Getting Started With ZOOM API in Python
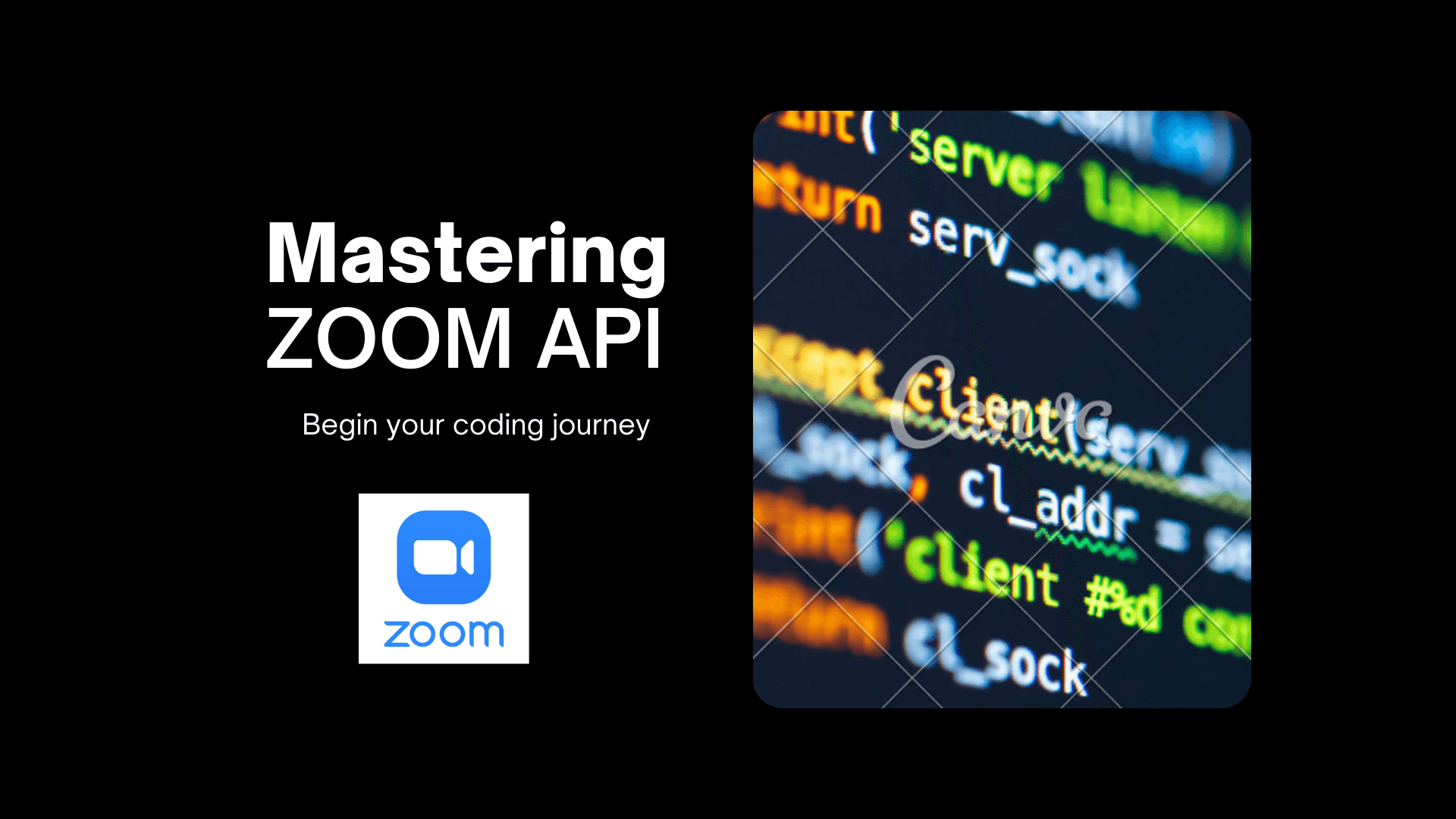
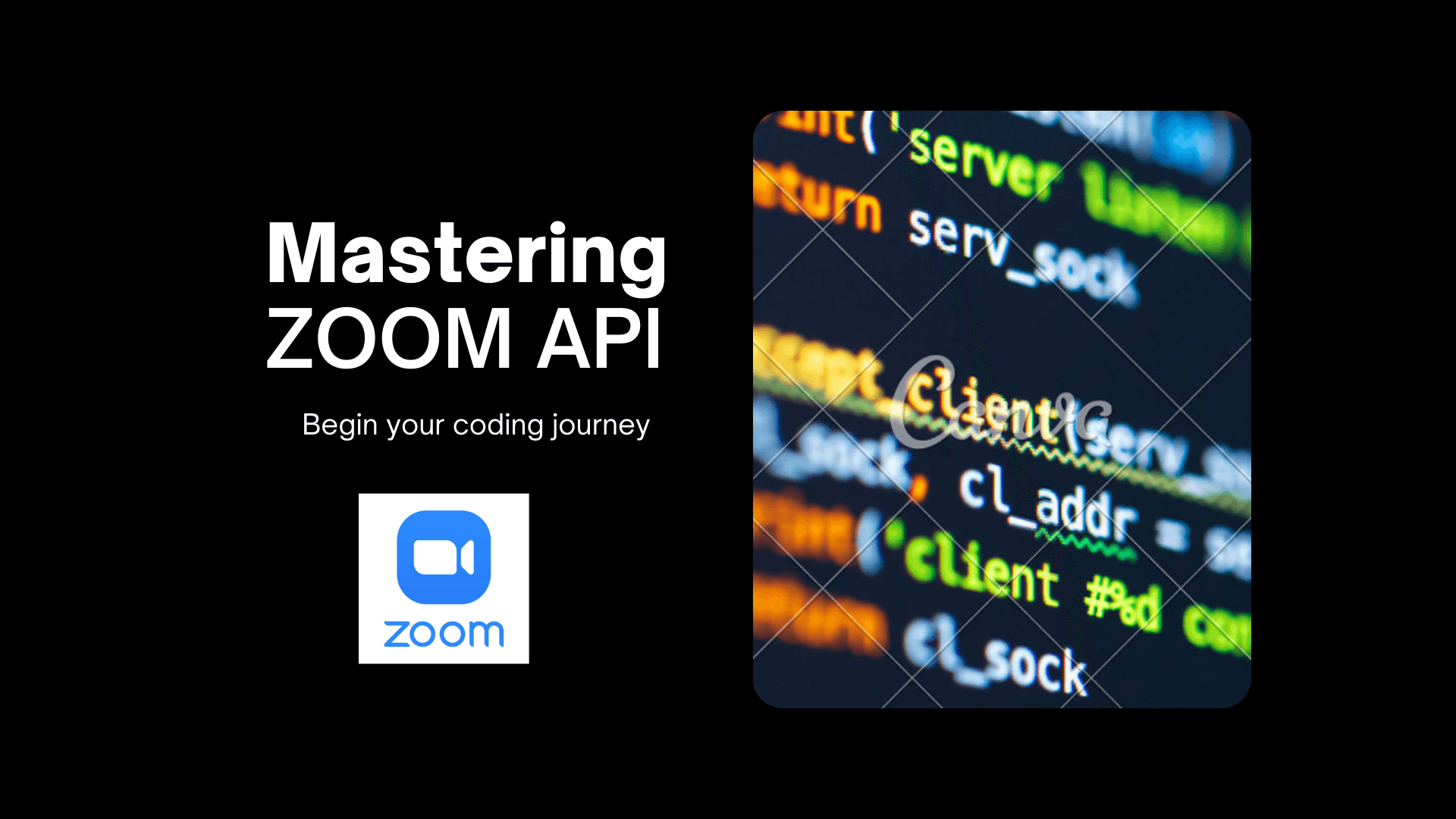
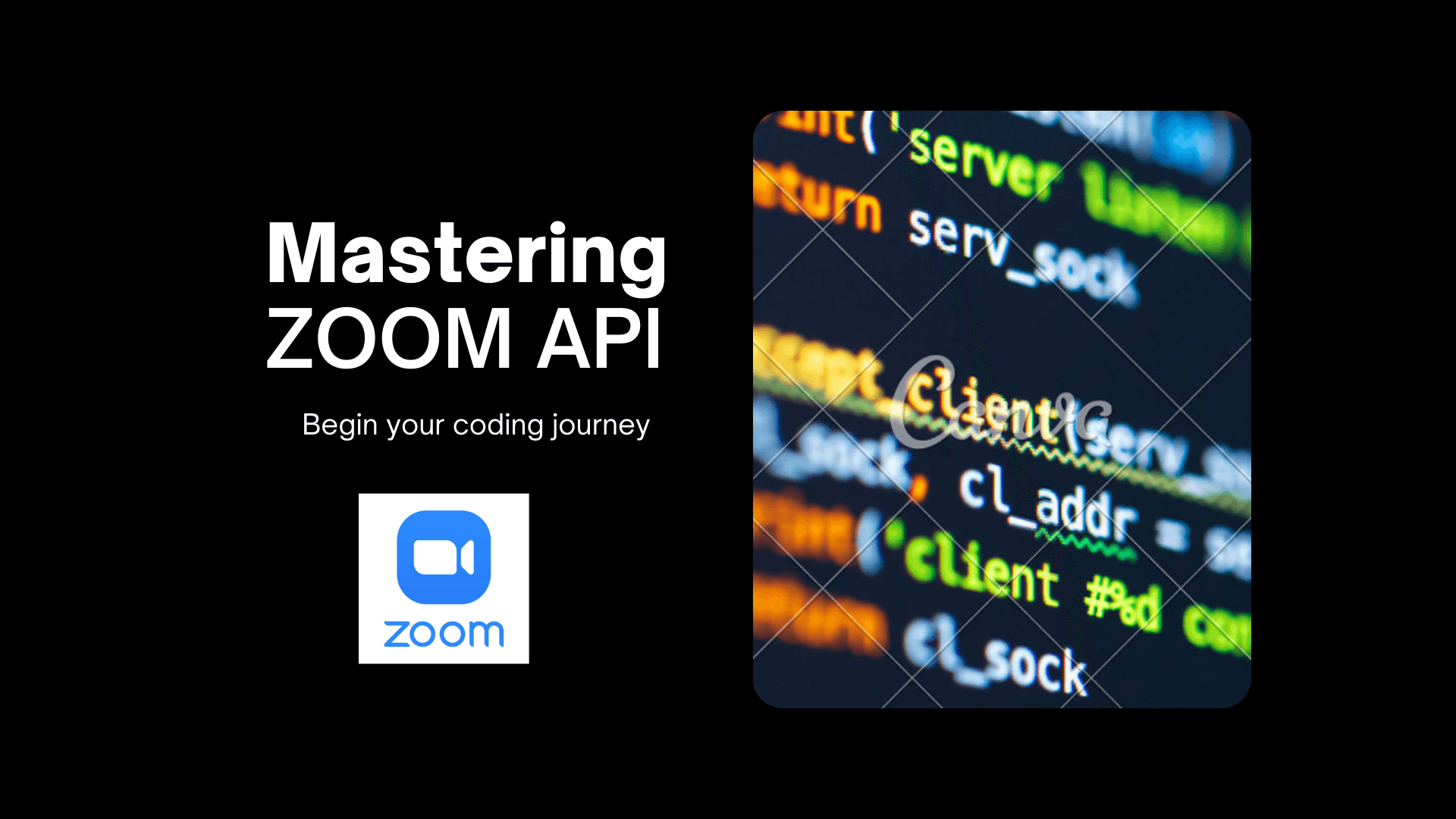
Introduction
Hey there, tech enthusiasts! Ever wondered how to automate your Zoom meetings using Python? Well, you're in for a treat! Today, we're diving into the world of Zoom API and how you can harness its power with just a few lines of Python code.
Zoom has become an integral part of our daily lives, whether for work, education, or catching up with friends. But did you know you can create, manage, and customize Zoom meetings programmatically? That's where the Zoom API comes in handy, and Python makes it a breeze to work with.
In this guide, we'll walk you through the process of setting up your Zoom API account, writing some simple Python code, and creating your very first Zoom meeting through API calls. Whether you're a Python newbie or a seasoned coder, this tutorial will have you automating Zoom tasks in no time.
So, grab your favorite caffeinated beverage, fire up your code editor, and let's get started on this exciting journey into the world of Zoom API and Python!
