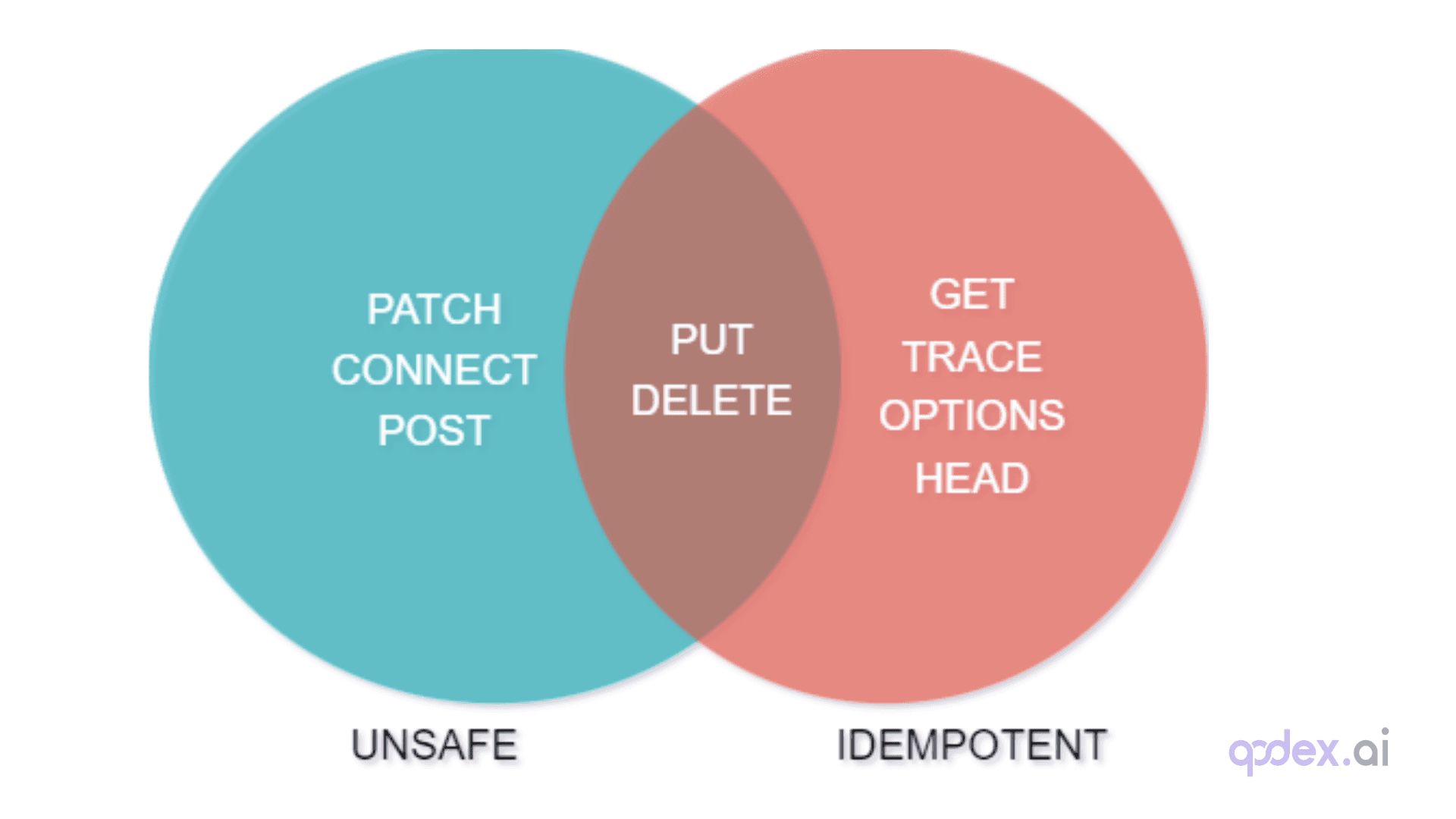
In the context of RESTful web services:
- Idempotent Methods: GET, PUT, and DELETE are considered idempotent methods in HTTP.
