REST Assured: A Complete Guide to API Test Automation
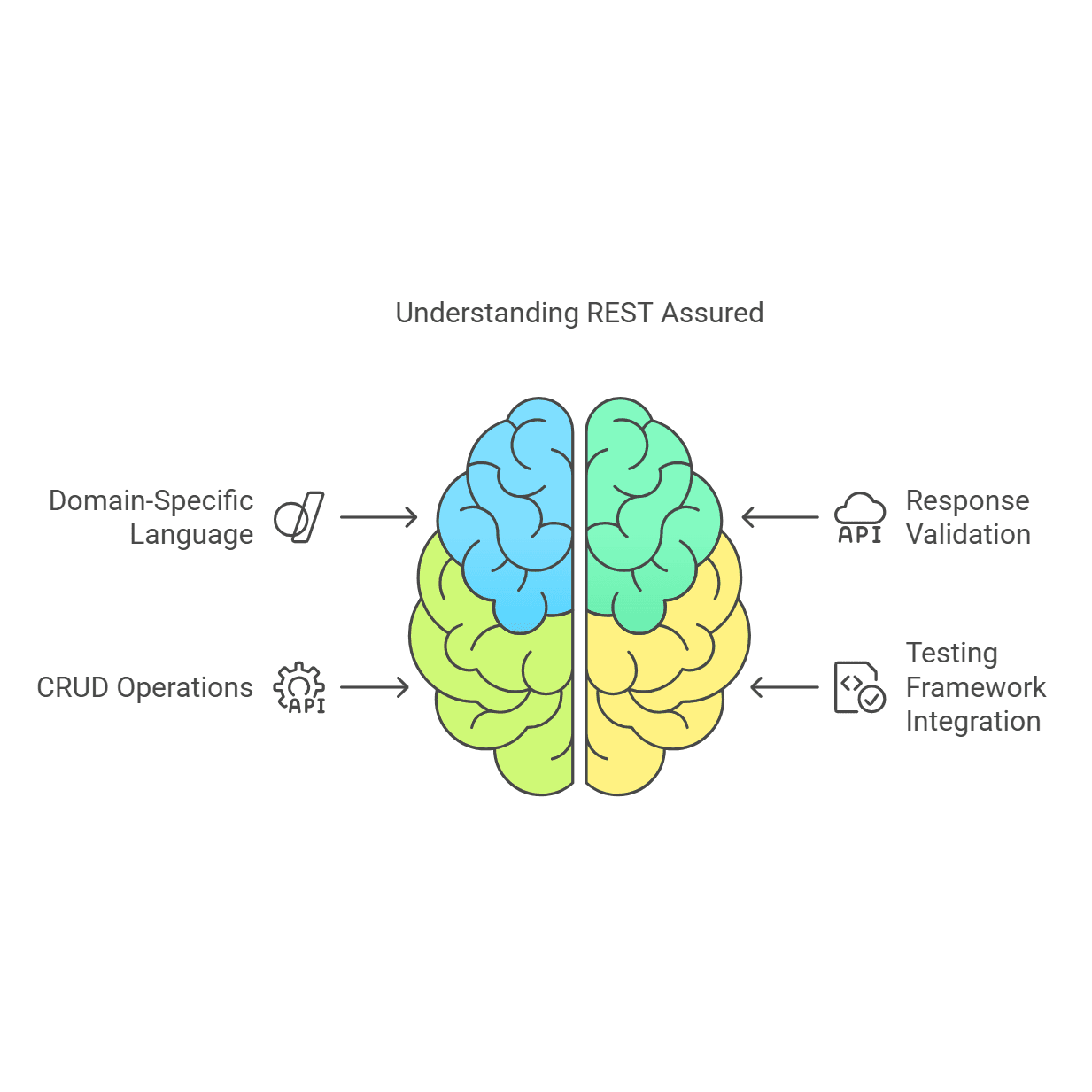
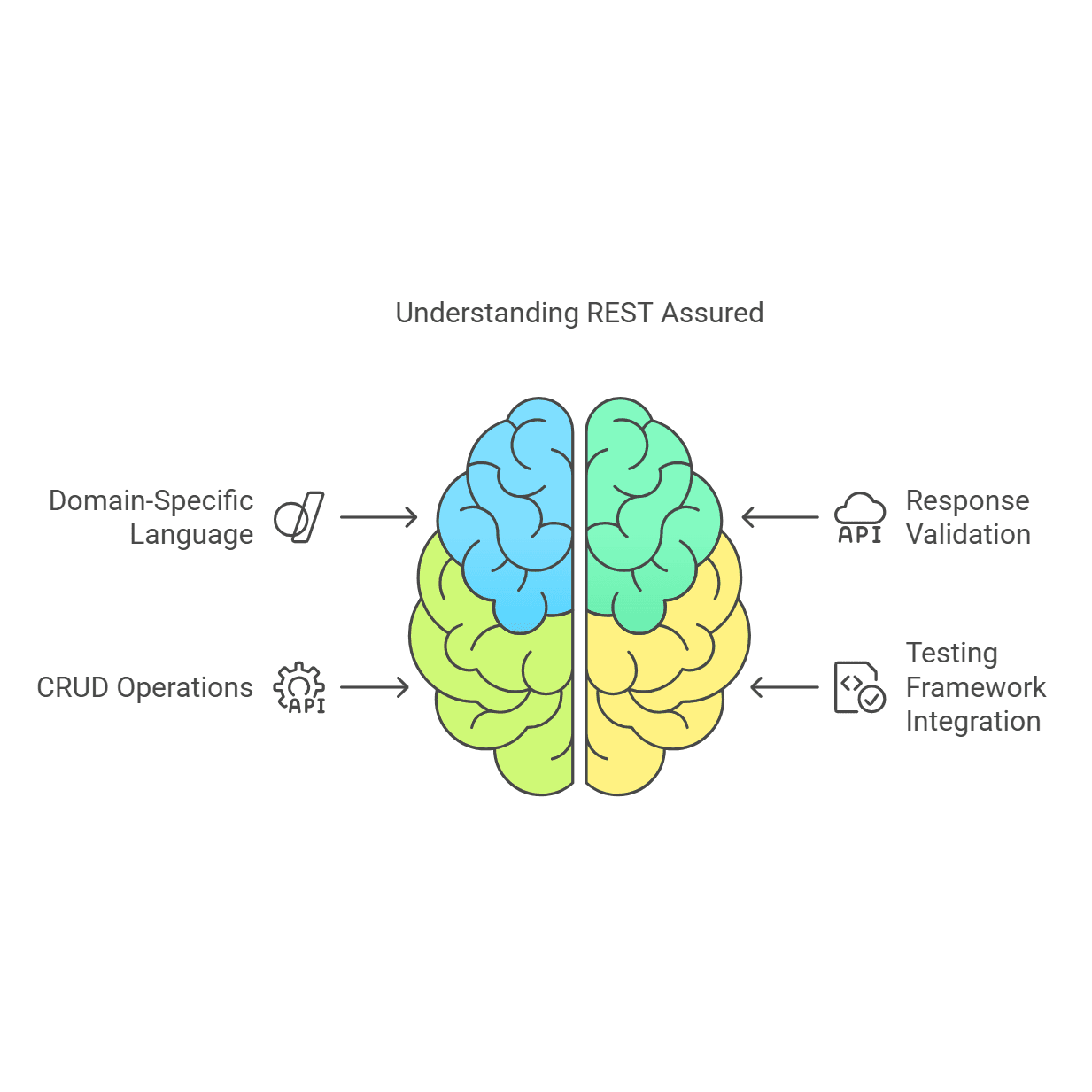
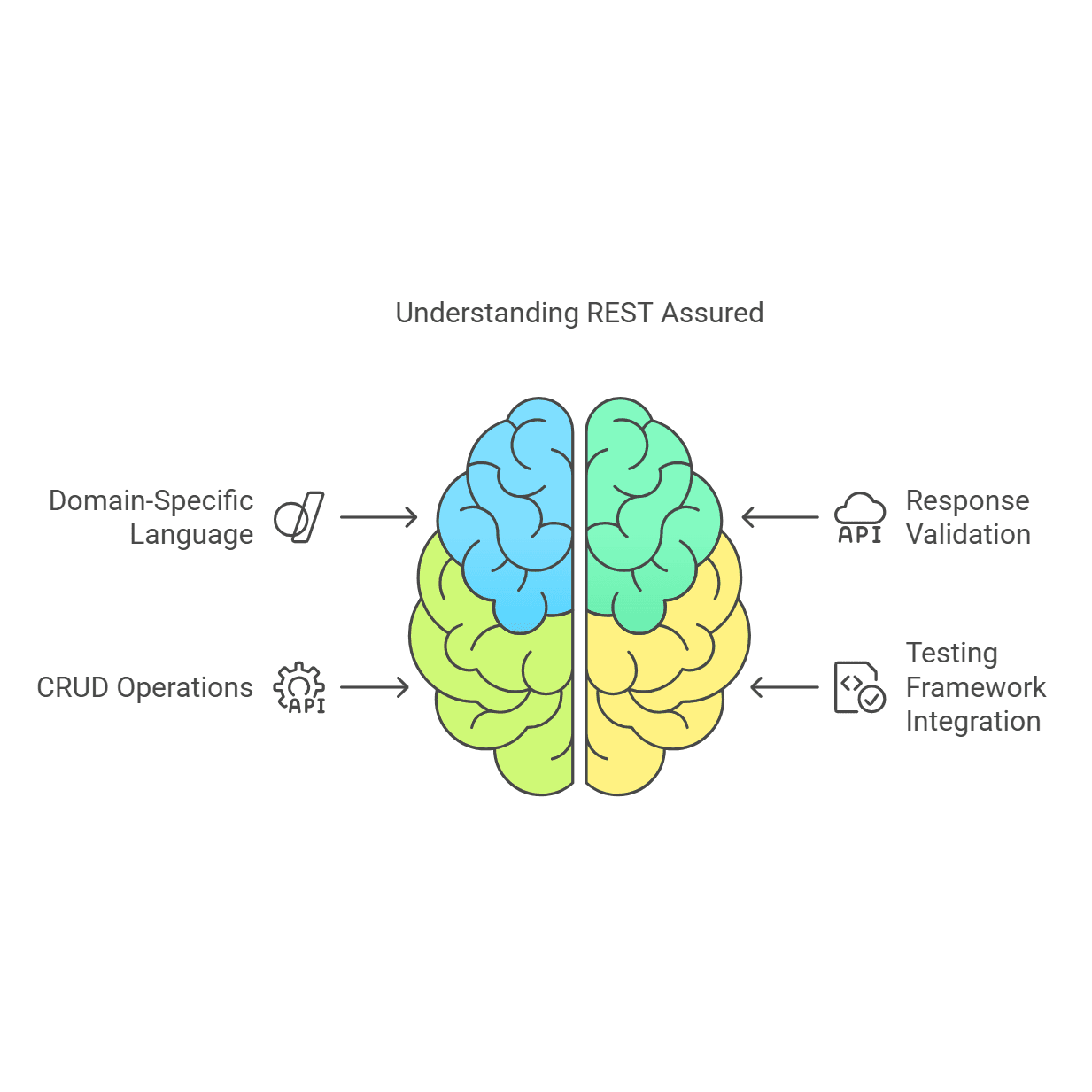
Ever wondered how companies ensure their APIs work flawlessly? Enter REST Assured, your go-to solution for API testing in Java. Think of REST Assured as your personal API detective – it methodically checks every request and response, making sure your APIs perform exactly as they should.
Let's break it down: REST Assured acts like an invisible middleman between your test code and your API. It's designed to speak the language of web services, handling all those complex HTTP requests and responses so you don't have to. Whether you're checking if your API correctly adds a new user or retrieves the right data, REST Assured has got your back.
Ever wondered how companies ensure their APIs work flawlessly? Enter REST Assured, your go-to solution for API testing in Java. Think of REST Assured as your personal API detective – it methodically checks every request and response, making sure your APIs perform exactly as they should.
Let's break it down: REST Assured acts like an invisible middleman between your test code and your API. It's designed to speak the language of web services, handling all those complex HTTP requests and responses so you don't have to. Whether you're checking if your API correctly adds a new user or retrieves the right data, REST Assured has got your back.
Ever wondered how companies ensure their APIs work flawlessly? Enter REST Assured, your go-to solution for API testing in Java. Think of REST Assured as your personal API detective – it methodically checks every request and response, making sure your APIs perform exactly as they should.
Let's break it down: REST Assured acts like an invisible middleman between your test code and your API. It's designed to speak the language of web services, handling all those complex HTTP requests and responses so you don't have to. Whether you're checking if your API correctly adds a new user or retrieves the right data, REST Assured has got your back.
Getting Started with REST Assured
Setting up REST Assured requires careful attention to your development environment. Let's walk through everything you need to get REST Assured running smoothly on your system.
First things first, you'll want to ensure your development environment is properly configured. REST Assured relies on specific tools and frameworks to function effectively:
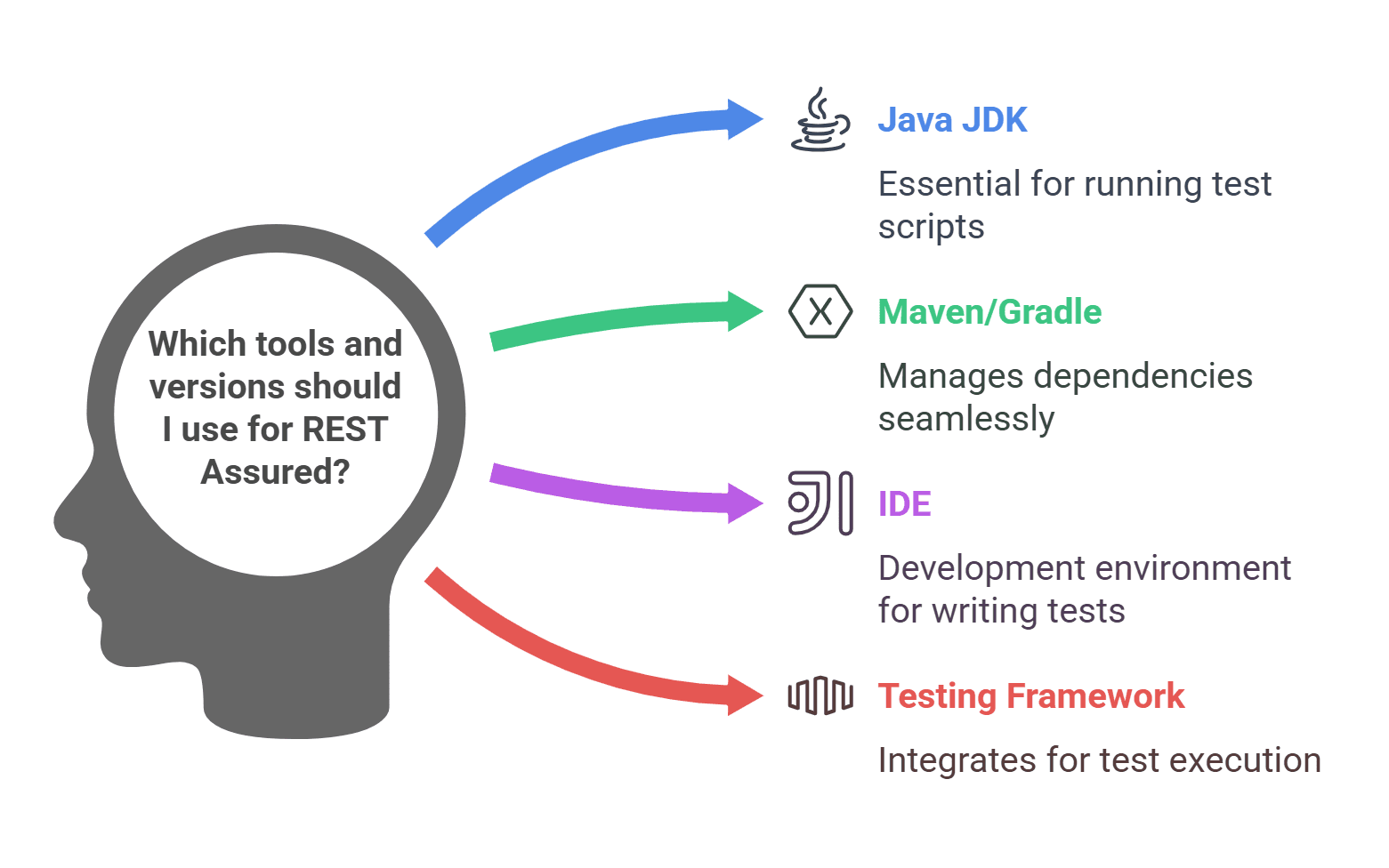
Once you have these prerequisites in place, you'll need to add REST Assured to your project. Here's the Maven dependency you'll want to include in your POM file:
xml
<dependency> <groupId>io.rest-assured</groupId> <artifactId>rest-assured</artifactId> <version>4.4.0</version> <scope>test</scope> </dependency>
After adding the dependency, REST Assured will be ready to use in your test classes. You can verify your setup by writing a simple test:
java
import io.restassured.RestAssured; import org.testng.annotations.Test; public class SimpleTest { @Test public void basicTest() { RestAssured.given() .when() .get("https://api.example.com") .then() .statusCode(200); } }
With this foundation in place, you're all set to start exploring REST Assured's powerful testing capabilities. Remember to keep your dependencies updated to access the latest features and security patches that REST Assured offers.
Setting up REST Assured requires careful attention to your development environment. Let's walk through everything you need to get REST Assured running smoothly on your system.