Hey there, tech enthusiasts! Ever wondered how to automate your Zoom meetings using Python? Well, you're in for a treat! Today, we're diving into the world of Zoom API and how you can harness its power with just a few lines of Python code.
Zoom has become an integral part of our daily lives, whether for work, education, or catching up with friends. But did you know you can create, manage, and customize Zoom meetings programmatically? That's where the Zoom API comes in handy, and Python makes it a breeze to work with.
In this guide, we'll walk you through the process of setting up your Zoom API account, writing some simple Python code, and creating your very first Zoom meeting through API calls. Whether you're a Python newbie or a seasoned coder, this tutorial will have you automating Zoom tasks in no time.
So, grab your favorite caffeinated beverage, fire up your code editor, and let's get started on this exciting journey into the world of Zoom API and Python!
Hey there, tech enthusiasts! Ever wondered how to automate your Zoom meetings using Python? Well, you're in for a treat! Today, we're diving into the world of Zoom API and how you can harness its power with just a few lines of Python code.
Zoom has become an integral part of our daily lives, whether for work, education, or catching up with friends. But did you know you can create, manage, and customize Zoom meetings programmatically? That's where the Zoom API comes in handy, and Python makes it a breeze to work with.
In this guide, we'll walk you through the process of setting up your Zoom API account, writing some simple Python code, and creating your very first Zoom meeting through API calls. Whether you're a Python newbie or a seasoned coder, this tutorial will have you automating Zoom tasks in no time.
So, grab your favorite caffeinated beverage, fire up your code editor, and let's get started on this exciting journey into the world of Zoom API and Python!
Setting Up Your Zoom API Account
Alright, let's kick things off by getting your Zoom API account ready for action. Don't worry, it's easier than you might think!