- Relevance in RESTful Web Services: Idempotent methods are crucial in RESTful web services because they ensure that repeating requests for resource retrieval (GET), creation or update (PUT), and deletion (DELETE) don't produce unintended side effects. This property simplifies error handling, enhances reliability, and improves scalability in distributed systems.
5. What are the differences between REST and AJAX?
REST vs AJAX:
1. Definition:
- REST (Representational State Transfer) is an architectural style for designing networked applications, emphasising stateless client-server communication via standardised HTTP methods.
- AJAX (Asynchronous JavaScript and XML) is a technique used in web development to create interactive and dynamic user interfaces, allowing asynchronous data retrieval from a server without refreshing the entire page.
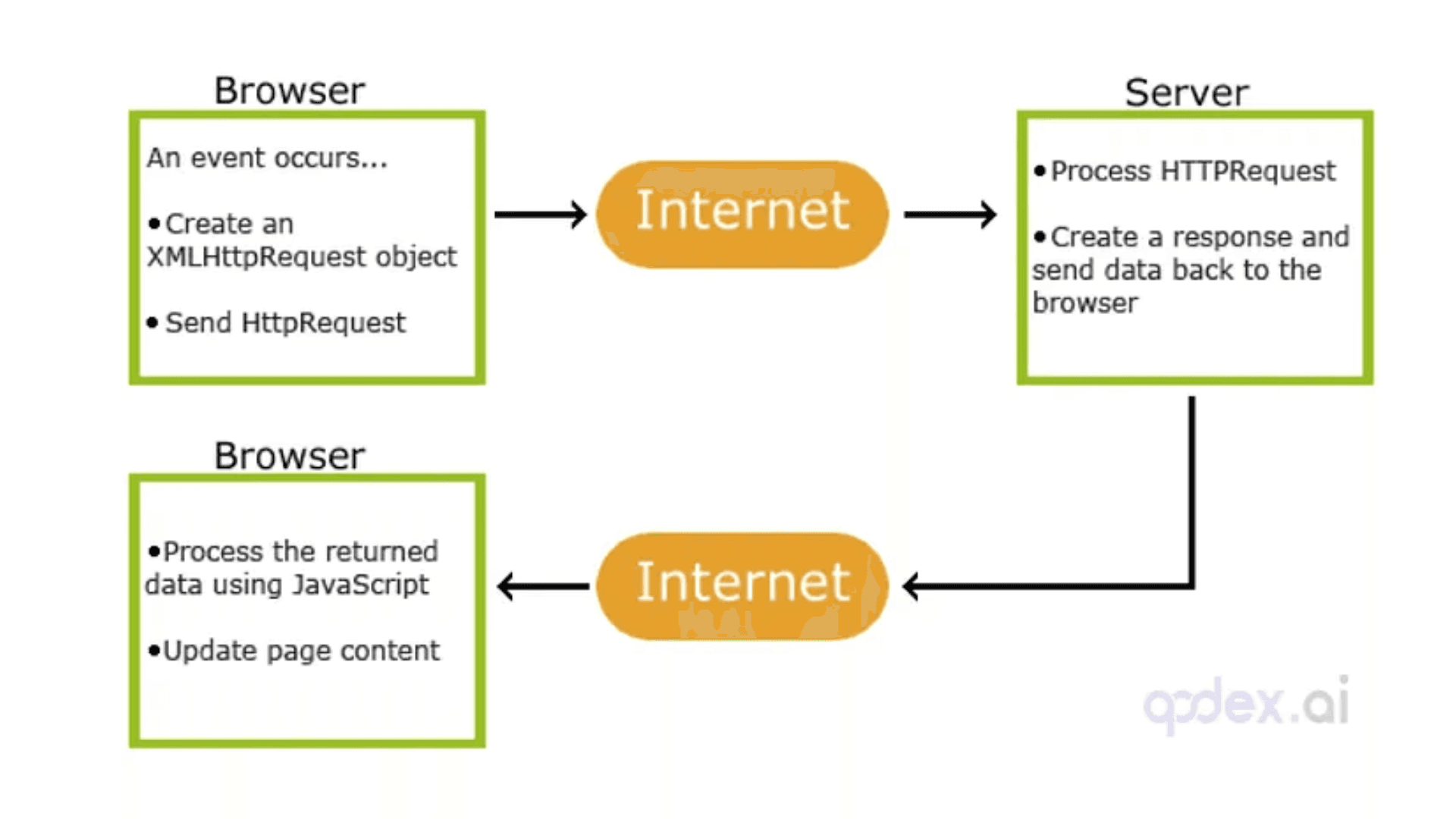
2. Purpose:
- REST is primarily used for designing web services and APIs that enable communication between client and server, typically for accessing and manipulating resources.