First things first, you'll want to ensure your development environment is properly configured. REST Assured relies on specific tools and frameworks to function effectively:
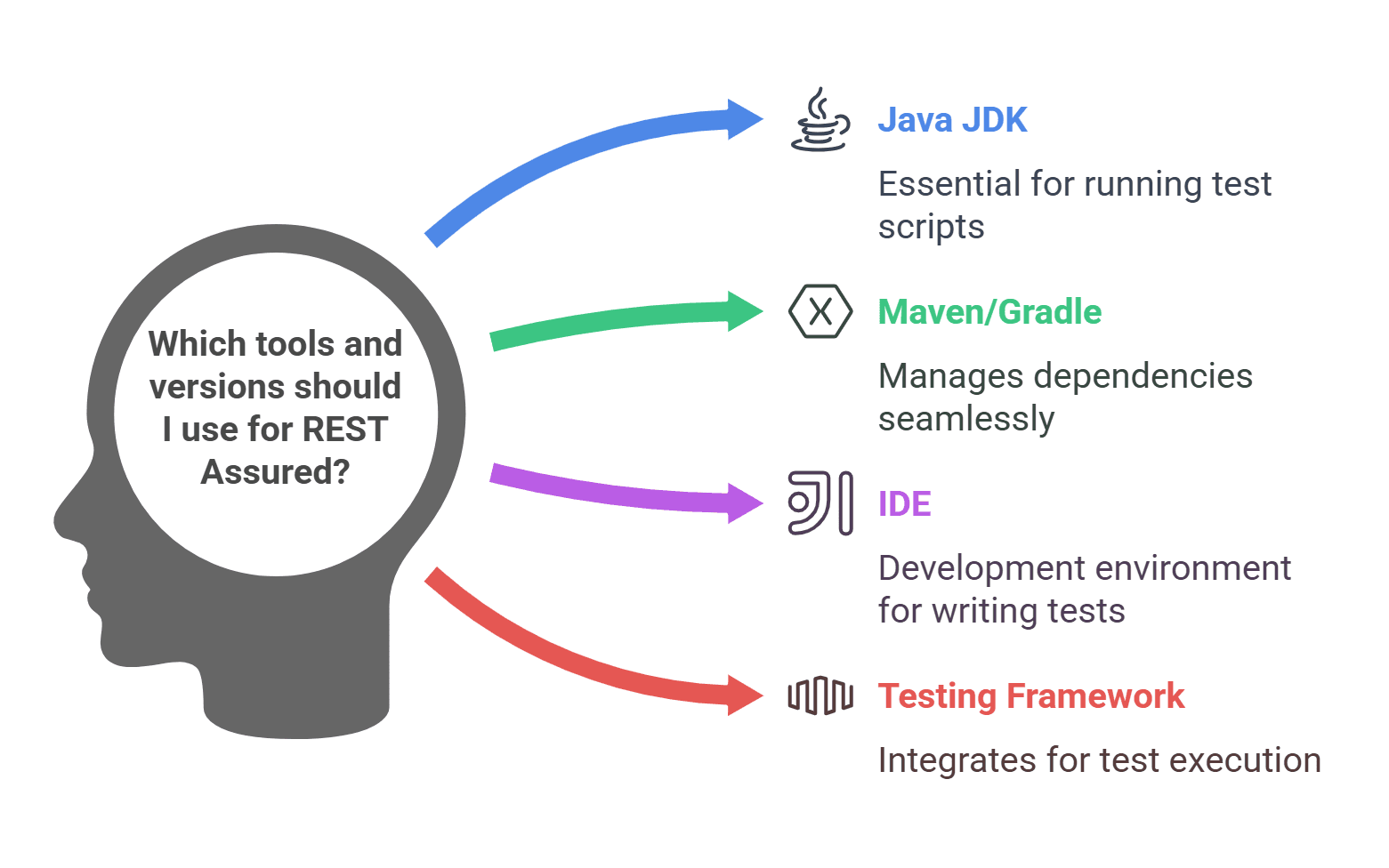
Once you have these prerequisites in place, you'll need to add REST Assured to your project. Here's the Maven dependency you'll want to include in your POM file:
xml
<dependency> <groupId>io.rest-assured</groupId> <artifactId>rest-assured</artifactId> <version>4.4.0</version> <scope>test</scope> </dependency>
After adding the dependency, REST Assured will be ready to use in your test classes. You can verify your setup by writing a simple test:
java
import io.restassured.RestAssured; import org.testng.annotations.Test; public class SimpleTest { @Test public void basicTest() { RestAssured.given() .when() .get("https://api.example.com") .then() .statusCode(200); } }
With this foundation in place, you're all set to start exploring REST Assured's powerful testing capabilities. Remember to keep your dependencies updated to access the latest features and security patches that REST Assured offers.
Setting up REST Assured requires careful attention to your development environment. Let's walk through everything you need to get REST Assured running smoothly on your system.
First things first, you'll want to ensure your development environment is properly configured. REST Assured relies on specific tools and frameworks to function effectively:
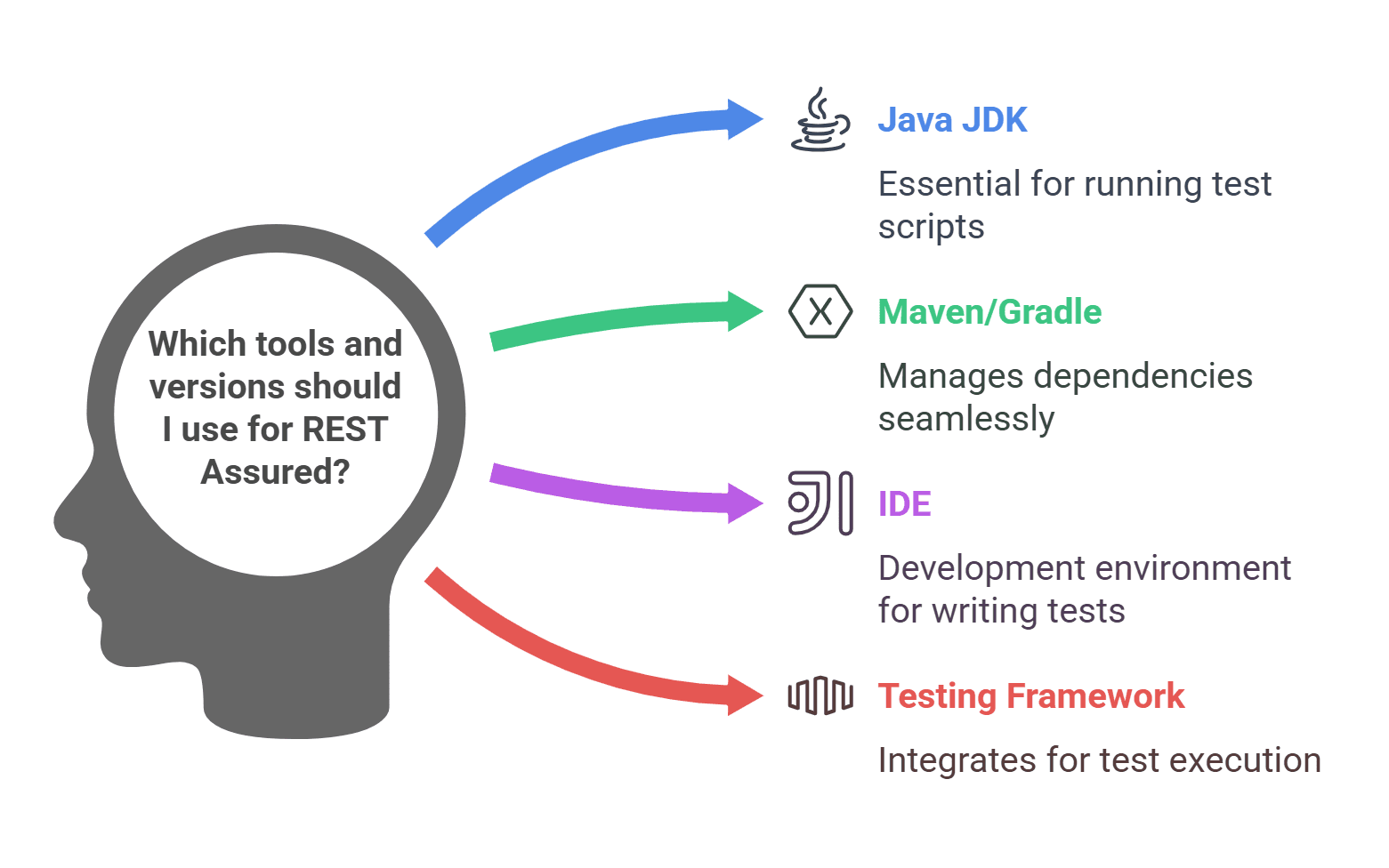
Once you have these prerequisites in place, you'll need to add REST Assured to your project. Here's the Maven dependency you'll want to include in your POM file:
xml
<dependency> <groupId>io.rest-assured</groupId> <artifactId>rest-assured</artifactId> <version>4.4.0</version> <scope>test</scope> </dependency>
After adding the dependency, REST Assured will be ready to use in your test classes. You can verify your setup by writing a simple test:
java
import io.restassured.RestAssured; import org.testng.annotations.Test; public class SimpleTest { @Test public void basicTest() { RestAssured.given() .when() .get("https://api.example.com") .then() .statusCode(200); } }
With this foundation in place, you're all set to start exploring REST Assured's powerful testing capabilities. Remember to keep your dependencies updated to access the latest features and security patches that REST Assured offers.

Ship bug-free software, 200% faster, in 20% testing budget. No coding required

Ship bug-free software, 200% faster, in 20% testing budget. No coding required

Ship bug-free software, 200% faster, in 20% testing budget. No coding required
Core Components of REST Assured
Understanding the core components of REST Assured helps you build robust API tests. Let's dive into how REST Assured handles different HTTP methods and validation techniques.
HTTP Methods in REST Assured
REST Assured supports all standard HTTP methods, making it versatile for different testing scenarios:
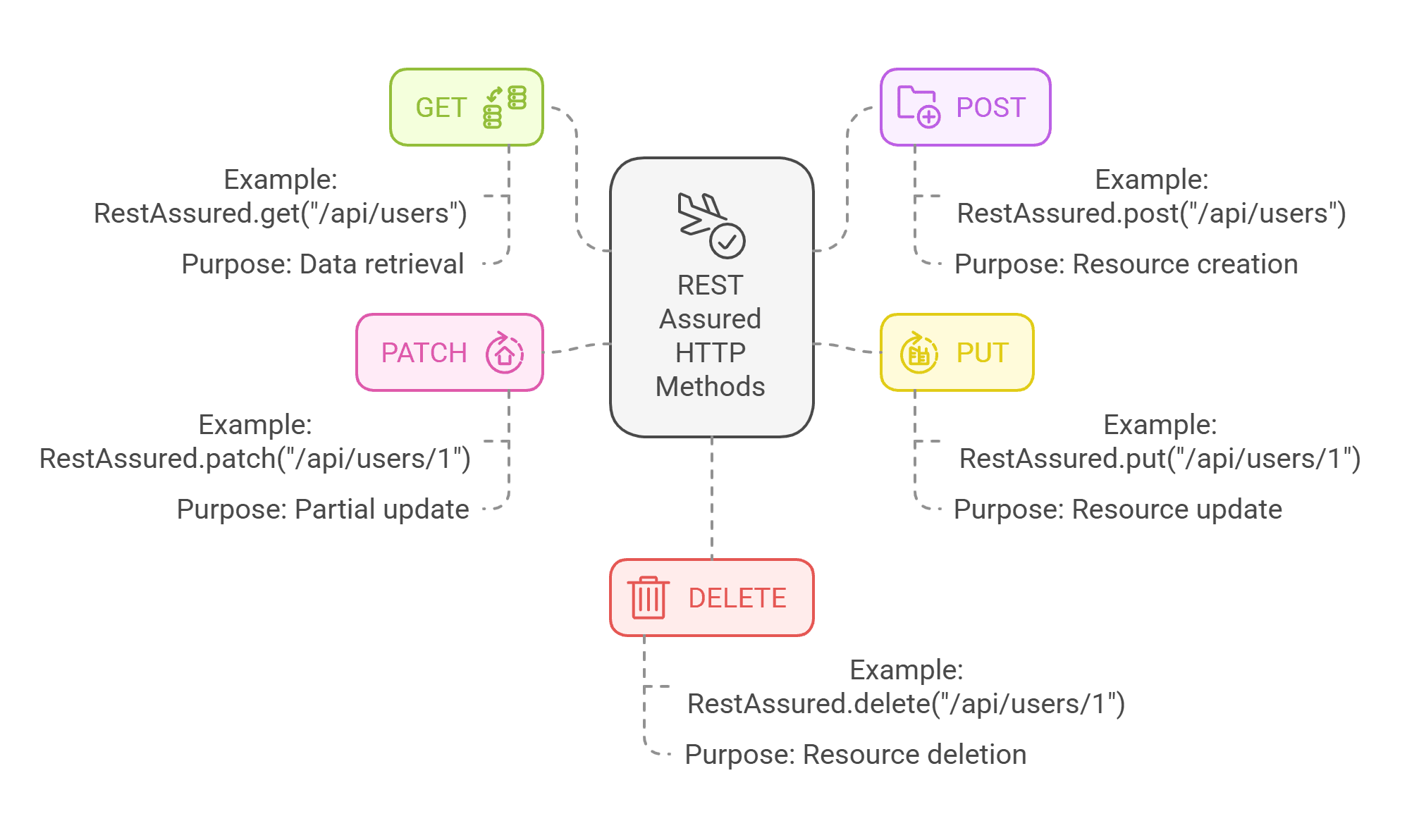
Response Validation
REST Assured shines in its response validation capabilities. Here's a typical validation flow:
java
RestAssured.given() .when() .get("/api/users") .then() .statusCode(200) .time(lessThan(2000L)) // Response time in milliseconds .header("Content-Type", "application/json") .body("name", equalTo("John"));
Status Code and Header Management
Response validation in REST Assured involves checking multiple components:
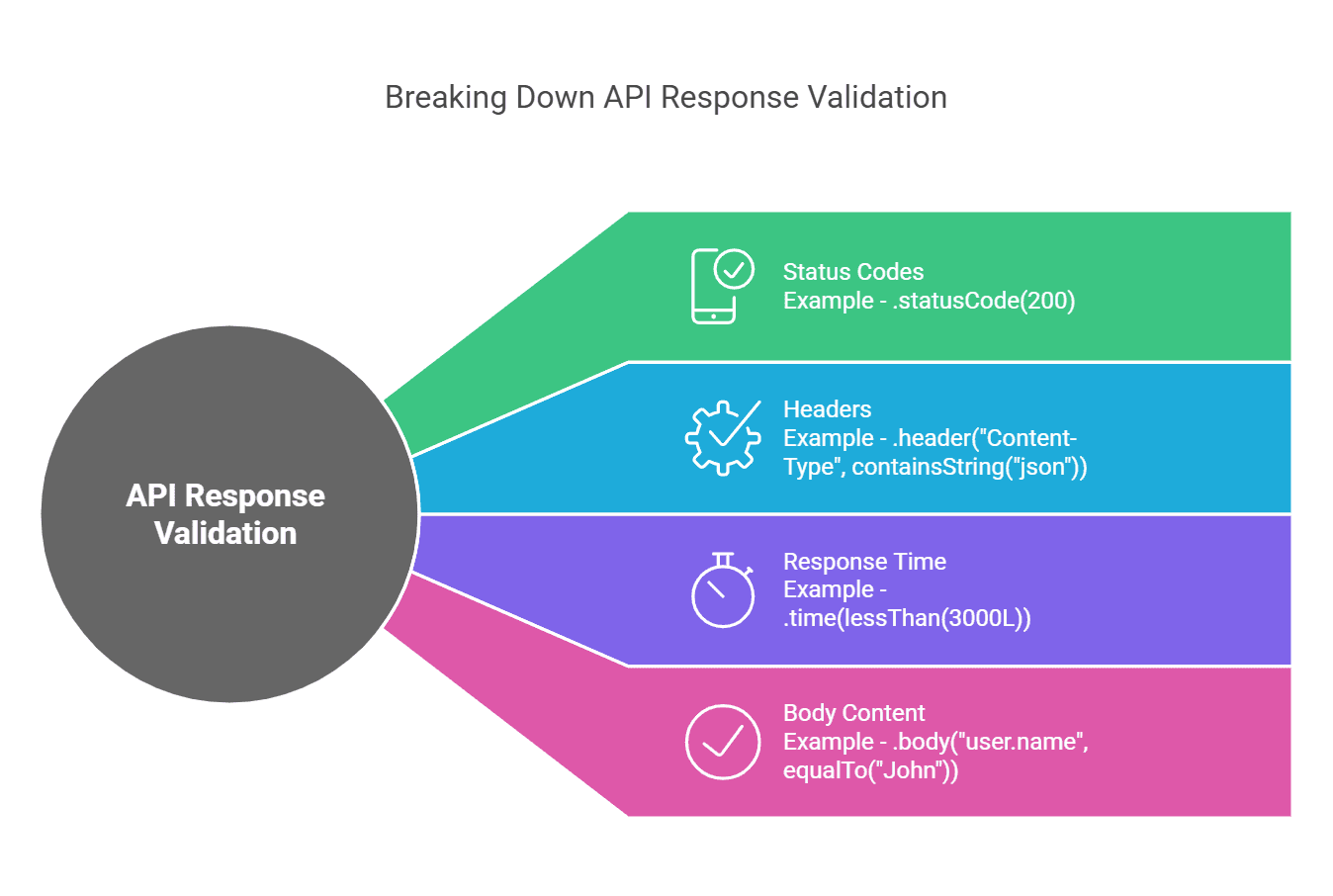
Response validation in REST Assured involves checking multiple components:These components work together in REST Assured to ensure your APIs function correctly and meet performance requirements. Remember to include appropriate assertions for each component based on your testing needs.