Creating a Zoom Account First things first, you'll need a Zoom account. If you already have one, great! If not, head over to zoom.us and sign up. It's free and takes just a minute.
Navigating to the Zoom Marketplace Once you're logged in, it's time to visit the Zoom App Marketplace. Here's how:
Go to marketplace.zoom.us
Click on the "Develop" dropdown in the top-right corner
Select "Build App"
You'll feel like a kid in a candy store with all the options, but stay focused!
Building a JWT App Now, let's create your app:
Click on "Create" under the JWT option
You'll see a pop-up about Zoom's API License and Terms of Use. Give it a quick read and click "I agree"
Time to name your app! Choose something cool (or boring, we won't judge)
Fill in some basic details like your name and email
Obtaining API Key and Secret You're in the home stretch now:
After creating your app, navigate to the "App Credentials" tab
Here you'll find your API Key and API Secret
Keep these safe! They're like the secret ingredients to your API recipe
Pro tip: Never share your API credentials publicly. It's like giving away the keys to your digital kingdom!
And there you have it! Your Zoom API account is set up and ready to go. Pat yourself on the back – you've just taken the first step towards Zoom automation mastery.
Alright, let's kick things off by getting your Zoom API account ready for action. Don't worry, it's easier than you might think!
Creating a Zoom Account First things first, you'll need a Zoom account. If you already have one, great! If not, head over to zoom.us and sign up. It's free and takes just a minute.
Navigating to the Zoom Marketplace Once you're logged in, it's time to visit the Zoom App Marketplace. Here's how:
Go to marketplace.zoom.us
Click on the "Develop" dropdown in the top-right corner
Select "Build App"
You'll feel like a kid in a candy store with all the options, but stay focused!
Building a JWT App Now, let's create your app:
Click on "Create" under the JWT option
You'll see a pop-up about Zoom's API License and Terms of Use. Give it a quick read and click "I agree"
Time to name your app! Choose something cool (or boring, we won't judge)
Fill in some basic details like your name and email
Obtaining API Key and Secret You're in the home stretch now:
After creating your app, navigate to the "App Credentials" tab
Here you'll find your API Key and API Secret
Keep these safe! They're like the secret ingredients to your API recipe
Pro tip: Never share your API credentials publicly. It's like giving away the keys to your digital kingdom!
And there you have it! Your Zoom API account is set up and ready to go. Pat yourself on the back – you've just taken the first step towards Zoom automation mastery.
Alright, let's kick things off by getting your Zoom API account ready for action. Don't worry, it's easier than you might think!
Creating a Zoom Account First things first, you'll need a Zoom account. If you already have one, great! If not, head over to zoom.us and sign up. It's free and takes just a minute.
Navigating to the Zoom Marketplace Once you're logged in, it's time to visit the Zoom App Marketplace. Here's how:
Go to marketplace.zoom.us
Click on the "Develop" dropdown in the top-right corner
Select "Build App"
You'll feel like a kid in a candy store with all the options, but stay focused!
Building a JWT App Now, let's create your app:
Click on "Create" under the JWT option
You'll see a pop-up about Zoom's API License and Terms of Use. Give it a quick read and click "I agree"
Time to name your app! Choose something cool (or boring, we won't judge)
Fill in some basic details like your name and email
Obtaining API Key and Secret You're in the home stretch now:
After creating your app, navigate to the "App Credentials" tab
Here you'll find your API Key and API Secret
Keep these safe! They're like the secret ingredients to your API recipe
Pro tip: Never share your API credentials publicly. It's like giving away the keys to your digital kingdom!
And there you have it! Your Zoom API account is set up and ready to go. Pat yourself on the back – you've just taken the first step towards Zoom automation mastery.

Ship bug-free software, 200% faster, in 20% testing budget. No coding required

Ship bug-free software, 200% faster, in 20% testing budget. No coding required

Ship bug-free software, 200% faster, in 20% testing budget. No coding required
Installing Required Python Libraries and Generating a JWT Token
Now that we've got our Zoom API account set up, it's time to get our hands dirty with some Python magic! Let's start by installing the necessary libraries and then move on to generating our JWT token.
Installing Required Python Libraries
Before we dive into the code, we need to equip our Python environment with two essential libraries:
Requests library: This is our go-to for making HTTP requests. It's like the Swiss Army knife for interacting with web APIs.
PyJWT library: This little gem will help us generate our JSON Web Tokens (JWT) for authentication.
To install these libraries, open your terminal and run:
pip install requests pyjwt
Easy peasy, right? Now we're ready to roll!
Generating a JWT Token
Alright, let's create a function to generate our JWT token. This token is like a VIP pass that lets us access the Zoom API. Here's how we do it:
import jwt from time import time API_KEY = 'Your_API_Key_Here' API_SEC = 'Your_API_Secret_Here' def generate_token(): token = jwt.encode( {'iss': API_KEY, 'exp': time() + 5000}, API_SEC, algorithm='HS256' )
Let's break this down:
We import the necessary modules: jwt for token generation and time for setting the expiration.