- AJAX is used for enhancing user experience by enabling seamless data retrieval and updating within a web page, without requiring a full page reload.
3. Communication Style:
- REST follows a stateless, resource-based communication style, where clients interact with resources via standardised HTTP methods (GET, POST, PUT, DELETE).
- AJAX allows asynchronous communication between client and server, typically using JavaScript to make HTTP requests in the background and update parts of a web page dynamically.
4. Scope:
- REST is more focused on defining the architecture and communication protocols for web services and APIs.
- AJAX is focused on the client-side implementation of web applications, particularly for handling dynamic content and user interactions.
5. Usage:
- REST is commonly used in web development for building APIs and web services that enable interoperability and data exchange between different systems.
- AJAX is widely used in web development for creating responsive and interactive user interfaces, such as updating content without page reloads, form validation, and real-time data fetching.
6. Can you tell what constitutes the core components of HTTP Request?
In REST, any HTTP Request has 5 main components, they are:
Method/Verb − This part tells what methods the request operation represents. Methods like GET, PUT, POST, DELETE, etc are some examples.
URI − This part is used for uniquely identifying the resources on the server.HTTP Version − This part indicates what version of HTTP protocol you are using. An example can be HTTP v1.1.
Request Header − This part has the details of the request metadata such as client type, the content format supported, message format, cache settings, etc.
Request Body − This part represents the actual message content to be sent to the server.
7. What constitutes the core components of HTTP Response?
HTTP Response has 4 components:
Response Status Code − This represents the server response status code for the requested resource. Example- 400 represents a client-side error, 200 represents a successful response.
HTTP Version − Indicates the HTTP protocol version.
Response Header − This part has the metadata of the response message. Data can describe what is the content length, content type, response date, what is server type, etc.
Response Body − This part contains what is the actual resource/message returned from the server.
8. Define Addressing in terms of RESTful Web Services.
Addressing is the process of locating a single/multiple resources that are present on the server. This task is accomplished by making use of URI (Uniform Resource Identifier). The general format of URI is <protocol>://<application-name>/<type-of-resource>/<id-of-resource>
9. What are the differences between PUT and POST in REST?
PUT:
- Purpose: Used to update or replace an existing resource or create a new resource if it doesn't exist.
- Idempotent: PUT requests are idempotent, meaning multiple identical requests have the same effect as a single request.
- Usage: Typically used when the client knows the exact URI of the resource it wants to update or create.
POST:
- Purpose: Used to submit data to be processed to a specified resource, often resulting in the creation of a new resource.
- Non-idempotent: POST requests are not idempotent, meaning multiple identical requests may have different effects.
- Usage: Often used for creating new resources when the server assigns the URI of the resource.
In short, PUT is used for updating or replacing existing resources, while POST is used for creating new resources or submitting data for processing.