By mastering these core components, you'll be able to create comprehensive API tests that verify both functionality and performance using REST Assured's intuitive syntax.
BDD Syntax Structure in REST Assured
The beauty of REST Assured lies in its intuitive Behavior-Driven Development (BDD) syntax. Let's explore how this structured approach makes API testing more readable and maintainable.
Understanding the Flow
REST Assured uses a natural language chain that mirrors how we think about testing:
java
RestAssured.given() .header("Authorization", "Bearer token123") .contentType("application/json") .when() .post("/api/users") .then() .statusCode(201) .body("message", equalTo("User created"));
The Three Pillars of REST Assured
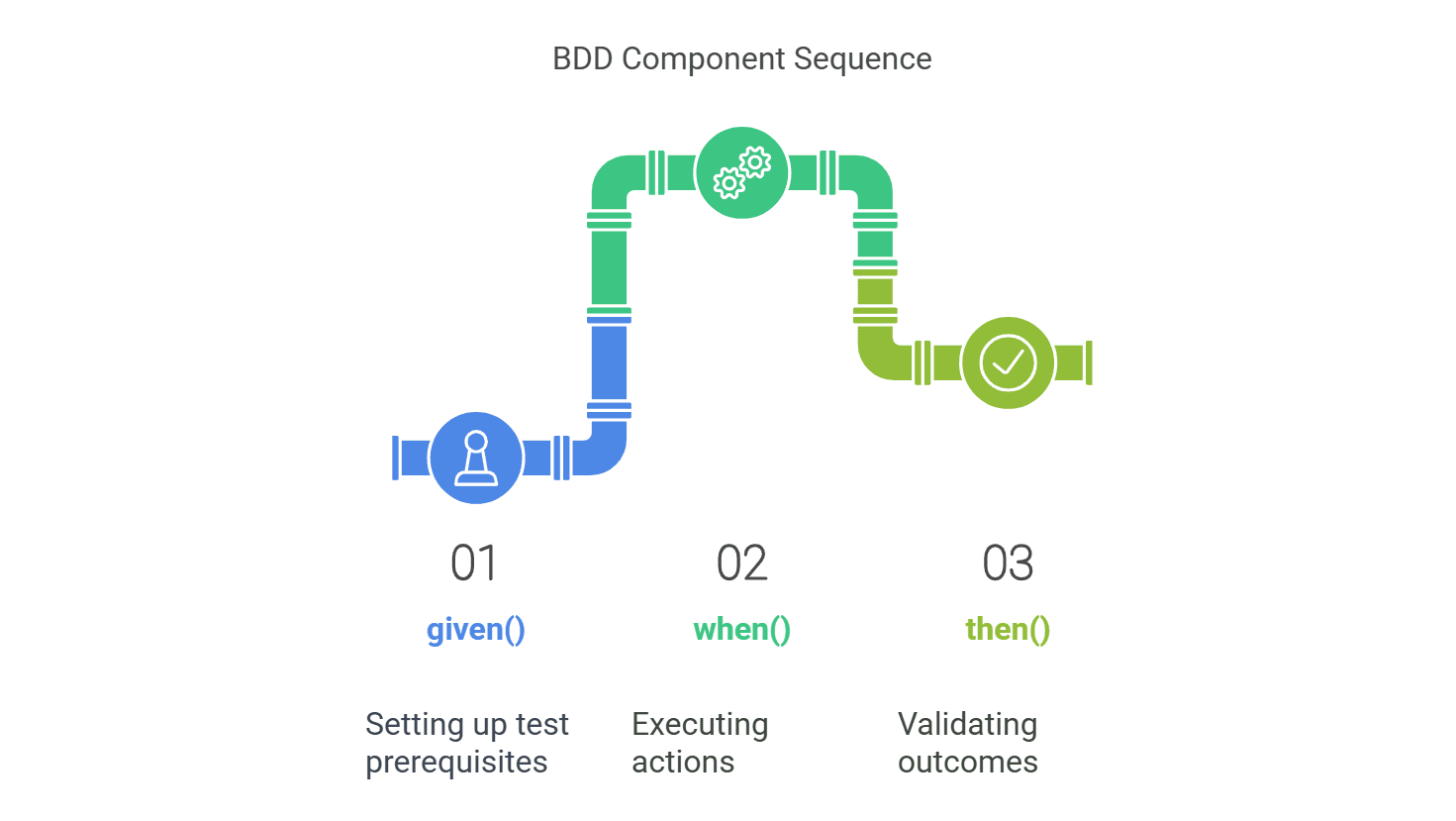
Practical Implementation
Here's how each component works in REST Assured:
given() - The Setup Phase
java
RestAssured.given() .baseUri("https://api.example.com") .header("Content-Type", "application/json") .body({"name": "John", "role": "developer"});
when() - The Action Phase
java
.when() .post("/users");
then() - The Verification Phase
java
.then() .statusCode(201) .body("success", equalTo(true));
This structured approach in REST Assured makes your tests not only easy to write but also simple to maintain and understand. Each section clearly indicates its purpose, making debugging and modifications straightforward.
Remember, while REST Assured follows this BDD pattern, you can be flexible with how you use it based on your testing needs. The key is maintaining readability while ensuring thorough API testing.
Understanding the core components of REST Assured helps you build robust API tests. Let's dive into how REST Assured handles different HTTP methods and validation techniques.
HTTP Methods in REST Assured
REST Assured supports all standard HTTP methods, making it versatile for different testing scenarios:
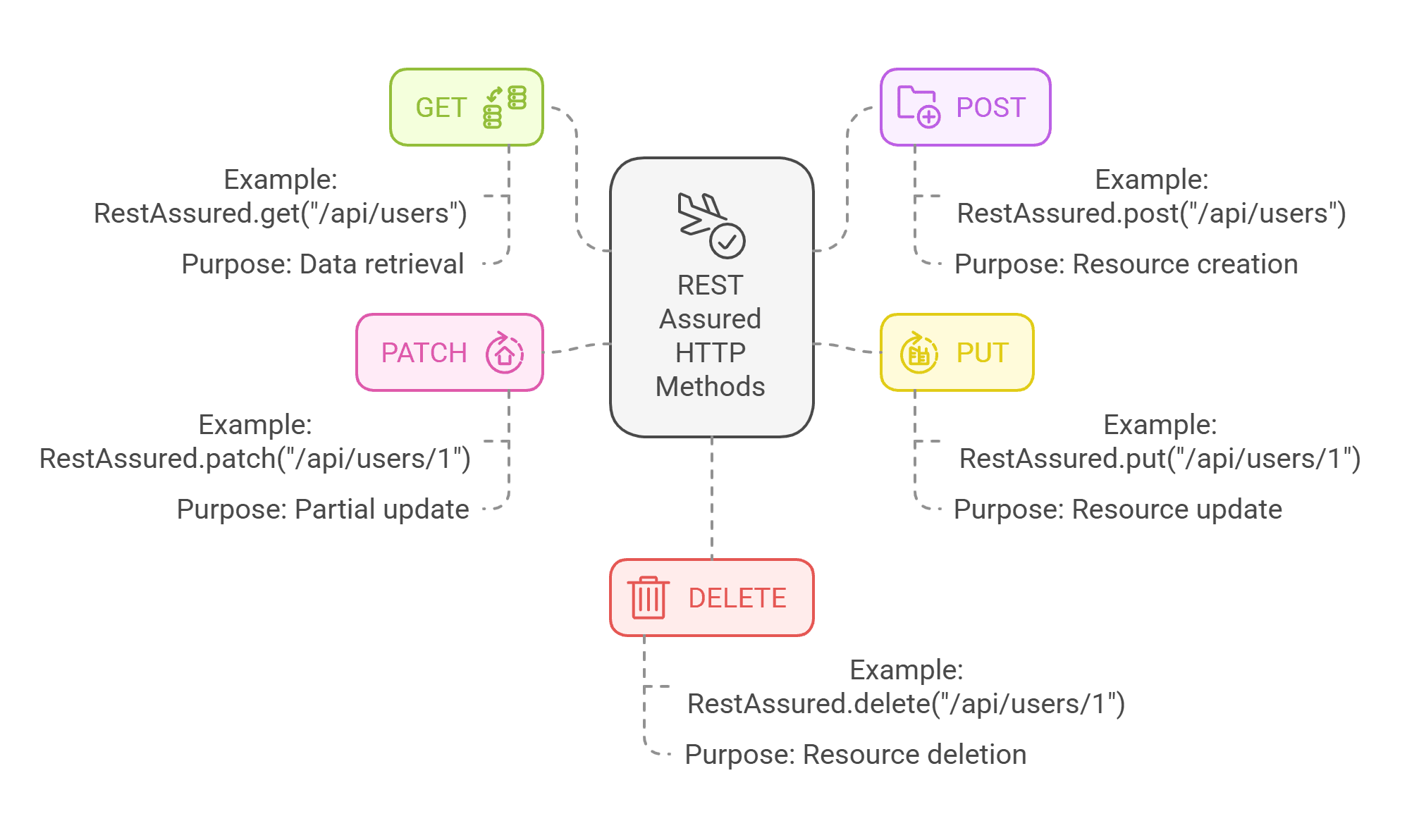
Response Validation
REST Assured shines in its response validation capabilities. Here's a typical validation flow:
java
RestAssured.given() .when() .get("/api/users") .then() .statusCode(200) .time(lessThan(2000L)) // Response time in milliseconds .header("Content-Type", "application/json") .body("name", equalTo("John"));
Status Code and Header Management
Response validation in REST Assured involves checking multiple components:
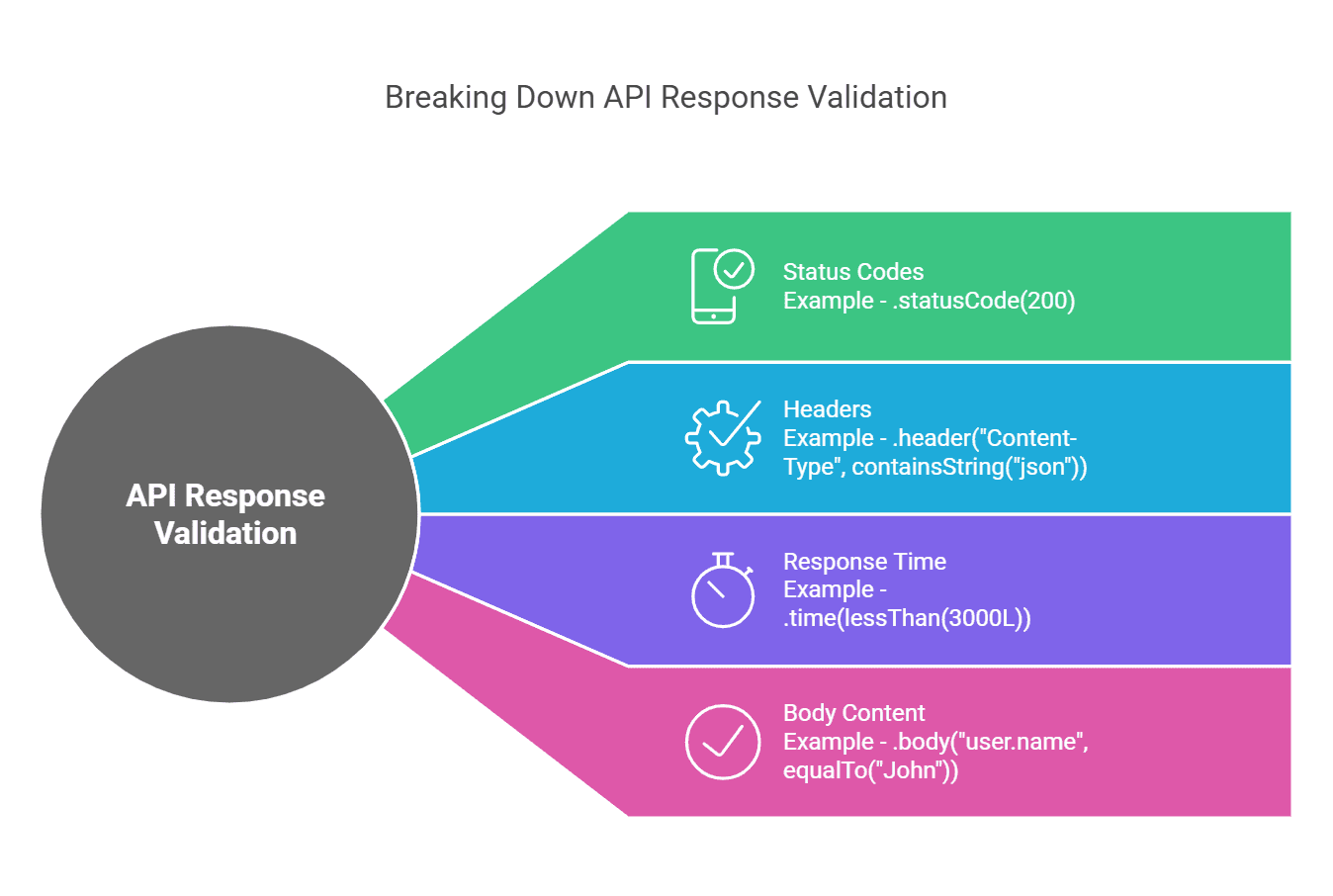
Response validation in REST Assured involves checking multiple components:These components work together in REST Assured to ensure your APIs function correctly and meet performance requirements. Remember to include appropriate assertions for each component based on your testing needs.
By mastering these core components, you'll be able to create comprehensive API tests that verify both functionality and performance using REST Assured's intuitive syntax.
BDD Syntax Structure in REST Assured
The beauty of REST Assured lies in its intuitive Behavior-Driven Development (BDD) syntax. Let's explore how this structured approach makes API testing more readable and maintainable.
Understanding the Flow
REST Assured uses a natural language chain that mirrors how we think about testing:
java
RestAssured.given() .header("Authorization", "Bearer token123") .contentType("application/json") .when() .post("/api/users") .then() .statusCode(201) .body("message", equalTo("User created"));
The Three Pillars of REST Assured
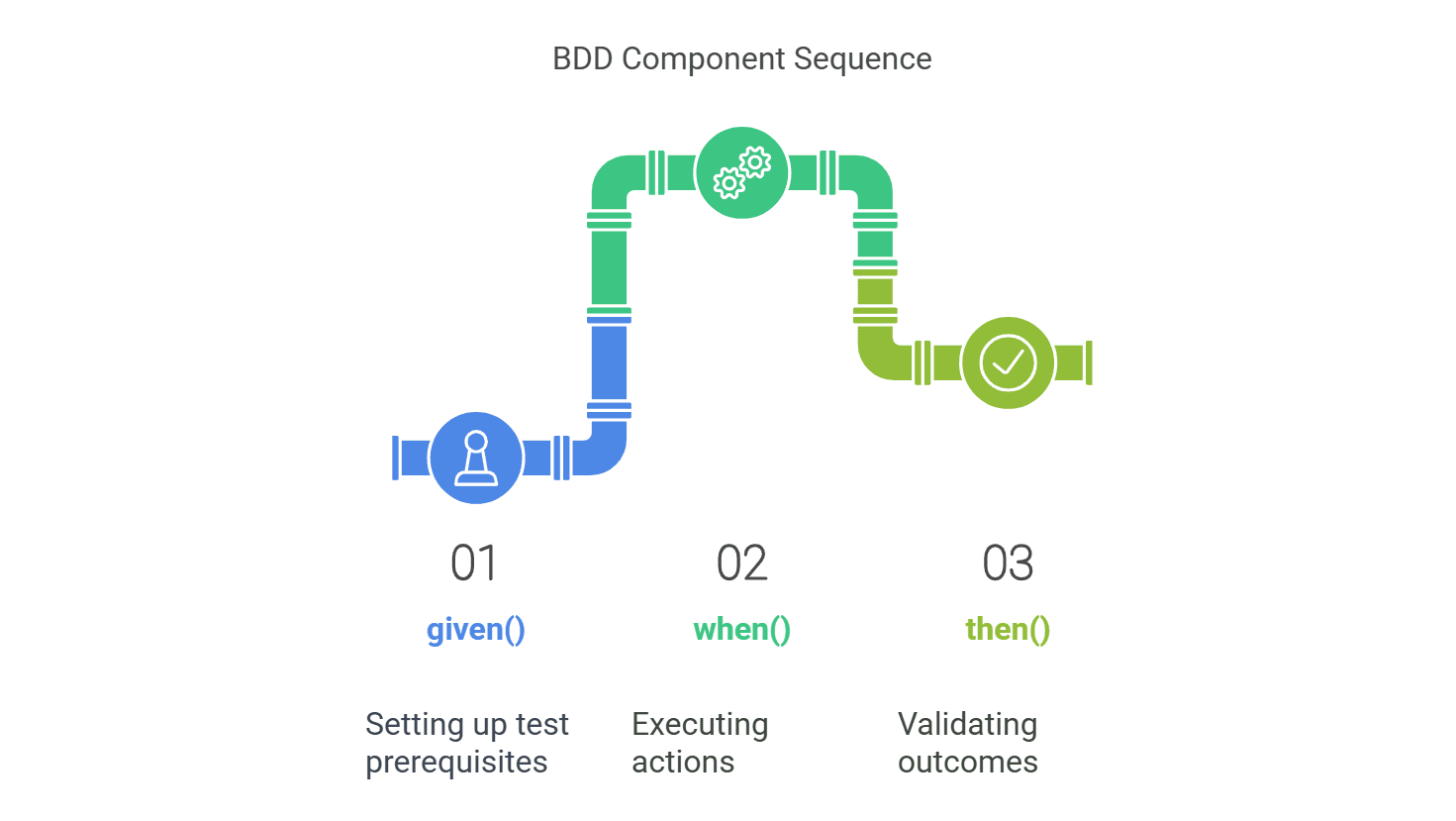
Practical Implementation
Here's how each component works in REST Assured:
given() - The Setup Phase
java
RestAssured.given() .baseUri("https://api.example.com") .header("Content-Type", "application/json") .body({"name": "John", "role": "developer"});
when() - The Action Phase
java
.when() .post("/users");
then() - The Verification Phase
java
.then() .statusCode(201) .body("success", equalTo(true));
This structured approach in REST Assured makes your tests not only easy to write but also simple to maintain and understand. Each section clearly indicates its purpose, making debugging and modifications straightforward.