We define our API_KEY and API_SEC variables. Remember to replace these with your actual credentials!
Our generate_token() function does the heavy lifting:
It creates a payload with two components:
'iss': This is our API Key, which identifies our app to Zoom.
'exp': This sets the expiration time for our token (current time + 5000 seconds).
We use our API Secret to sign the token.
We specify the hashing algorithm as 'HS256'.
And voilà! We've got ourselves a shiny new JWT token generator.
This token is like a temporary password that proves to Zoom that we're authorized to use the API. It's more secure than sending our API key and secret with every request.
Creating a Zoom Meeting and Handling the API Response
Now that we've got our token generator up and running, let's dive into the fun part – creating a Zoom meeting with just a few lines of code!
Creating a Zoom Meeting
First, we'll define our meeting details in JSON format. This is like filling out a form for Zoom, telling it what kind of meeting we want:
import json meeting_details = { "topic": "Python Zoom Meeting", "type": 2, # Scheduled meeting "start_time": "2023-06-14T10:00:00", # Use your desired date and time "duration": "45", # Duration in minutes "timezone": "America/New_York", "agenda": "Discuss Zoom API Integration", "settings": { "host_video": True, "participant_video": True, "join_before_host": False, "mute_upon_entry": True, "watermark": False, "audio": "voip", "auto_recording": "none" } }
Now, let's create a function to send this to Zoom's API:
import requests def create_meeting(): headers = { 'authorization': 'Bearer ' + generate_token(), 'content-type': 'application/json' } r = requests.post( f'https://api.zoom.us/v2/users/me/meetings', headers=headers, data=json.dumps(meeting_details) ) return r.json()
This function does a few key things:
It sets up the headers, including our JWT token.
It sends a POST request to Zoom's API endpoint.
It includes our meeting details in the request.
Handling the API Response
def display_meeting_info(): response = create_meeting() join_url = response['join_url'] meeting_password = response['password'] print(f"Your meeting has been created!") print(f"Join URL: {join_url}") print(f"Password: {meeting_password}") # Let's run it! display_meeting_info()
And there you have it! When you run this script, it will create a Zoom meeting and print out the join URL and password. It's that simple!
You've just created your first Zoom meeting using Python and the Zoom API. How cool is that? With this foundation, you can start building more complex applications – maybe a meeting scheduler, or even a custom Zoom client. The possibilities are endless!
Complete Code Example and Running the Script
Alright, let's put it all together! Here's the complete Python script that combines all the steps we've covered. This is your one-stop shop for creating Zoom meetings programmatically:
import jwt import requests import json from time import time # Your Zoom API credentials API_KEY = 'Your_API_Key_Here' API_SEC = 'Your_API_Secret_Here' # Function to generate a JWT token def generate_token(): token = jwt.encode( {'iss': API_KEY, 'exp': time() + 5000}, API_SEC, algorithm='HS256' ) return token # Meeting details meeting_details = { "topic": "Python Zoom Meeting", "type": 2, # Scheduled meeting "start_time": "2023-06-14T10:00:00", # Use your desired date and time "duration": "45", # Duration in minutes "timezone": "America/New_York", "agenda": "Discuss Zoom API Integration", "settings": { "host_video": True, "participant_video": True, "join_before_host": False, "mute_upon_entry": True, "watermark": False, "audio": "voip", "auto_recording": "none" } } # Function to create a meeting def create_meeting(): headers = { 'authorization': 'Bearer ' + generate_token(), 'content-type': 'application/json' } r = requests.post( f'https://api.zoom.us/v2/users/me/meetings', headers=headers, data=json.dumps(meeting_details) ) return r.json() # Function to display meeting info def display_meeting_info(): response = create_meeting() join_url = response['join_url'] meeting_password = response['password'] print(f"Your meeting has been created!") print(f"Join URL: {join_url}") print(f"Password: {meeting_password}") # Run the script if __name__ == "__main__": display_meeting_info()
Running the Script
Now for the moment of truth! Let's run this script and see the magic happen.
Save the above code in a file, let's call it zoom_meeting_creator.py.
Open your terminal or command prompt.
Navigate to the directory where you saved the file.
Run the script by typing:
python zoom_meeting_creator.py
Understanding the Output
When you run the script, you should see output similar to this:
Your meeting has been created! Join URL: https://us04web.zoom.us/j/12345678901?pwd=abcdefghijklmnop Password: 123456
Let's break down what's happening:
The script generates a JWT token for authentication.
It sends a request to Zoom's API to create a meeting with your specified details.
Zoom responds with the meeting information.
The script extracts and displays the Join URL and Password.
The Join URL is what you'd share with participants to join your meeting. The password adds an extra layer of security.