10. What makes REST services to be easily scalable?
REST services follow the concept of statelessness which essentially means no storing of any data across the requests on the server. This makes it easier to scale horizontally because the servers need not communicate much with each other while serving requests.
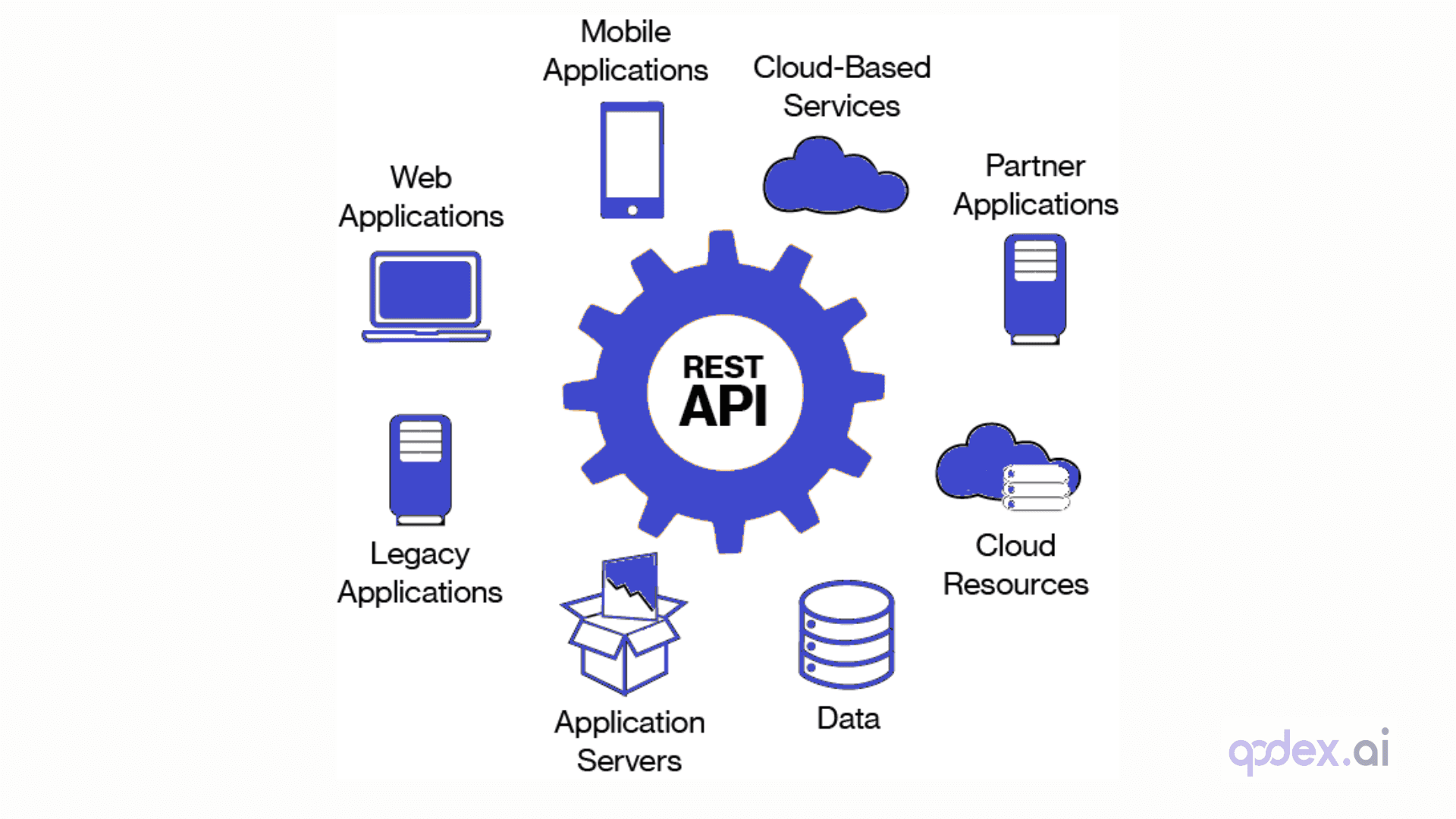
11. What is Payload in terms of RESTful web services?
Payload refers to the data passes in the request body. In RESTful web services, the term "payload" refers to the data that is sent as part of a request or response. It is the actual content of the message being sent.