Remember, while REST Assured follows this BDD pattern, you can be flexible with how you use it based on your testing needs. The key is maintaining readability while ensuring thorough API testing.
Understanding the core components of REST Assured helps you build robust API tests. Let's dive into how REST Assured handles different HTTP methods and validation techniques.
HTTP Methods in REST Assured
REST Assured supports all standard HTTP methods, making it versatile for different testing scenarios:
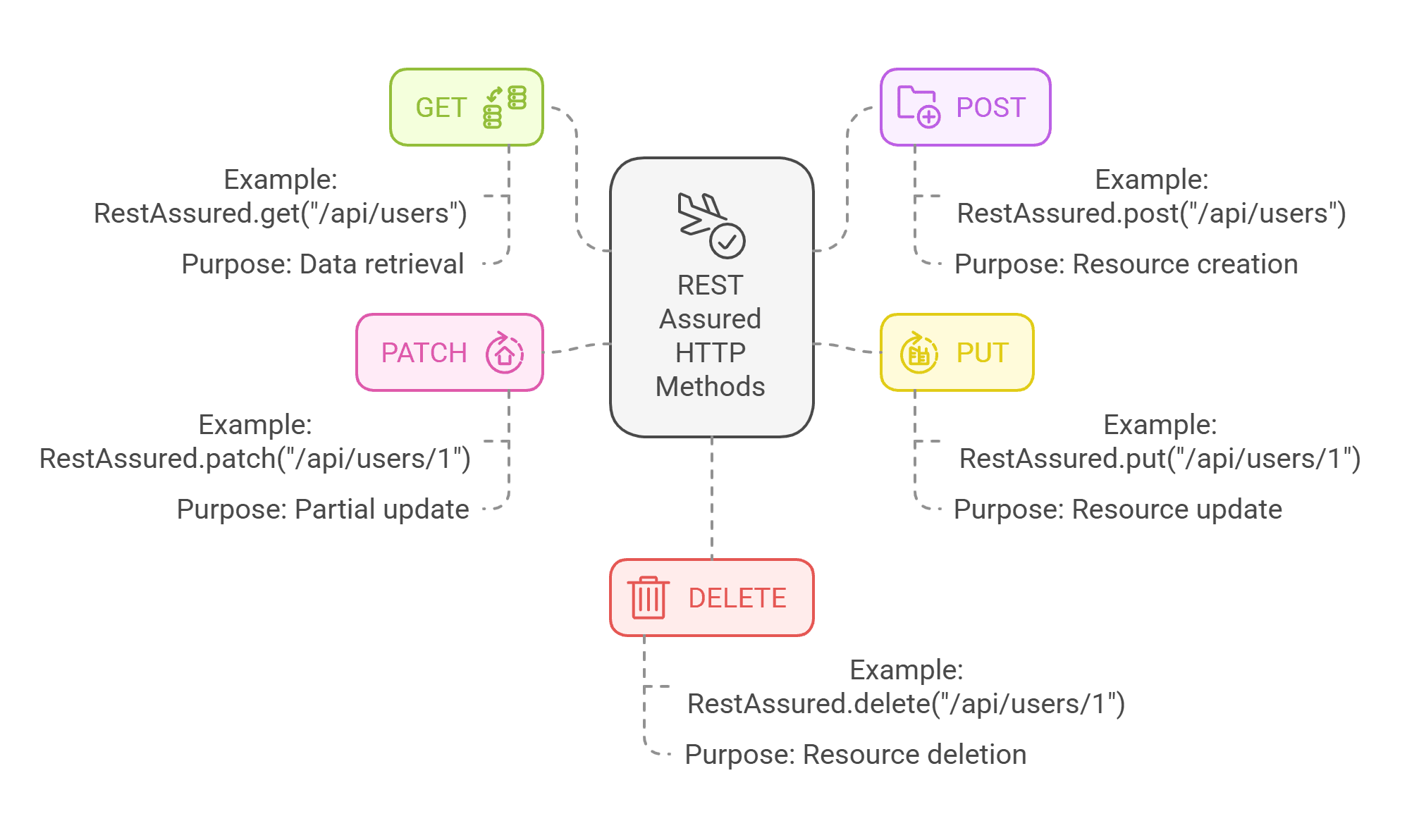
Response Validation
REST Assured shines in its response validation capabilities. Here's a typical validation flow:
java
RestAssured.given() .when() .get("/api/users") .then() .statusCode(200) .time(lessThan(2000L)) // Response time in milliseconds .header("Content-Type", "application/json") .body("name", equalTo("John"));
Status Code and Header Management
Response validation in REST Assured involves checking multiple components:
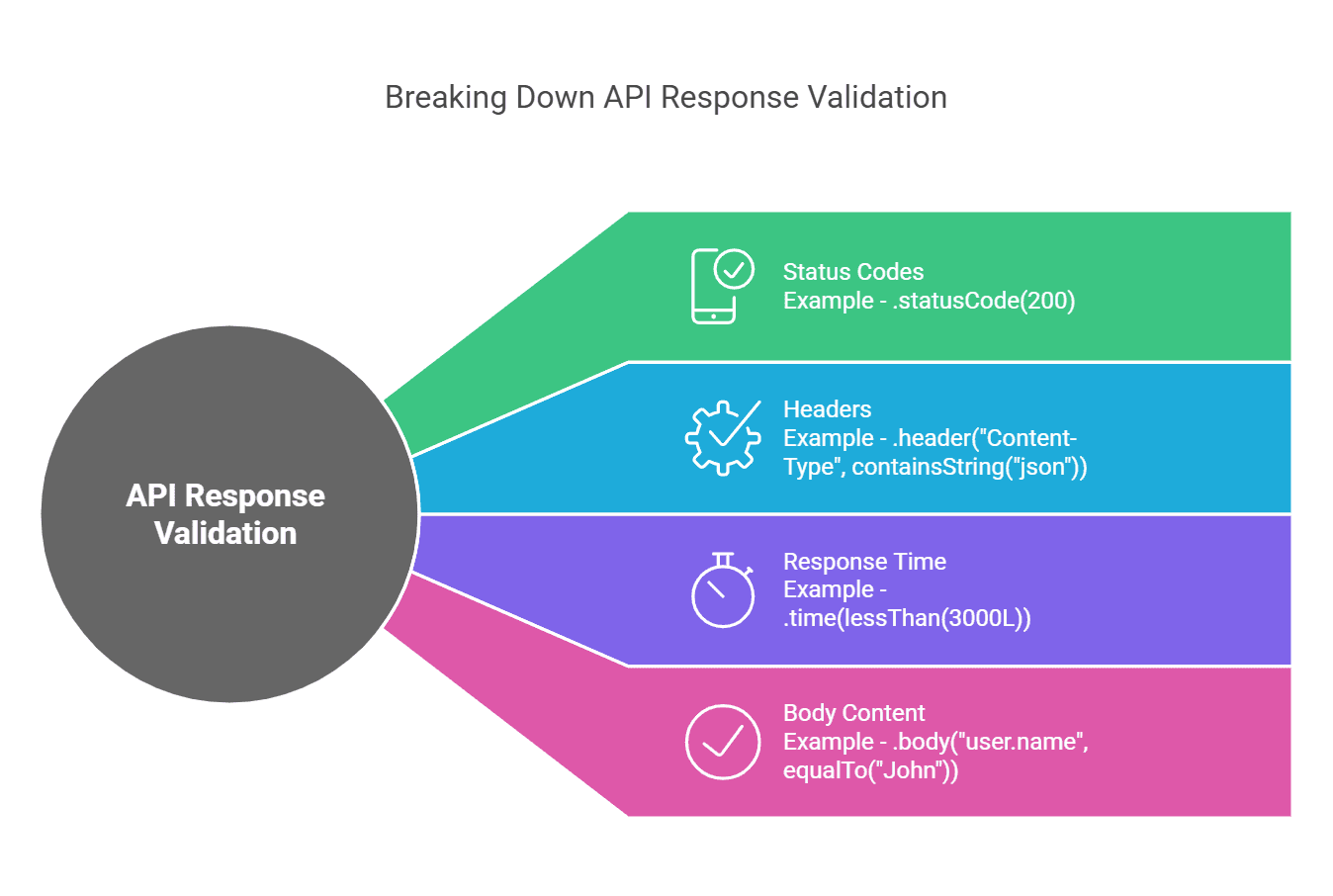
Response validation in REST Assured involves checking multiple components:These components work together in REST Assured to ensure your APIs function correctly and meet performance requirements. Remember to include appropriate assertions for each component based on your testing needs.
By mastering these core components, you'll be able to create comprehensive API tests that verify both functionality and performance using REST Assured's intuitive syntax.
BDD Syntax Structure in REST Assured
The beauty of REST Assured lies in its intuitive Behavior-Driven Development (BDD) syntax. Let's explore how this structured approach makes API testing more readable and maintainable.
Understanding the Flow
REST Assured uses a natural language chain that mirrors how we think about testing:
java
RestAssured.given() .header("Authorization", "Bearer token123") .contentType("application/json") .when() .post("/api/users") .then() .statusCode(201) .body("message", equalTo("User created"));
The Three Pillars of REST Assured
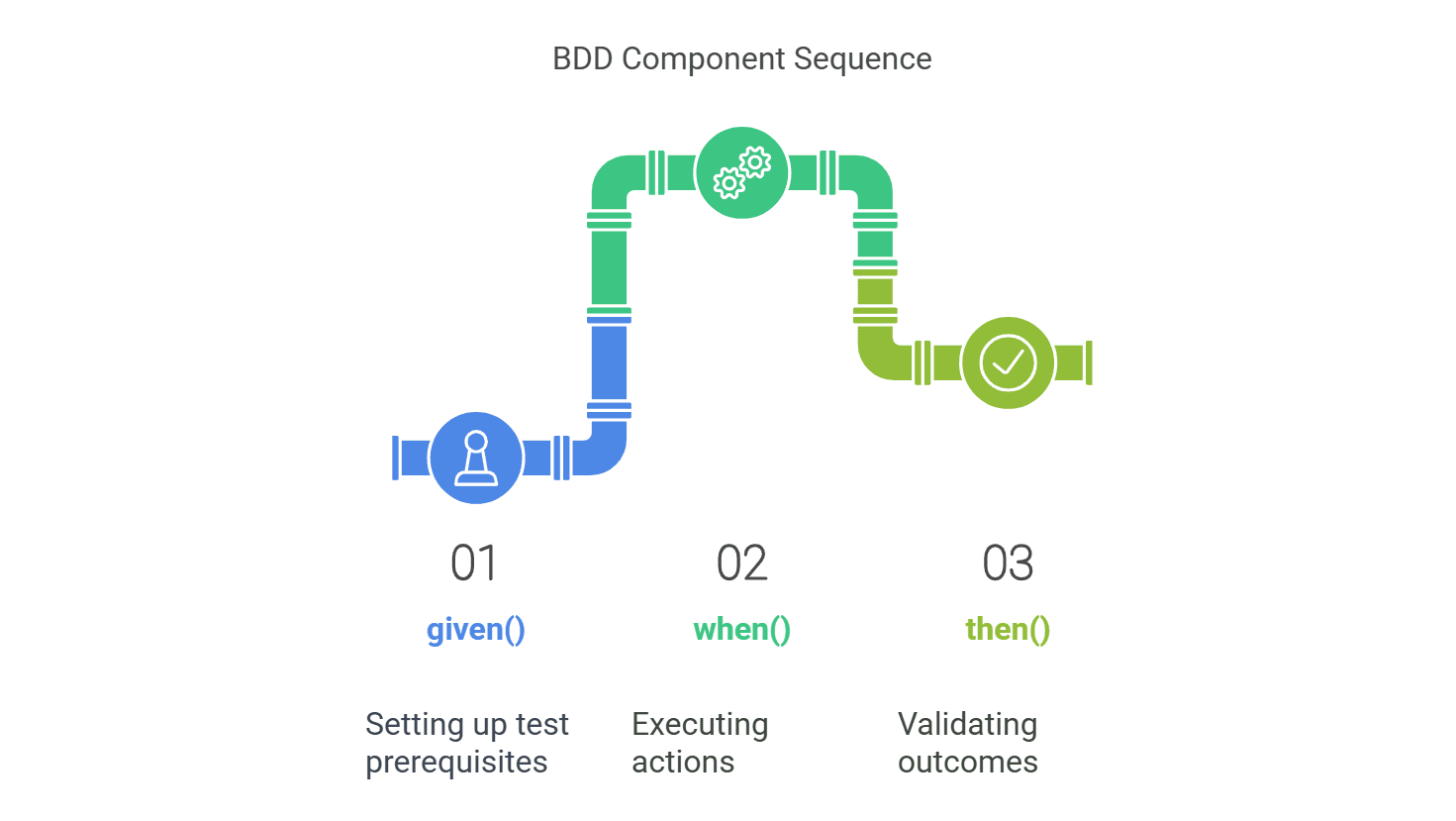
Practical Implementation
Here's how each component works in REST Assured:
given() - The Setup Phase
java
RestAssured.given() .baseUri("https://api.example.com") .header("Content-Type", "application/json") .body({"name": "John", "role": "developer"});
when() - The Action Phase
java
.when() .post("/users");
then() - The Verification Phase
java
.then() .statusCode(201) .body("success", equalTo(true));
This structured approach in REST Assured makes your tests not only easy to write but also simple to maintain and understand. Each section clearly indicates its purpose, making debugging and modifications straightforward.
Remember, while REST Assured follows this BDD pattern, you can be flexible with how you use it based on your testing needs. The key is maintaining readability while ensuring thorough API testing.
Implementation Steps for REST Assured
Let's walk through setting up your first REST Assured project. I'll break down each step to ensure you're ready to start testing APIs effectively.
Creating Your First Project
Setting up a REST Assured project is straightforward. Here's a quick guide to get you started:
1. Maven Project Creation
xml
<project> <groupId>com.apitest</groupId> <artifactId>rest-assured-demo</artifactId> <version>1.0-SNAPSHOT</version> </project>
Essential Dependencies
Your POM file needs these key dependencies for REST Assured:
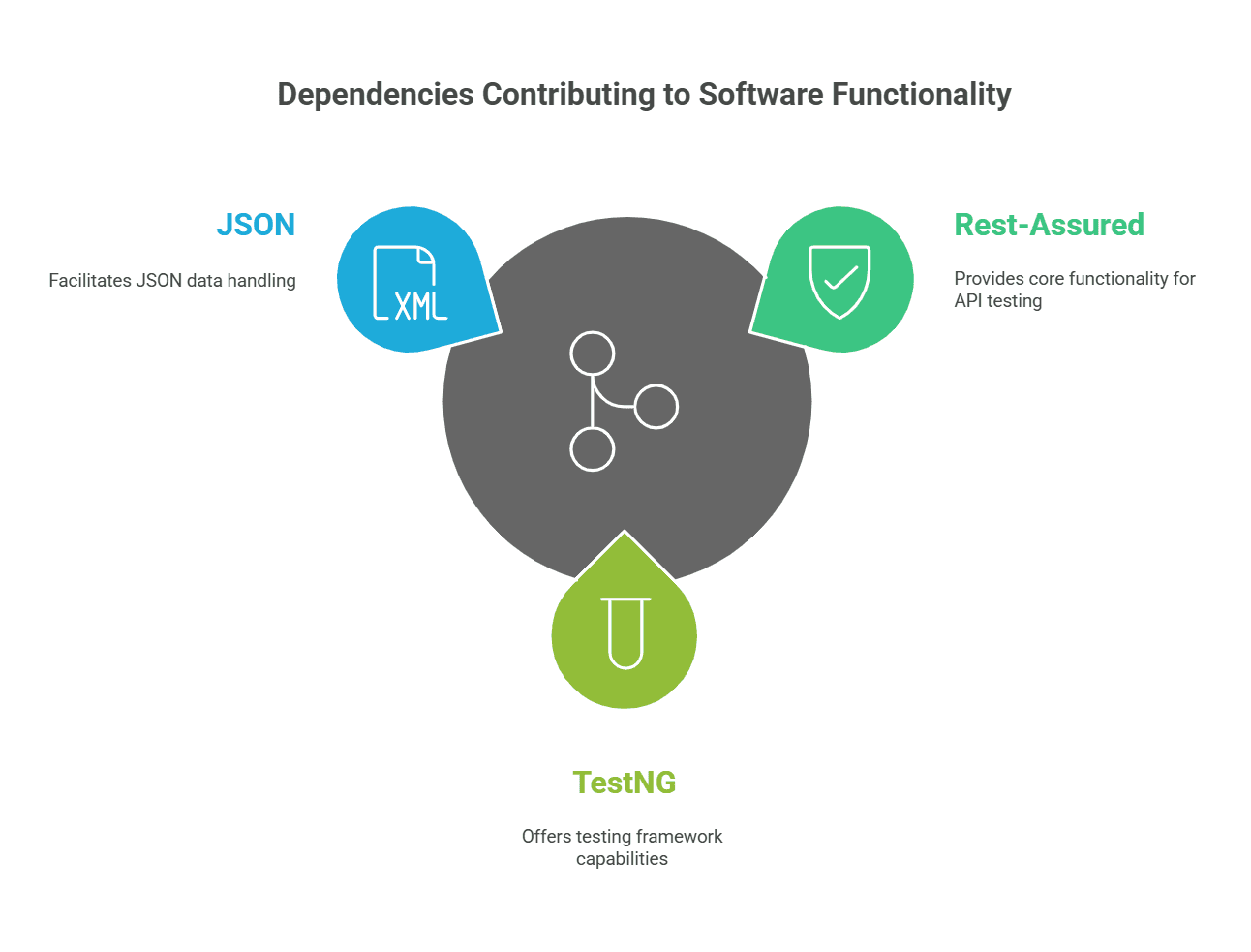
Here's a complete dependency setup:
xml
<dependencies> <!-- REST Assured --> <dependency> <groupId>io.rest-assured</groupId> <artifactId>rest-assured</artifactId> <version>4.4.0</version> <scope>test</scope> </dependency>
<!-- TestNG --> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.4.0</version> <scope>test</scope> </dependency> </dependencies>
Basic Configuration
Once your dependencies are set, configure REST Assured with these essential settings:
java
public class BaseTest { @BeforeClass public void setup() { RestAssured.baseURI = "https://api.example.com"; RestAssured.basePath = "/api/v1"; RestAssured.enableLoggingOfRequestAndResponseIfValidationFails(); } }
This setup provides a foundation for all your REST Assured tests. By extending the BaseTest class, your test classes will inherit these configurations, making your testing process more efficient and organized.