And there you have it! You've just created a Zoom meeting programmatically. Pretty neat, huh?
Remember, this is just the tip of the iceberg. You can expand this script to create multiple meetings, schedule recurring meetings, or even integrate it into a larger application. The possibilities are endless!
Now that we've got our Zoom API account set up, it's time to get our hands dirty with some Python magic! Let's start by installing the necessary libraries and then move on to generating our JWT token.
Installing Required Python Libraries
Before we dive into the code, we need to equip our Python environment with two essential libraries:
Requests library: This is our go-to for making HTTP requests. It's like the Swiss Army knife for interacting with web APIs.
PyJWT library: This little gem will help us generate our JSON Web Tokens (JWT) for authentication.
To install these libraries, open your terminal and run:
pip install requests pyjwt
Easy peasy, right? Now we're ready to roll!
Generating a JWT Token
Alright, let's create a function to generate our JWT token. This token is like a VIP pass that lets us access the Zoom API. Here's how we do it:
import jwt from time import time API_KEY = 'Your_API_Key_Here' API_SEC = 'Your_API_Secret_Here' def generate_token(): token = jwt.encode( {'iss': API_KEY, 'exp': time() + 5000}, API_SEC, algorithm='HS256' )
Let's break this down:
We import the necessary modules: jwt for token generation and time for setting the expiration.
We define our API_KEY and API_SEC variables. Remember to replace these with your actual credentials!
Our generate_token() function does the heavy lifting:
It creates a payload with two components:
'iss': This is our API Key, which identifies our app to Zoom.
'exp': This sets the expiration time for our token (current time + 5000 seconds).
We use our API Secret to sign the token.
We specify the hashing algorithm as 'HS256'.
And voilà! We've got ourselves a shiny new JWT token generator.
This token is like a temporary password that proves to Zoom that we're authorized to use the API. It's more secure than sending our API key and secret with every request.
Creating a Zoom Meeting and Handling the API Response
Now that we've got our token generator up and running, let's dive into the fun part – creating a Zoom meeting with just a few lines of code!
Creating a Zoom Meeting
First, we'll define our meeting details in JSON format. This is like filling out a form for Zoom, telling it what kind of meeting we want:
import json meeting_details = { "topic": "Python Zoom Meeting", "type": 2, # Scheduled meeting "start_time": "2023-06-14T10:00:00", # Use your desired date and time "duration": "45", # Duration in minutes "timezone": "America/New_York", "agenda": "Discuss Zoom API Integration", "settings": { "host_video": True, "participant_video": True, "join_before_host": False, "mute_upon_entry": True, "watermark": False, "audio": "voip", "auto_recording": "none" } }
Now, let's create a function to send this to Zoom's API:
import requests def create_meeting(): headers = { 'authorization': 'Bearer ' + generate_token(), 'content-type': 'application/json' } r = requests.post( f'https://api.zoom.us/v2/users/me/meetings', headers=headers, data=json.dumps(meeting_details) ) return r.json()
This function does a few key things:
It sets up the headers, including our JWT token.
It sends a POST request to Zoom's API endpoint.
It includes our meeting details in the request.
Handling the API Response
def display_meeting_info(): response = create_meeting() join_url = response['join_url'] meeting_password = response['password'] print(f"Your meeting has been created!") print(f"Join URL: {join_url}") print(f"Password: {meeting_password}") # Let's run it! display_meeting_info()
And there you have it! When you run this script, it will create a Zoom meeting and print out the join URL and password. It's that simple!
You've just created your first Zoom meeting using Python and the Zoom API. How cool is that? With this foundation, you can start building more complex applications – maybe a meeting scheduler, or even a custom Zoom client. The possibilities are endless!
Complete Code Example and Running the Script
Alright, let's put it all together! Here's the complete Python script that combines all the steps we've covered. This is your one-stop shop for creating Zoom meetings programmatically:
import jwt import requests import json from time import time # Your Zoom API credentials API_KEY = 'Your_API_Key_Here' API_SEC = 'Your_API_Secret_Here' # Function to generate a JWT token def generate_token(): token = jwt.encode( {'iss': API_KEY, 'exp': time() + 5000}, API_SEC, algorithm='HS256' ) return token # Meeting details meeting_details = { "topic": "Python Zoom Meeting", "type": 2, # Scheduled meeting "start_time": "2023-06-14T10:00:00", # Use your desired date and time "duration": "45", # Duration in minutes "timezone": "America/New_York", "agenda": "Discuss Zoom API Integration", "settings": { "host_video": True, "participant_video": True, "join_before_host": False, "mute_upon_entry": True, "watermark": False, "audio": "voip", "auto_recording": "none" } } # Function to create a meeting def create_meeting(): headers = { 'authorization': 'Bearer ' + generate_token(), 'content-type': 'application/json' } r = requests.post( f'https://api.zoom.us/v2/users/me/meetings', headers=headers, data=json.dumps(meeting_details) ) return r.json() # Function to display meeting info def display_meeting_info(): response = create_meeting() join_url = response['join_url'] meeting_password = response['password'] print(f"Your meeting has been created!") print(f"Join URL: {join_url}") print(f"Password: {meeting_password}") # Run the script if __name__ == "__main__": display_meeting_info()
Running the Script
Now for the moment of truth! Let's run this script and see the magic happen.