Request Payload: In a request, the payload typically includes data that a client wants to send to the server, such as JSON or XML data. This payload is included in the body of the HTTP request.
Response Payload: In a response, the payload includes the data that the server sends back to the client in response to a request. This can also be JSON, XML, HTML, or any other format based on what the client requested.
In essence, the payload is the essential content that is transmitted between the client and server in a RESTful interaction.
12. Is it possible to send payload in the GET and DELETE methods?
While it's technically possible to include a payload in GET and DELETE requests according to the HTTP specification, it's generally discouraged due to issues with caching, security risks, and semantic clarity. It's considered best practice to use other HTTP methods like POST, PUT, or PATCH for requests requiring a payload.
13. What is the maximum payload size that can be sent in POST methods?
Theoretically, there is no restriction on the size of the payload that can be sent. But one must remember that the greater the size of the payload, the larger would be the bandwidth consumption and time taken to process the request that can impact the server performance.
14. How does HTTP Basic Authentication work?
While implementing Basic Authentication as part of APIs, the user must provide the username and password which is then concatenated by the browser in the form of “username: password” and then perform base64 encoding on it. The encoded value is then sent as the value for the “Authorization” header on every HTTP request from the browser. Since the credentials are only encoded, it is advised to use this form when requests are sent over HTTPS as they are not secure and can be intercepted by anyone if secure protocols are not used.
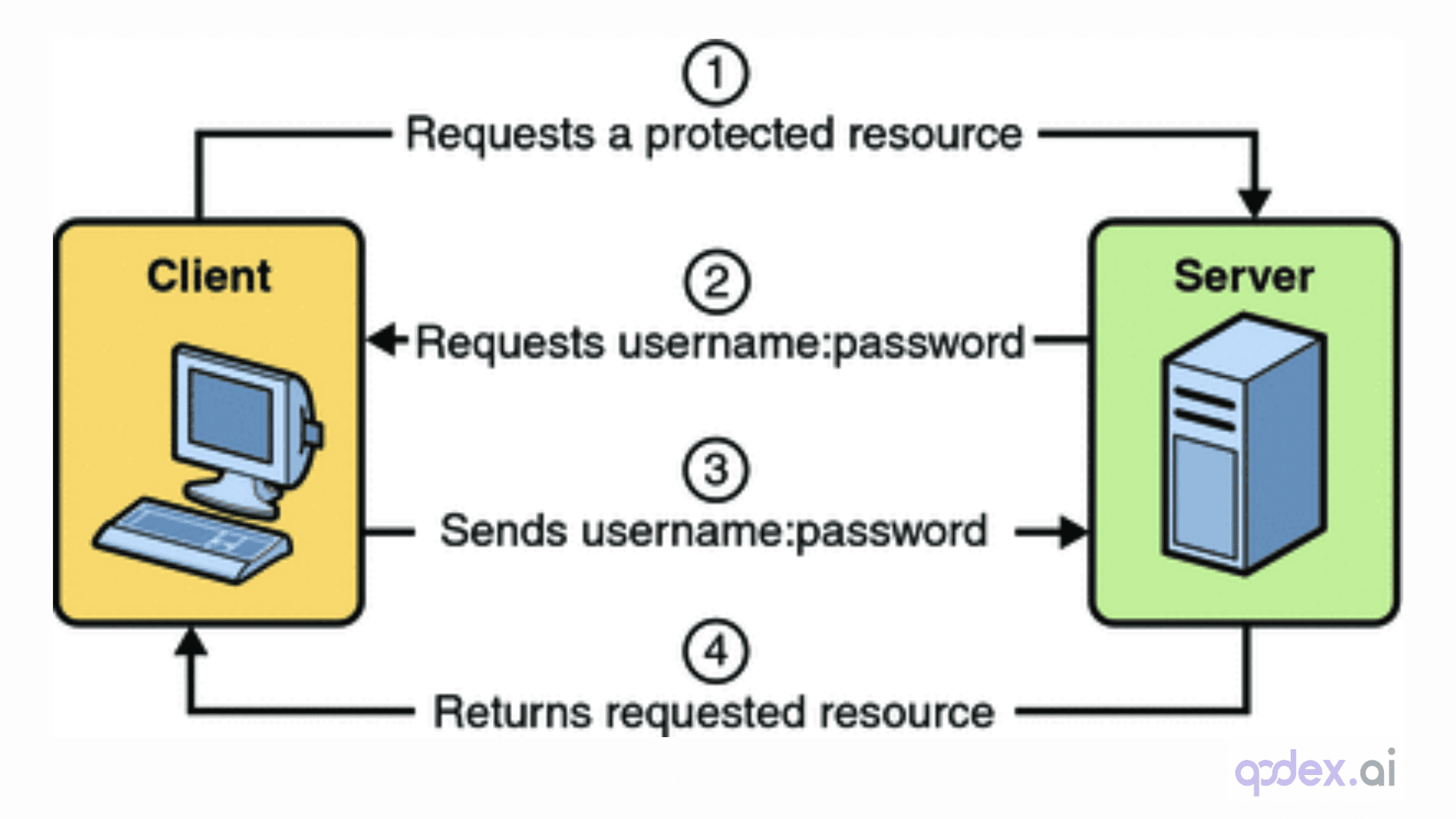
15. What is the difference between idempotent and safe HTTP methods?
Safe methods are those that do not change any resources internally. These methods can be cached and can be retrieved without any effects on the resource.
Idempotent methods are those methods that do not change the responses to the resources externally. They can be called multiple times without any change in the responses.
In the context of HTTP methods, the difference between idempotent and safe methods is as follows:
Safe Methods:
Definition: Safe methods are HTTP methods that do not modify resources on the server.
Characteristics: They are read-only operations that do not change the state of the server. Safe methods can be repeated without causing any additional side effects.
Examples include GET, HEAD, and OPTIONS.
Idempotent Methods:
Definition: Idempotent methods are HTTP methods that can be applied multiple times without changing the result beyond the first application.
Characteristics: They can be repeated multiple times with the same outcome.They do not cause unintended side effects even if the operation is repeated.
Examples include GET, PUT, DELETE, and certain uses of POST.