Remember to customize the baseURI and basePath according to your API's requirements. With this setup complete, you're ready to start writing your first REST Assured test cases.
Let's walk through setting up your first REST Assured project. I'll break down each step to ensure you're ready to start testing APIs effectively.
Creating Your First Project
Setting up a REST Assured project is straightforward. Here's a quick guide to get you started:
1. Maven Project Creation
xml
<project> <groupId>com.apitest</groupId> <artifactId>rest-assured-demo</artifactId> <version>1.0-SNAPSHOT</version> </project>
Essential Dependencies
Your POM file needs these key dependencies for REST Assured:
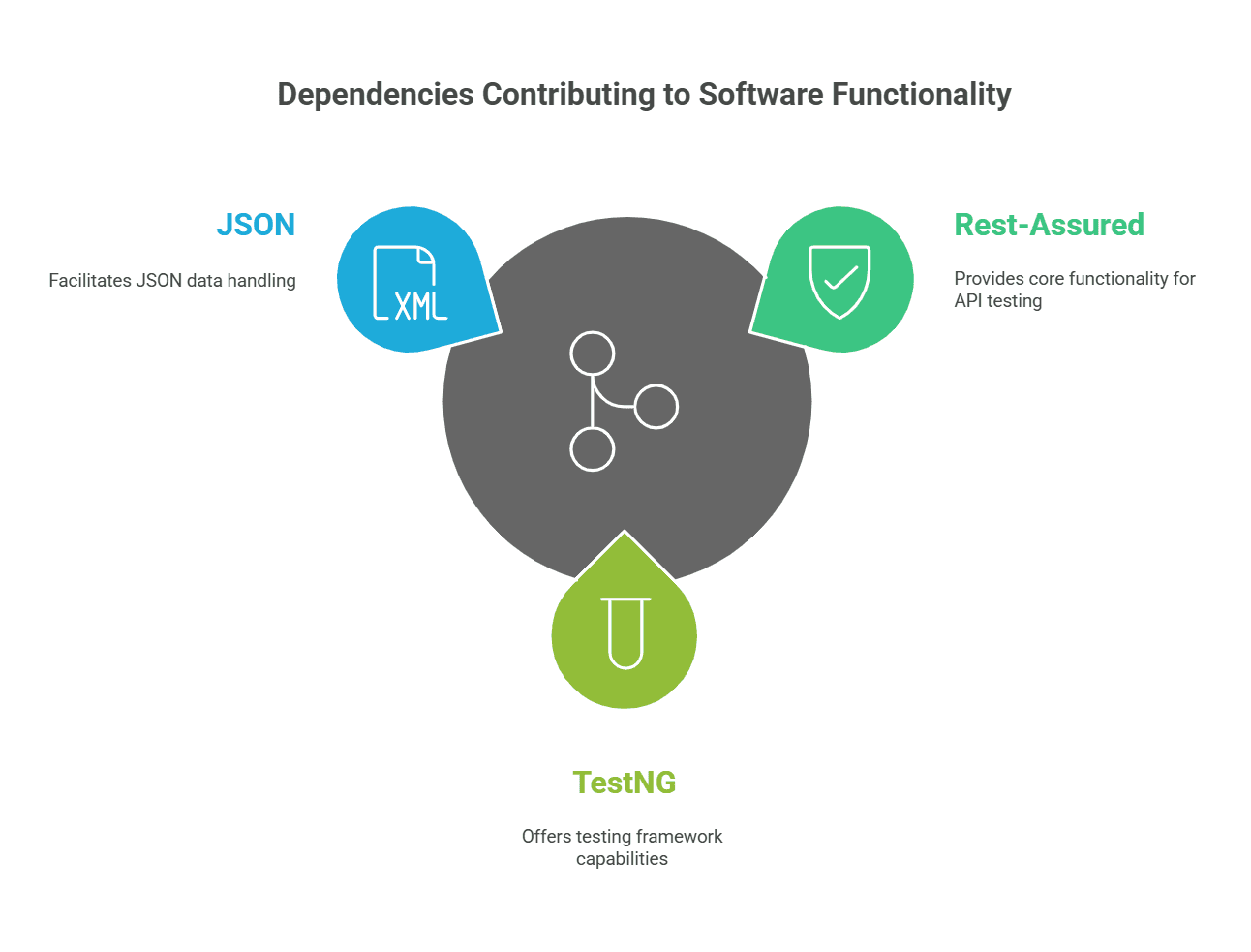
Here's a complete dependency setup:
xml
<dependencies> <!-- REST Assured --> <dependency> <groupId>io.rest-assured</groupId> <artifactId>rest-assured</artifactId> <version>4.4.0</version> <scope>test</scope> </dependency>
<!-- TestNG --> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.4.0</version> <scope>test</scope> </dependency> </dependencies>
Basic Configuration
Once your dependencies are set, configure REST Assured with these essential settings:
java
public class BaseTest { @BeforeClass public void setup() { RestAssured.baseURI = "https://api.example.com"; RestAssured.basePath = "/api/v1"; RestAssured.enableLoggingOfRequestAndResponseIfValidationFails(); } }
This setup provides a foundation for all your REST Assured tests. By extending the BaseTest class, your test classes will inherit these configurations, making your testing process more efficient and organized.
Remember to customize the baseURI and basePath according to your API's requirements. With this setup complete, you're ready to start writing your first REST Assured test cases.
Let's walk through setting up your first REST Assured project. I'll break down each step to ensure you're ready to start testing APIs effectively.
Creating Your First Project
Setting up a REST Assured project is straightforward. Here's a quick guide to get you started:
1. Maven Project Creation
xml
<project> <groupId>com.apitest</groupId> <artifactId>rest-assured-demo</artifactId> <version>1.0-SNAPSHOT</version> </project>
Essential Dependencies
Your POM file needs these key dependencies for REST Assured:
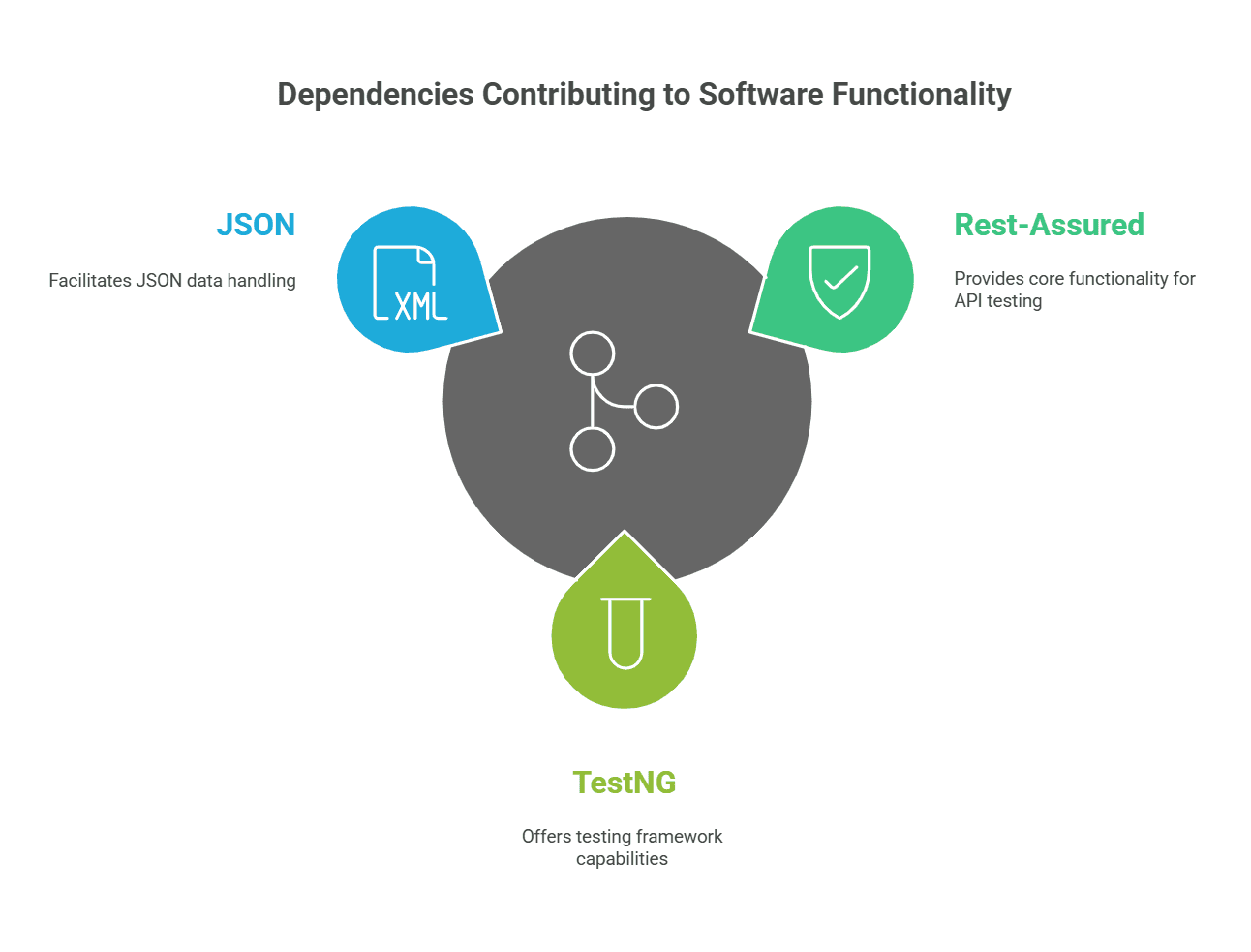
Here's a complete dependency setup:
xml
<dependencies> <!-- REST Assured --> <dependency> <groupId>io.rest-assured</groupId> <artifactId>rest-assured</artifactId> <version>4.4.0</version> <scope>test</scope> </dependency>
<!-- TestNG --> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.4.0</version> <scope>test</scope> </dependency> </dependencies>
Basic Configuration
Once your dependencies are set, configure REST Assured with these essential settings:
java
public class BaseTest { @BeforeClass public void setup() { RestAssured.baseURI = "https://api.example.com"; RestAssured.basePath = "/api/v1"; RestAssured.enableLoggingOfRequestAndResponseIfValidationFails(); } }
This setup provides a foundation for all your REST Assured tests. By extending the BaseTest class, your test classes will inherit these configurations, making your testing process more efficient and organized.
Remember to customize the baseURI and basePath according to your API's requirements. With this setup complete, you're ready to start writing your first REST Assured test cases.
Request Components in REST Assured
Understanding how to structure your requests in REST Assured is crucial for effective API testing. Let's break down each component and see how they work together.
URI Structure
REST Assured uses a clear URI hierarchy:
java
RestAssured.given() .baseUri("https://api.example.com") // Base .basePath("/users") // Resource .pathParam("id", "123") // Path .queryParam("status", "active") // Query .get("/{id}");
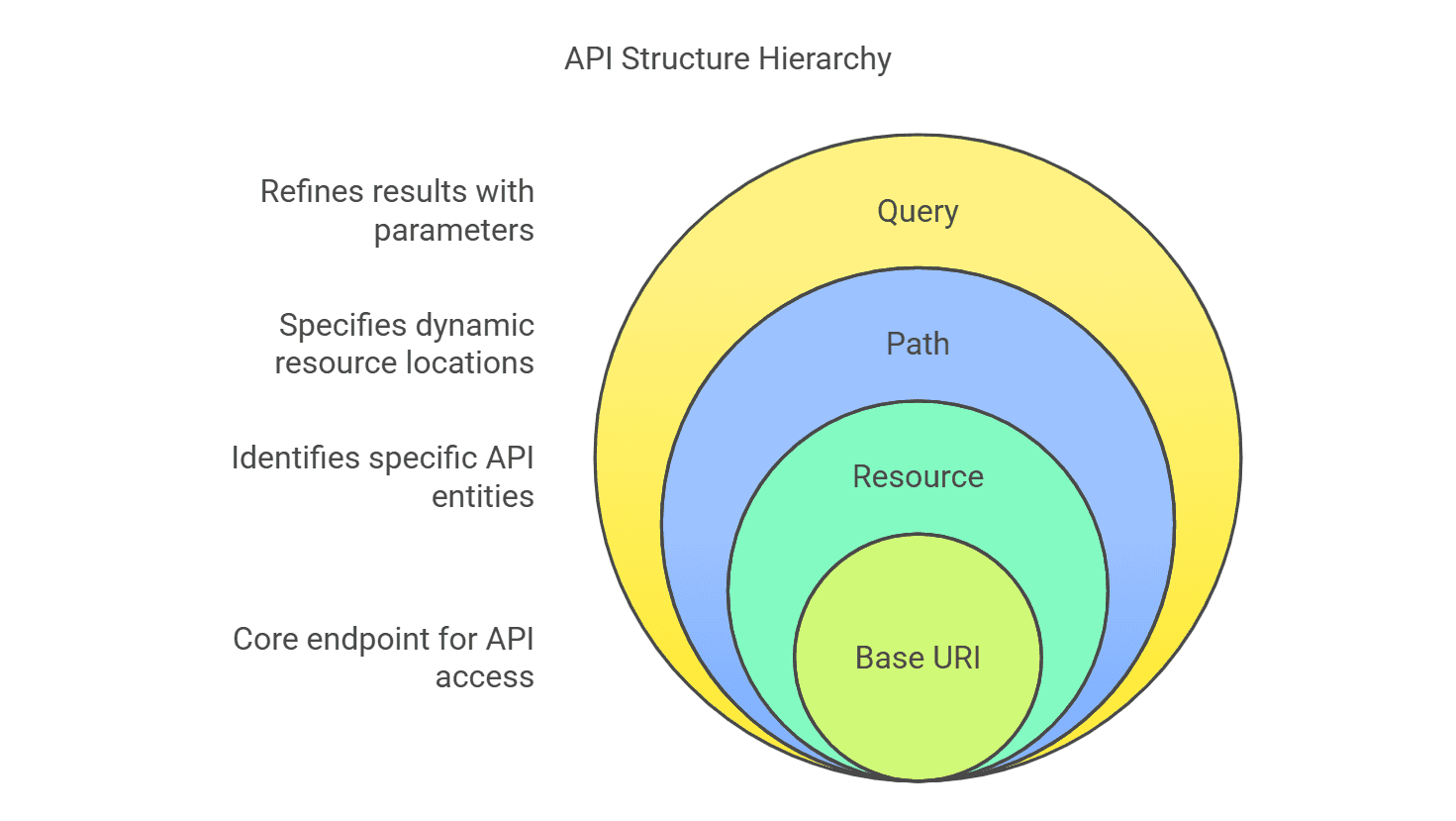
Headers Configuration
Configure headers in REST Assured to handle different content types and authentication:
java
RestAssured.given() .header("Content-Type", "application/json") .header("Accept", "application/json") .header("Authorization", "Bearer " + token)
Request Body Formats
REST Assured supports various request body formats:
java
// JSON Body RestAssured.given() .contentType(ContentType.JSON) .body({ "name": "John", "role": "developer" }) // Form Data RestAssured.given() .contentType(ContentType.URLENC) .formParam("username", "john") .formParam("password", "secret")
Authentication Handling
REST Assured provides multiple authentication methods:
java
// Basic Auth RestAssured.given() .auth() .basic("username", "password") // OAuth 2.0 RestAssured.given() .auth() .oauth2("your-oauth-token") // API Key RestAssured.given() .header("X-API-Key", "your-api-key")
By understanding these components, you can create well-structured and secure requests in REST Assured. Remember to choose the appropriate configuration based on your API's requirements and security needs.