Save the above code in a file, let's call it zoom_meeting_creator.py.
Open your terminal or command prompt.
Navigate to the directory where you saved the file.
Run the script by typing:
python zoom_meeting_creator.py
Understanding the Output
When you run the script, you should see output similar to this:
Your meeting has been created! Join URL: https://us04web.zoom.us/j/12345678901?pwd=abcdefghijklmnop Password: 123456
Let's break down what's happening:
The script generates a JWT token for authentication.
It sends a request to Zoom's API to create a meeting with your specified details.
Zoom responds with the meeting information.
The script extracts and displays the Join URL and Password.
The Join URL is what you'd share with participants to join your meeting. The password adds an extra layer of security.
And there you have it! You've just created a Zoom meeting programmatically. Pretty neat, huh?
Remember, this is just the tip of the iceberg. You can expand this script to create multiple meetings, schedule recurring meetings, or even integrate it into a larger application. The possibilities are endless!
Now that we've got our Zoom API account set up, it's time to get our hands dirty with some Python magic! Let's start by installing the necessary libraries and then move on to generating our JWT token.
Installing Required Python Libraries
Before we dive into the code, we need to equip our Python environment with two essential libraries:
Requests library: This is our go-to for making HTTP requests. It's like the Swiss Army knife for interacting with web APIs.
PyJWT library: This little gem will help us generate our JSON Web Tokens (JWT) for authentication.
To install these libraries, open your terminal and run:
pip install requests pyjwt
Easy peasy, right? Now we're ready to roll!
Generating a JWT Token
Alright, let's create a function to generate our JWT token. This token is like a VIP pass that lets us access the Zoom API. Here's how we do it:
import jwt from time import time API_KEY = 'Your_API_Key_Here' API_SEC = 'Your_API_Secret_Here' def generate_token(): token = jwt.encode( {'iss': API_KEY, 'exp': time() + 5000}, API_SEC, algorithm='HS256' )
Let's break this down:
We import the necessary modules: jwt for token generation and time for setting the expiration.
We define our API_KEY and API_SEC variables. Remember to replace these with your actual credentials!
Our generate_token() function does the heavy lifting:
It creates a payload with two components:
'iss': This is our API Key, which identifies our app to Zoom.
'exp': This sets the expiration time for our token (current time + 5000 seconds).
We use our API Secret to sign the token.
We specify the hashing algorithm as 'HS256'.
And voilà! We've got ourselves a shiny new JWT token generator.
This token is like a temporary password that proves to Zoom that we're authorized to use the API. It's more secure than sending our API key and secret with every request.
Creating a Zoom Meeting and Handling the API Response
Now that we've got our token generator up and running, let's dive into the fun part – creating a Zoom meeting with just a few lines of code!
Creating a Zoom Meeting
First, we'll define our meeting details in JSON format. This is like filling out a form for Zoom, telling it what kind of meeting we want:
import json meeting_details = { "topic": "Python Zoom Meeting", "type": 2, # Scheduled meeting "start_time": "2023-06-14T10:00:00", # Use your desired date and time "duration": "45", # Duration in minutes "timezone": "America/New_York", "agenda": "Discuss Zoom API Integration", "settings": { "host_video": True, "participant_video": True, "join_before_host": False, "mute_upon_entry": True, "watermark": False, "audio": "voip", "auto_recording": "none" } }
Now, let's create a function to send this to Zoom's API:
import requests def create_meeting(): headers = { 'authorization': 'Bearer ' + generate_token(), 'content-type': 'application/json' } r = requests.post( f'https://api.zoom.us/v2/users/me/meetings', headers=headers, data=json.dumps(meeting_details) ) return r.json()
This function does a few key things:
It sets up the headers, including our JWT token.
It sends a POST request to Zoom's API endpoint.
It includes our meeting details in the request.
Handling the API Response
def display_meeting_info(): response = create_meeting() join_url = response['join_url'] meeting_password = response['password'] print(f"Your meeting has been created!") print(f"Join URL: {join_url}") print(f"Password: {meeting_password}") # Let's run it! display_meeting_info()
And there you have it! When you run this script, it will create a Zoom meeting and print out the join URL and password. It's that simple!
You've just created your first Zoom meeting using Python and the Zoom API. How cool is that? With this foundation, you can start building more complex applications – maybe a meeting scheduler, or even a custom Zoom client. The possibilities are endless!