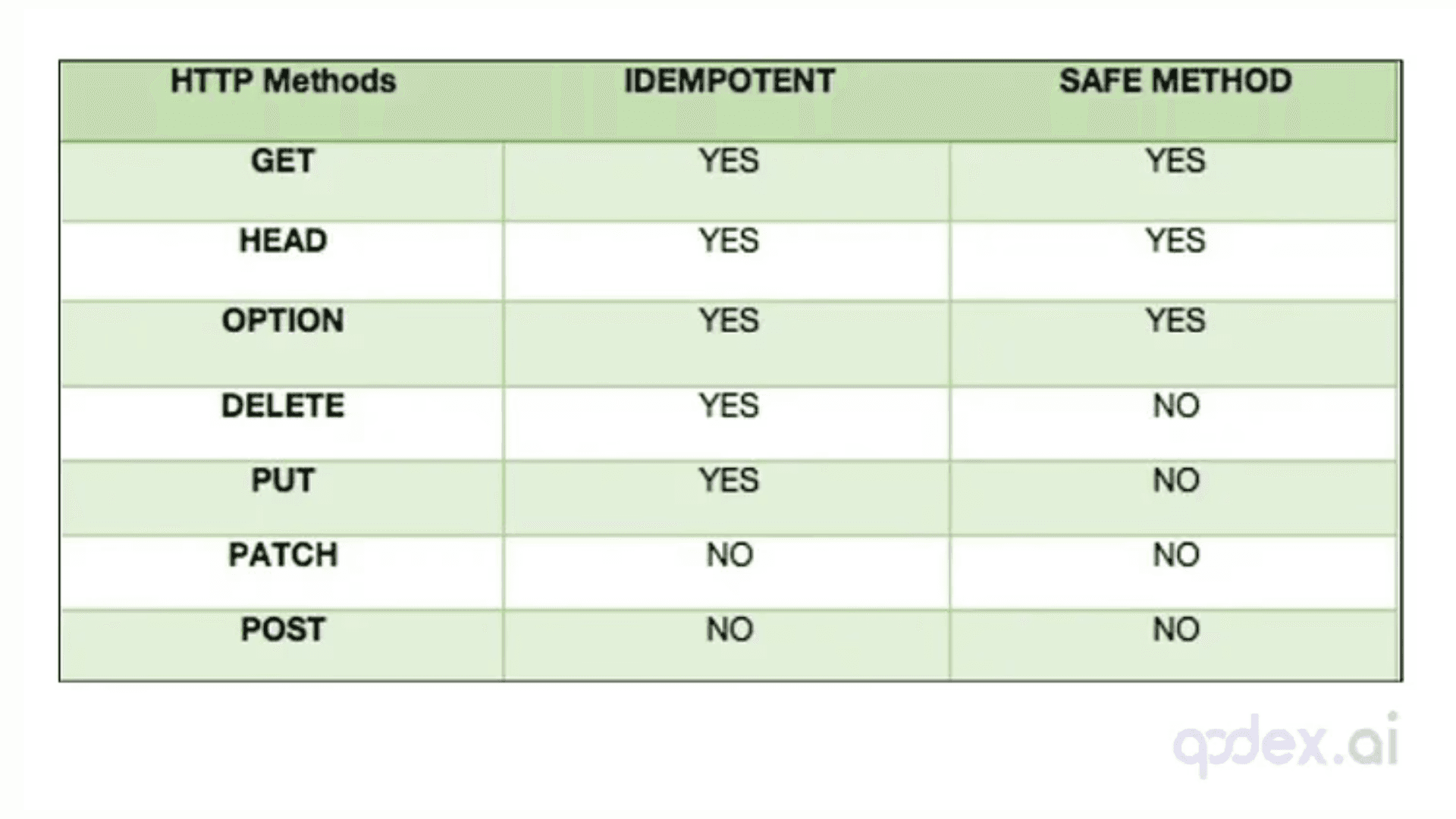
"Stay connected with us for the latest updates, insights, and exciting content! 🚀 Follow us on X and LinkedIn. Hit the 'Like' button, give us a 'Follow,' and don't forget to 'Share' to spread the knowledge and inspiration."

Ship bug-free software, 200% faster, in 20% testing budget. No coding required

Ship bug-free software, 200% faster, in 20% testing budget. No coding required

Ship bug-free software, 200% faster, in 20% testing budget. No coding required
FAQs
Why should you choose Qodex.ai?
Why should you choose Qodex.ai?
Why should you choose Qodex.ai?
15 Advanced REST API Interview Questions for Developers
Ship bug-free software,
200% faster, in 20% testing budget
Remommended posts

Hire our AI Software Test Engineer
Experience the future of automation software testing.
Copyright © 2024 Qodex
|
All Rights Reserved

Hire our AI Software Test Engineer
Experience the future of automation software testing.
Copyright © 2024 Qodex
All Rights Reserved

Hire our AI Software Test Engineer
Experience the future of automation software testing.
Copyright © 2024 Qodex
|
All Rights Reserved