Understanding how to structure your requests in REST Assured is crucial for effective API testing. Let's break down each component and see how they work together.
URI Structure
REST Assured uses a clear URI hierarchy:
java
RestAssured.given() .baseUri("https://api.example.com") // Base .basePath("/users") // Resource .pathParam("id", "123") // Path .queryParam("status", "active") // Query .get("/{id}");
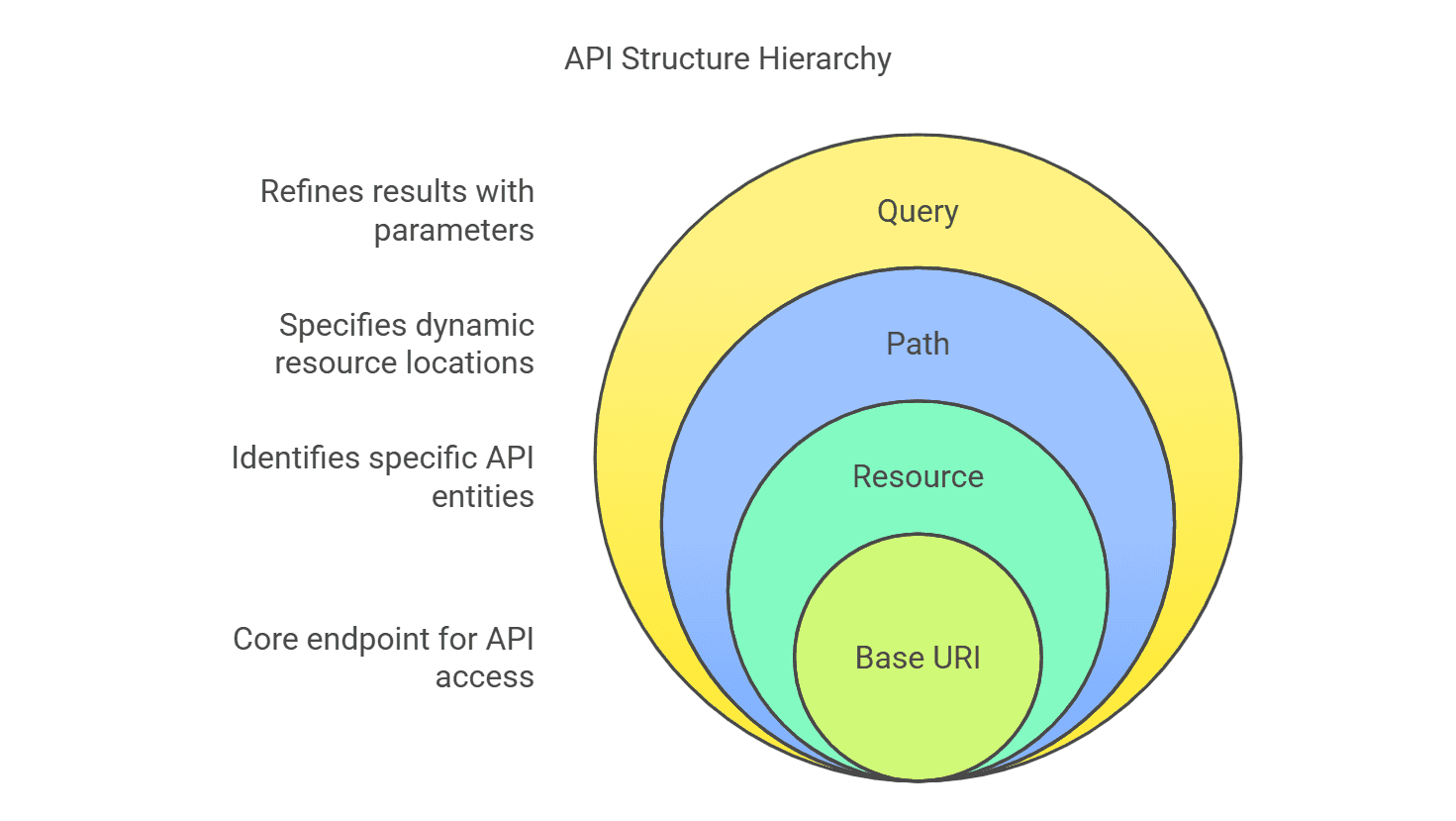
Headers Configuration
Configure headers in REST Assured to handle different content types and authentication:
java
RestAssured.given() .header("Content-Type", "application/json") .header("Accept", "application/json") .header("Authorization", "Bearer " + token)
Request Body Formats
REST Assured supports various request body formats:
java
// JSON Body RestAssured.given() .contentType(ContentType.JSON) .body({ "name": "John", "role": "developer" }) // Form Data RestAssured.given() .contentType(ContentType.URLENC) .formParam("username", "john") .formParam("password", "secret")
Authentication Handling
REST Assured provides multiple authentication methods:
java
// Basic Auth RestAssured.given() .auth() .basic("username", "password") // OAuth 2.0 RestAssured.given() .auth() .oauth2("your-oauth-token") // API Key RestAssured.given() .header("X-API-Key", "your-api-key")
By understanding these components, you can create well-structured and secure requests in REST Assured. Remember to choose the appropriate configuration based on your API's requirements and security needs.
Understanding how to structure your requests in REST Assured is crucial for effective API testing. Let's break down each component and see how they work together.
URI Structure
REST Assured uses a clear URI hierarchy:
java
RestAssured.given() .baseUri("https://api.example.com") // Base .basePath("/users") // Resource .pathParam("id", "123") // Path .queryParam("status", "active") // Query .get("/{id}");
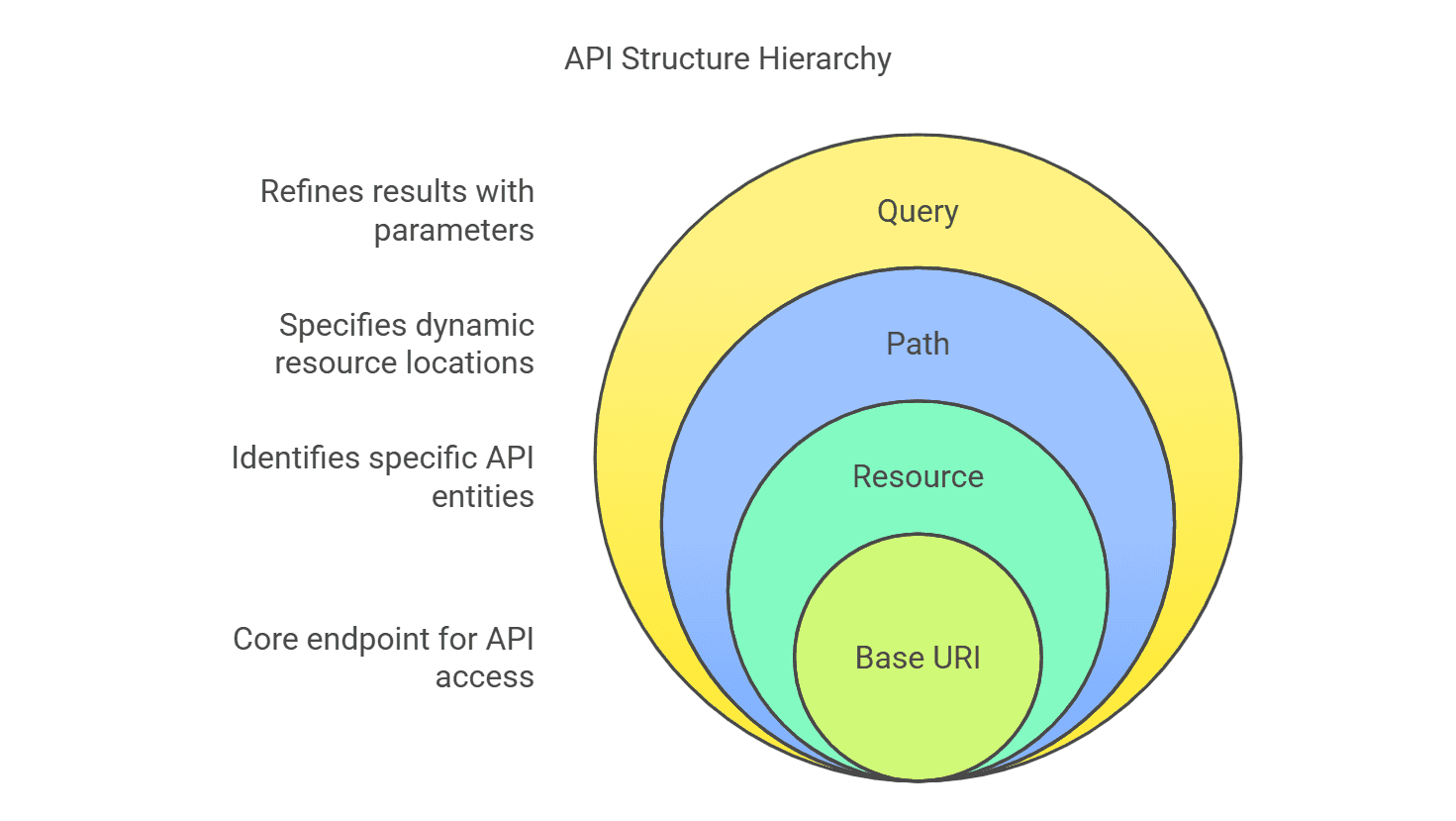
Headers Configuration
Configure headers in REST Assured to handle different content types and authentication:
java
RestAssured.given() .header("Content-Type", "application/json") .header("Accept", "application/json") .header("Authorization", "Bearer " + token)
Request Body Formats
REST Assured supports various request body formats:
java
// JSON Body RestAssured.given() .contentType(ContentType.JSON) .body({ "name": "John", "role": "developer" }) // Form Data RestAssured.given() .contentType(ContentType.URLENC) .formParam("username", "john") .formParam("password", "secret")
Authentication Handling
REST Assured provides multiple authentication methods:
java
// Basic Auth RestAssured.given() .auth() .basic("username", "password") // OAuth 2.0 RestAssured.given() .auth() .oauth2("your-oauth-token") // API Key RestAssured.given() .header("X-API-Key", "your-api-key")
By understanding these components, you can create well-structured and secure requests in REST Assured. Remember to choose the appropriate configuration based on your API's requirements and security needs.
Response Handling in REST Assured
Mastering response handling in REST Assured is essential for robust API testing. Let's explore how to effectively validate responses and handle different scenarios.
Status Code Validation
Here's a comprehensive approach to status code verification in REST Assured:
java
RestAssured.given() .when() .get("/api/users") .then() .assertThat() .statusCode(200) .log().ifError();
Common Response Scenarios
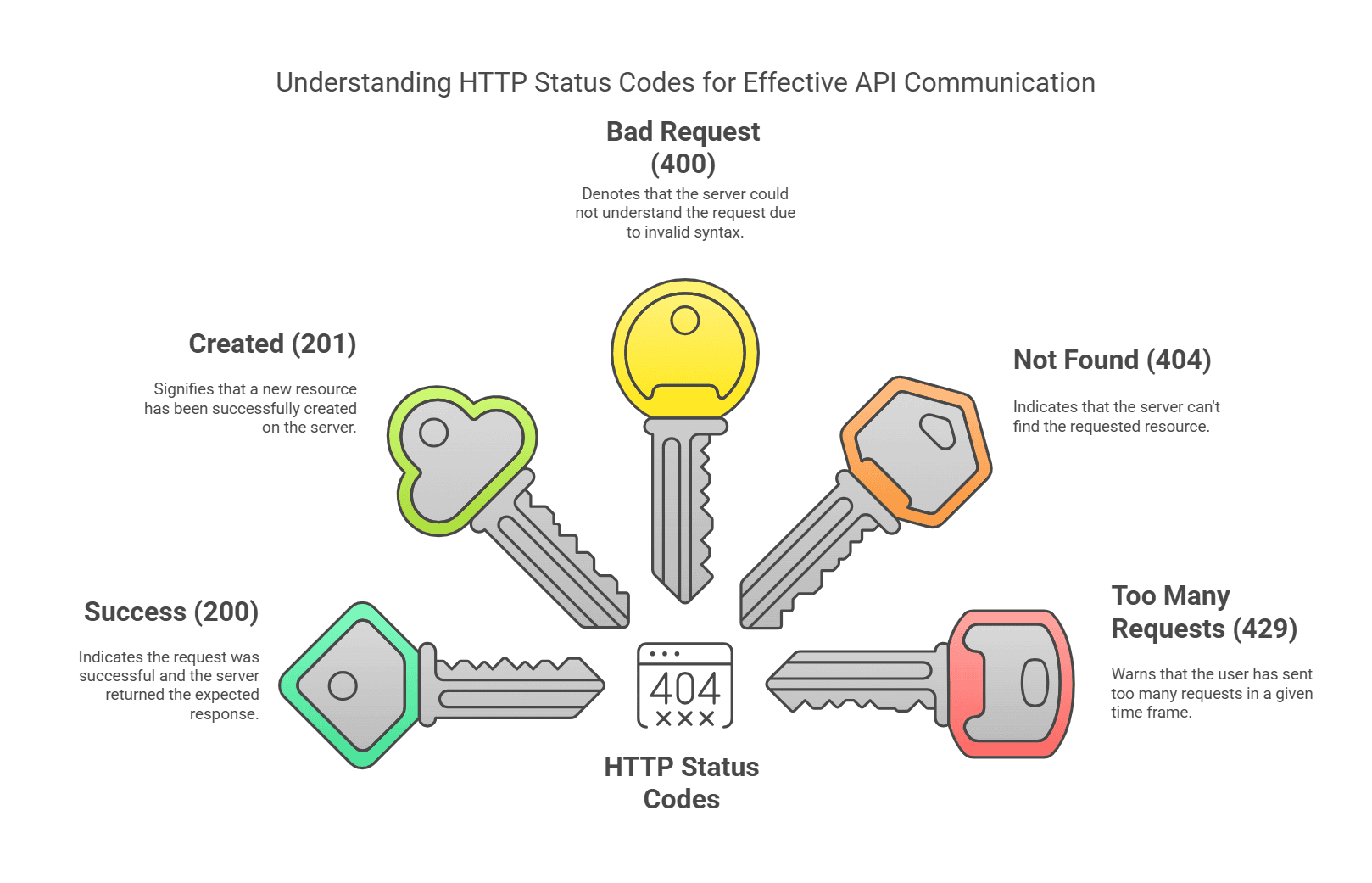
Response Body Validation
REST Assured offers multiple ways to validate response content:
java
RestAssured.given() .when() .get("/api/users/1") .then() .body("name", equalTo("John")) .body("email", containsString("@")) .body("roles.size()", greaterThan(0))
Error Handling Best Practices
Implement robust error handling in REST Assured:
java
try { RestAssured.given() .when() .get("/api/users") .then() .statusCode(200) .body("users", not(empty())); } catch (AssertionError e) { // Log the error System.err.println("API validation failed: " + e.getMessage()); // Custom error handling handleTestFailure(); } catch (Exception e) { // Handle unexpected errors System.err.println("Unexpected error in REST Assured test: " + e.getMessage()); throw e; }
Remember to implement proper logging and error reporting in your REST Assured tests to make debugging easier when issues arise. This structured approach to response handling ensures your API tests are both thorough and maintainable.
Mastering response handling in REST Assured is essential for robust API testing. Let's explore how to effectively validate responses and handle different scenarios.