Complete Code Example and Running the Script
Alright, let's put it all together! Here's the complete Python script that combines all the steps we've covered. This is your one-stop shop for creating Zoom meetings programmatically:
import jwt import requests import json from time import time # Your Zoom API credentials API_KEY = 'Your_API_Key_Here' API_SEC = 'Your_API_Secret_Here' # Function to generate a JWT token def generate_token(): token = jwt.encode( {'iss': API_KEY, 'exp': time() + 5000}, API_SEC, algorithm='HS256' ) return token # Meeting details meeting_details = { "topic": "Python Zoom Meeting", "type": 2, # Scheduled meeting "start_time": "2023-06-14T10:00:00", # Use your desired date and time "duration": "45", # Duration in minutes "timezone": "America/New_York", "agenda": "Discuss Zoom API Integration", "settings": { "host_video": True, "participant_video": True, "join_before_host": False, "mute_upon_entry": True, "watermark": False, "audio": "voip", "auto_recording": "none" } } # Function to create a meeting def create_meeting(): headers = { 'authorization': 'Bearer ' + generate_token(), 'content-type': 'application/json' } r = requests.post( f'https://api.zoom.us/v2/users/me/meetings', headers=headers, data=json.dumps(meeting_details) ) return r.json() # Function to display meeting info def display_meeting_info(): response = create_meeting() join_url = response['join_url'] meeting_password = response['password'] print(f"Your meeting has been created!") print(f"Join URL: {join_url}") print(f"Password: {meeting_password}") # Run the script if __name__ == "__main__": display_meeting_info()
Running the Script
Now for the moment of truth! Let's run this script and see the magic happen.
Save the above code in a file, let's call it zoom_meeting_creator.py.
Open your terminal or command prompt.
Navigate to the directory where you saved the file.
Run the script by typing:
python zoom_meeting_creator.py
Understanding the Output
When you run the script, you should see output similar to this:
Your meeting has been created! Join URL: https://us04web.zoom.us/j/12345678901?pwd=abcdefghijklmnop Password: 123456
Let's break down what's happening:
The script generates a JWT token for authentication.
It sends a request to Zoom's API to create a meeting with your specified details.
Zoom responds with the meeting information.
The script extracts and displays the Join URL and Password.
The Join URL is what you'd share with participants to join your meeting. The password adds an extra layer of security.
And there you have it! You've just created a Zoom meeting programmatically. Pretty neat, huh?
Remember, this is just the tip of the iceberg. You can expand this script to create multiple meetings, schedule recurring meetings, or even integrate it into a larger application. The possibilities are endless!
Conclusion
Congratulations! You've just unlocked the power of the Zoom API with Python. We've covered everything from setting up your API account to creating meetings with just a few lines of code. This is just the beginning of what you can achieve. Imagine automating your entire meeting schedule or integrating Zoom features into your own apps. The possibilities are endless!
Remember, practice makes perfect. Keep experimenting, and don't be afraid to dive deeper into the Zoom API documentation. Who knows? You might just create the next big Zoom integration that revolutionizes how we connect online.
Congratulations! You've just unlocked the power of the Zoom API with Python. We've covered everything from setting up your API account to creating meetings with just a few lines of code. This is just the beginning of what you can achieve. Imagine automating your entire meeting schedule or integrating Zoom features into your own apps. The possibilities are endless!
Remember, practice makes perfect. Keep experimenting, and don't be afraid to dive deeper into the Zoom API documentation. Who knows? You might just create the next big Zoom integration that revolutionizes how we connect online.
Congratulations! You've just unlocked the power of the Zoom API with Python. We've covered everything from setting up your API account to creating meetings with just a few lines of code. This is just the beginning of what you can achieve. Imagine automating your entire meeting schedule or integrating Zoom features into your own apps. The possibilities are endless!
Remember, practice makes perfect. Keep experimenting, and don't be afraid to dive deeper into the Zoom API documentation. Who knows? You might just create the next big Zoom integration that revolutionizes how we connect online.
FAQs
Why should you choose Qodex.ai?
Why should you choose Qodex.ai?
Why should you choose Qodex.ai?
How can I validate an email address using Python regex?
How can I validate an email address using Python regex?
How can I validate an email address using Python regex?
What is Go Regex Tester?
What is Go Regex Tester?
What is Go Regex Tester?
Remommended posts
Discover, Test, and Secure your APIs — 10x Faster.

Product
All Rights Reserved.
Copyright © 2025 Qodex
Discover, Test, and Secure your APIs — 10x Faster.

Product
All Rights Reserved.
Copyright © 2025 Qodex
Discover, Test, and Secure your APIs — 10x Faster.

Product
All Rights Reserved.
Copyright © 2025 Qodex