Status Code Validation
Here's a comprehensive approach to status code verification in REST Assured:
java
RestAssured.given() .when() .get("/api/users") .then() .assertThat() .statusCode(200) .log().ifError();
Common Response Scenarios
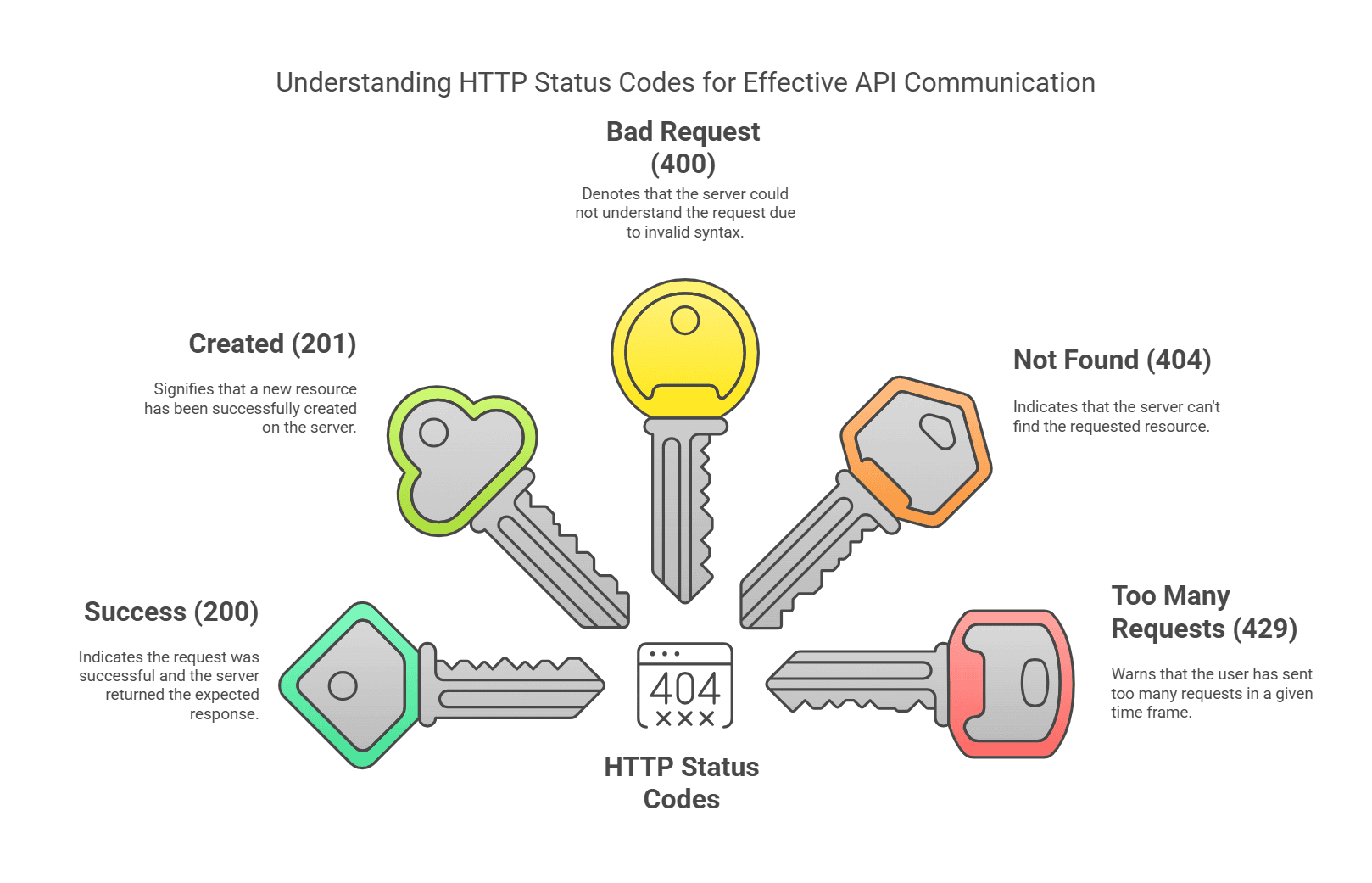
Response Body Validation
REST Assured offers multiple ways to validate response content:
java
RestAssured.given() .when() .get("/api/users/1") .then() .body("name", equalTo("John")) .body("email", containsString("@")) .body("roles.size()", greaterThan(0))
Error Handling Best Practices
Implement robust error handling in REST Assured:
java
try { RestAssured.given() .when() .get("/api/users") .then() .statusCode(200) .body("users", not(empty())); } catch (AssertionError e) { // Log the error System.err.println("API validation failed: " + e.getMessage()); // Custom error handling handleTestFailure(); } catch (Exception e) { // Handle unexpected errors System.err.println("Unexpected error in REST Assured test: " + e.getMessage()); throw e; }
Remember to implement proper logging and error reporting in your REST Assured tests to make debugging easier when issues arise. This structured approach to response handling ensures your API tests are both thorough and maintainable.
Mastering response handling in REST Assured is essential for robust API testing. Let's explore how to effectively validate responses and handle different scenarios.
Status Code Validation
Here's a comprehensive approach to status code verification in REST Assured:
java
RestAssured.given() .when() .get("/api/users") .then() .assertThat() .statusCode(200) .log().ifError();
Common Response Scenarios
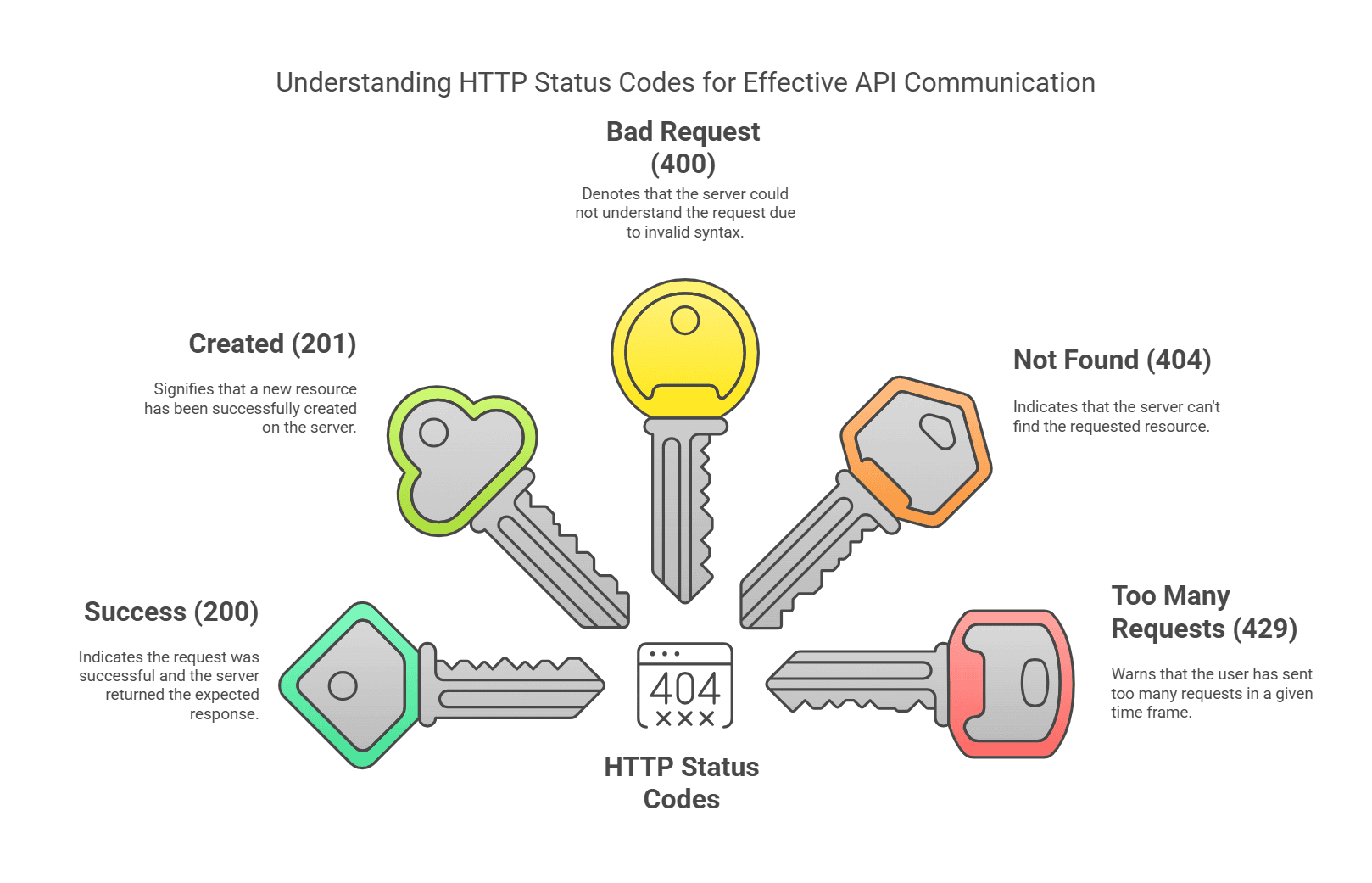
Response Body Validation
REST Assured offers multiple ways to validate response content:
java
RestAssured.given() .when() .get("/api/users/1") .then() .body("name", equalTo("John")) .body("email", containsString("@")) .body("roles.size()", greaterThan(0))
Error Handling Best Practices
Implement robust error handling in REST Assured:
java
try { RestAssured.given() .when() .get("/api/users") .then() .statusCode(200) .body("users", not(empty())); } catch (AssertionError e) { // Log the error System.err.println("API validation failed: " + e.getMessage()); // Custom error handling handleTestFailure(); } catch (Exception e) { // Handle unexpected errors System.err.println("Unexpected error in REST Assured test: " + e.getMessage()); throw e; }
Remember to implement proper logging and error reporting in your REST Assured tests to make debugging easier when issues arise. This structured approach to response handling ensures your API tests are both thorough and maintainable.
Best Practices for REST Assured Testing
Making your REST Assured tests robust and maintainable requires following industry best practices. Let's explore key strategies that will elevate your API testing game.
Framework Integration
REST Assured works best when properly integrated with testing frameworks:
java
@Test(groups = "api") public class ApiTest { private static RequestSpecification requestSpec; @BeforeClass public void setupRestAssured() { requestSpec = RestAssured.given() .baseUri("https://api.example.com") .contentType(ContentType.JSON) .filter(new ResponseLoggingFilter()); } }
Assertion Strategies
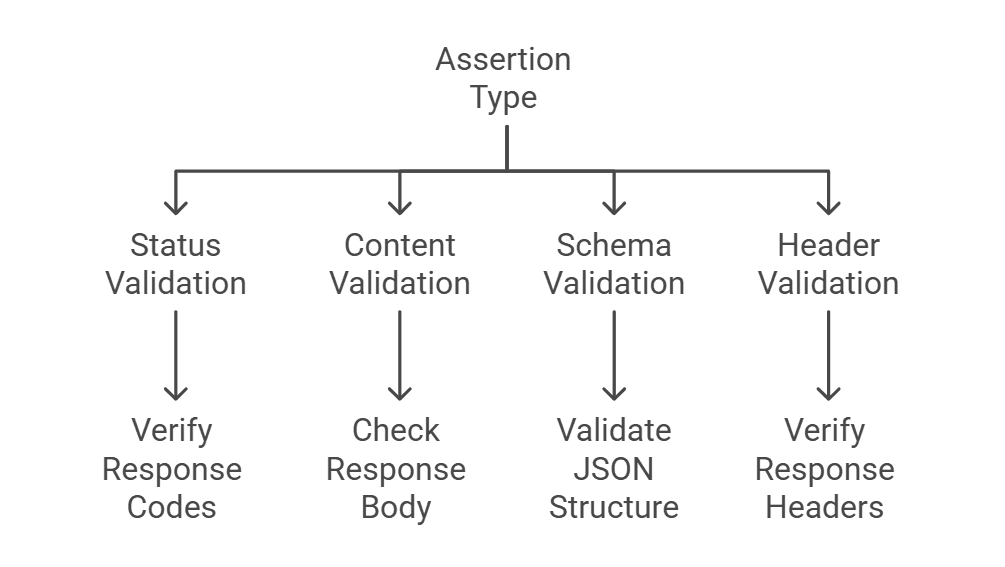
Test Data Management
Organize your test data effectively:
java
public class TestDataManager { private static final String TEST_DATA_PATH = "src/test/resources/testdata/"; public static String getTestData(String fileName) { return new File(TEST_DATA_PATH + fileName) .readText(); } public static RequestSpecification createTestRequest() { return RestAssured.given() .body(getTestData("user.json")); } }
Error Handling Guidelines
Implement comprehensive error handling in REST Assured:
java
@Test public void userApiTest() { try { Response response = RestAssured.given() .spec(requestSpec) .when() .get("/users") .then() .log().ifError() .extract().response(); // Custom validation validateResponse(response); } catch (AssertionError e) { logger.error("API test failed: {}", e.getMessage()); throw e; } }
Remember these key points for maintaining high-quality REST Assured tests:
Keep your test code clean and well-organized
Use reusable components for common operations
Implement proper logging and reporting
Regularly update your REST Assured version for the latest features and security patches
By following these best practices, you'll create more reliable and maintainable API tests with REST Assured.
Making your REST Assured tests robust and maintainable requires following industry best practices. Let's explore key strategies that will elevate your API testing game.
Framework Integration
REST Assured works best when properly integrated with testing frameworks:
java
@Test(groups = "api") public class ApiTest { private static RequestSpecification requestSpec; @BeforeClass public void setupRestAssured() { requestSpec = RestAssured.given() .baseUri("https://api.example.com") .contentType(ContentType.JSON) .filter(new ResponseLoggingFilter()); } }
Assertion Strategies
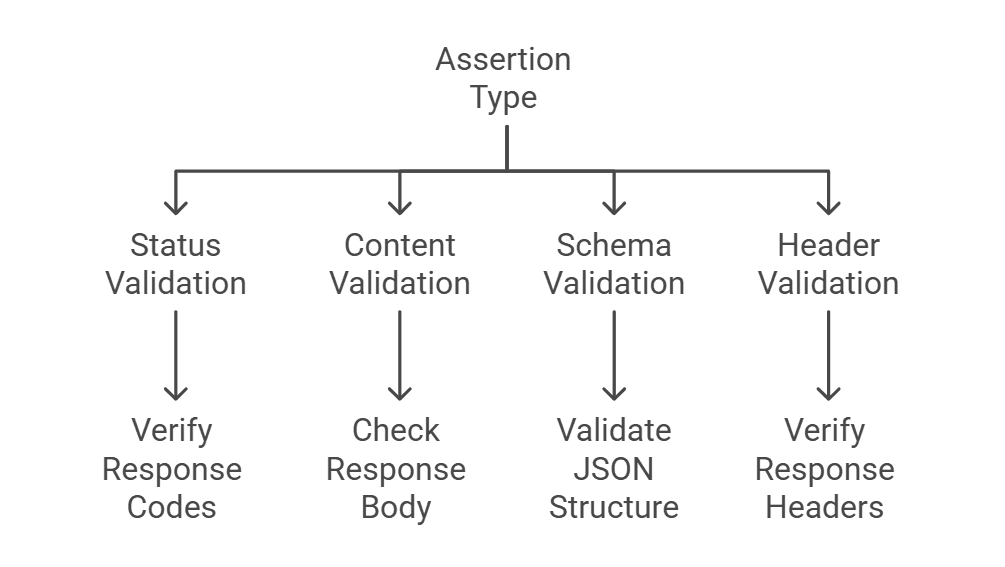
Test Data Management
Organize your test data effectively:
java
public class TestDataManager { private static final String TEST_DATA_PATH = "src/test/resources/testdata/"; public static String getTestData(String fileName) { return new File(TEST_DATA_PATH + fileName) .readText(); } public static RequestSpecification createTestRequest() { return RestAssured.given() .body(getTestData("user.json")); } }
Error Handling Guidelines
Implement comprehensive error handling in REST Assured:
java
@Test public void userApiTest() { try { Response response = RestAssured.given() .spec(requestSpec) .when() .get("/users") .then() .log().ifError() .extract().response(); // Custom validation validateResponse(response); } catch (AssertionError e) { logger.error("API test failed: {}", e.getMessage()); throw e; } }
Remember these key points for maintaining high-quality REST Assured tests:
Keep your test code clean and well-organized
Use reusable components for common operations
Implement proper logging and reporting
Regularly update your REST Assured version for the latest features and security patches
By following these best practices, you'll create more reliable and maintainable API tests with REST Assured.
Making your REST Assured tests robust and maintainable requires following industry best practices. Let's explore key strategies that will elevate your API testing game.
Framework Integration
REST Assured works best when properly integrated with testing frameworks:
java
@Test(groups = "api") public class ApiTest { private static RequestSpecification requestSpec; @BeforeClass public void setupRestAssured() { requestSpec = RestAssured.given() .baseUri("https://api.example.com") .contentType(ContentType.JSON) .filter(new ResponseLoggingFilter()); } }
Assertion Strategies
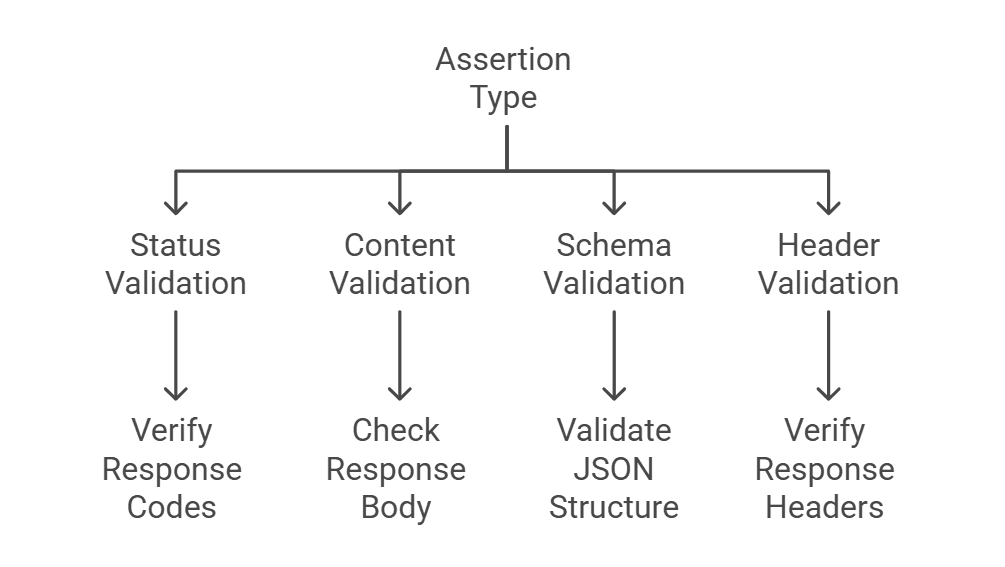
Test Data Management
Organize your test data effectively:
java
public class TestDataManager { private static final String TEST_DATA_PATH = "src/test/resources/testdata/"; public static String getTestData(String fileName) { return new File(TEST_DATA_PATH + fileName) .readText(); } public static RequestSpecification createTestRequest() { return RestAssured.given() .body(getTestData("user.json")); } }
Error Handling Guidelines
Implement comprehensive error handling in REST Assured:
java
@Test public void userApiTest() { try { Response response = RestAssured.given() .spec(requestSpec) .when() .get("/users") .then() .log().ifError() .extract().response(); // Custom validation validateResponse(response); } catch (AssertionError e) { logger.error("API test failed: {}", e.getMessage()); throw e; } }
Remember these key points for maintaining high-quality REST Assured tests:
Keep your test code clean and well-organized
Use reusable components for common operations
Implement proper logging and reporting
Regularly update your REST Assured version for the latest features and security patches
By following these best practices, you'll create more reliable and maintainable API tests with REST Assured.
Advantages of REST Assured
REST Assured has become a go-to choice for API testing, and for good reason. Let's explore the key benefits that make it stand out among other testing frameworks.
Seamless Java Integration
REST Assured's deep integration with Java offers significant advantages:
java
// Example showing Java-native features in REST Assured public class ApiTest { @Test public void demonstrateJavaIntegration() { List<String> userIds = RestAssured.given() .when() .get("/users") .then() .extract() .jsonPath().getList("users.id"); // Use Java streams for processing userIds.stream() .filter(id -> Integer.parseInt(id) > 100) .forEach(this::validateUser); } }
Feature Comparison
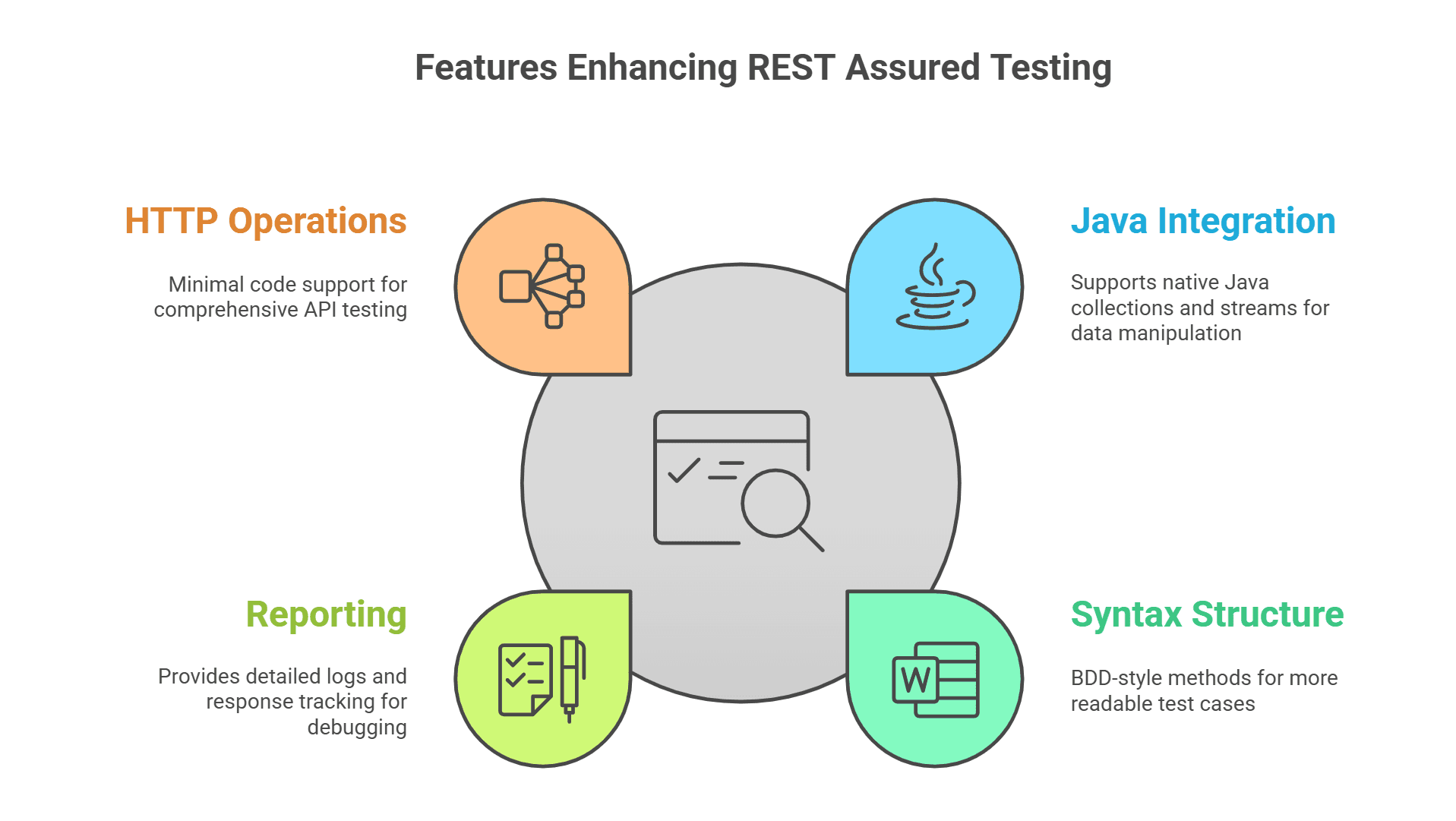
Enhanced Productivity
REST Assured streamlines the testing process:
java
// Example of simplified authentication and validation RestAssured.given() .auth().oauth2(token) .when() .post("/api/data") .then() .log().ifValidationFails() .assertThat() .statusCode(201);
Flexible Response Handling
The framework offers versatile ways to handle API responses:
java
// Extract and process response data Response response = RestAssured.get("/api/users") .then() .extract() .response(); // Use response in multiple ways String responseBody = response.getBody().asString(); JsonPath jsonPath = response.jsonPath(); Headers headers = response.getHeaders();
Remember, REST Assured's combination of simplicity and power makes it an excellent choice for both beginners and experienced testers alike. Its extensive feature set continues to evolve, making it a reliable tool for modern API testing needs.
Conclusion
REST Assured stands out as a powerful tool for API testing, combining Java's robustness with intuitive syntax. Whether you're a beginner starting with API testing or an experienced tester looking for efficient solutions, REST Assured offers the flexibility and features you need. From its seamless BDD approach to comprehensive response validation capabilities, it streamlines the entire testing process. By following the best practices and implementation steps outlined in this guide, you'll be well-equipped to create reliable, maintainable API tests that ensure your applications work flawlessly.
REST Assured has become a go-to choice for API testing, and for good reason. Let's explore the key benefits that make it stand out among other testing frameworks.
Seamless Java Integration
REST Assured's deep integration with Java offers significant advantages:
java
// Example showing Java-native features in REST Assured public class ApiTest { @Test public void demonstrateJavaIntegration() { List<String> userIds = RestAssured.given() .when() .get("/users") .then() .extract() .jsonPath().getList("users.id"); // Use Java streams for processing userIds.stream() .filter(id -> Integer.parseInt(id) > 100) .forEach(this::validateUser); } }
Feature Comparison
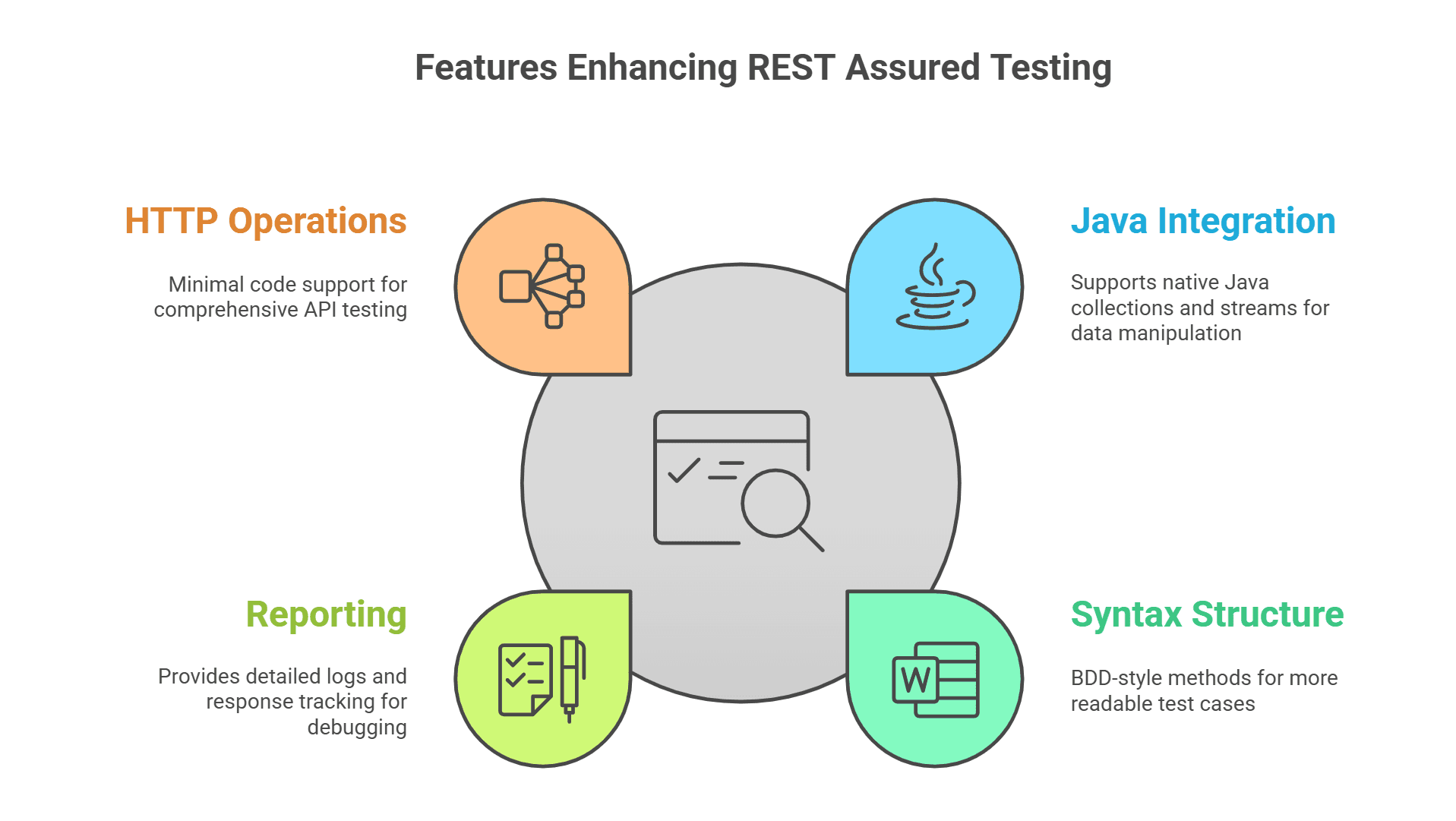
Enhanced Productivity
REST Assured streamlines the testing process:
java
// Example of simplified authentication and validation RestAssured.given() .auth().oauth2(token) .when() .post("/api/data") .then() .log().ifValidationFails() .assertThat() .statusCode(201);
Flexible Response Handling
The framework offers versatile ways to handle API responses:
java
// Extract and process response data Response response = RestAssured.get("/api/users") .then() .extract() .response(); // Use response in multiple ways String responseBody = response.getBody().asString(); JsonPath jsonPath = response.jsonPath(); Headers headers = response.getHeaders();
Remember, REST Assured's combination of simplicity and power makes it an excellent choice for both beginners and experienced testers alike. Its extensive feature set continues to evolve, making it a reliable tool for modern API testing needs.
Conclusion
REST Assured stands out as a powerful tool for API testing, combining Java's robustness with intuitive syntax. Whether you're a beginner starting with API testing or an experienced tester looking for efficient solutions, REST Assured offers the flexibility and features you need. From its seamless BDD approach to comprehensive response validation capabilities, it streamlines the entire testing process. By following the best practices and implementation steps outlined in this guide, you'll be well-equipped to create reliable, maintainable API tests that ensure your applications work flawlessly.
REST Assured has become a go-to choice for API testing, and for good reason. Let's explore the key benefits that make it stand out among other testing frameworks.
Seamless Java Integration
REST Assured's deep integration with Java offers significant advantages:
java
// Example showing Java-native features in REST Assured public class ApiTest { @Test public void demonstrateJavaIntegration() { List<String> userIds = RestAssured.given() .when() .get("/users") .then() .extract() .jsonPath().getList("users.id"); // Use Java streams for processing userIds.stream() .filter(id -> Integer.parseInt(id) > 100) .forEach(this::validateUser); } }
Feature Comparison
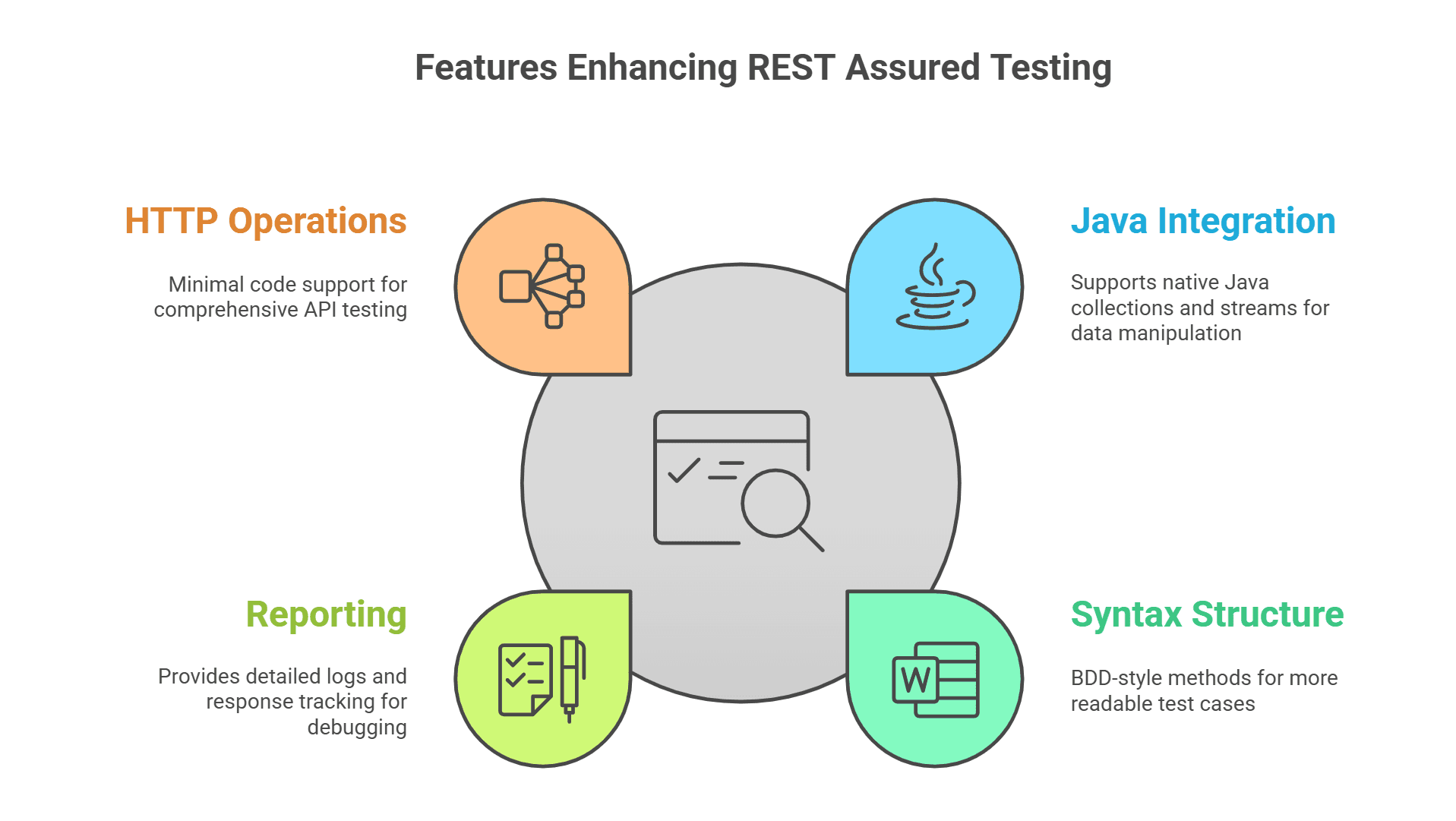
Enhanced Productivity
REST Assured streamlines the testing process:
java
// Example of simplified authentication and validation RestAssured.given() .auth().oauth2(token) .when() .post("/api/data") .then() .log().ifValidationFails() .assertThat() .statusCode(201);
Flexible Response Handling
The framework offers versatile ways to handle API responses:
java
// Extract and process response data Response response = RestAssured.get("/api/users") .then() .extract() .response(); // Use response in multiple ways String responseBody = response.getBody().asString(); JsonPath jsonPath = response.jsonPath(); Headers headers = response.getHeaders();
Remember, REST Assured's combination of simplicity and power makes it an excellent choice for both beginners and experienced testers alike. Its extensive feature set continues to evolve, making it a reliable tool for modern API testing needs.
Conclusion
REST Assured stands out as a powerful tool for API testing, combining Java's robustness with intuitive syntax. Whether you're a beginner starting with API testing or an experienced tester looking for efficient solutions, REST Assured offers the flexibility and features you need. From its seamless BDD approach to comprehensive response validation capabilities, it streamlines the entire testing process. By following the best practices and implementation steps outlined in this guide, you'll be well-equipped to create reliable, maintainable API tests that ensure your applications work flawlessly.
FAQs
Why should you choose Qodex.ai?
Why should you choose Qodex.ai?
Why should you choose Qodex.ai?
How can I validate an email address using Python regex?
How can I validate an email address using Python regex?
How can I validate an email address using Python regex?
What is Go Regex Tester?
What is Go Regex Tester?
What is Go Regex Tester?
Remommended posts
Discover, Test, and Secure your APIs — 10x Faster.

Product
All Rights Reserved.
Copyright © 2025 Qodex
Discover, Test, and Secure your APIs — 10x Faster.

Product
All Rights Reserved.
Copyright © 2025 Qodex
Discover, Test, and Secure your APIs — 10x Faster.

Product
All Rights Reserved.
Copyright © 2025 Qodex