Search Blogs
10 Advanced REST API Interview Questions for Developers
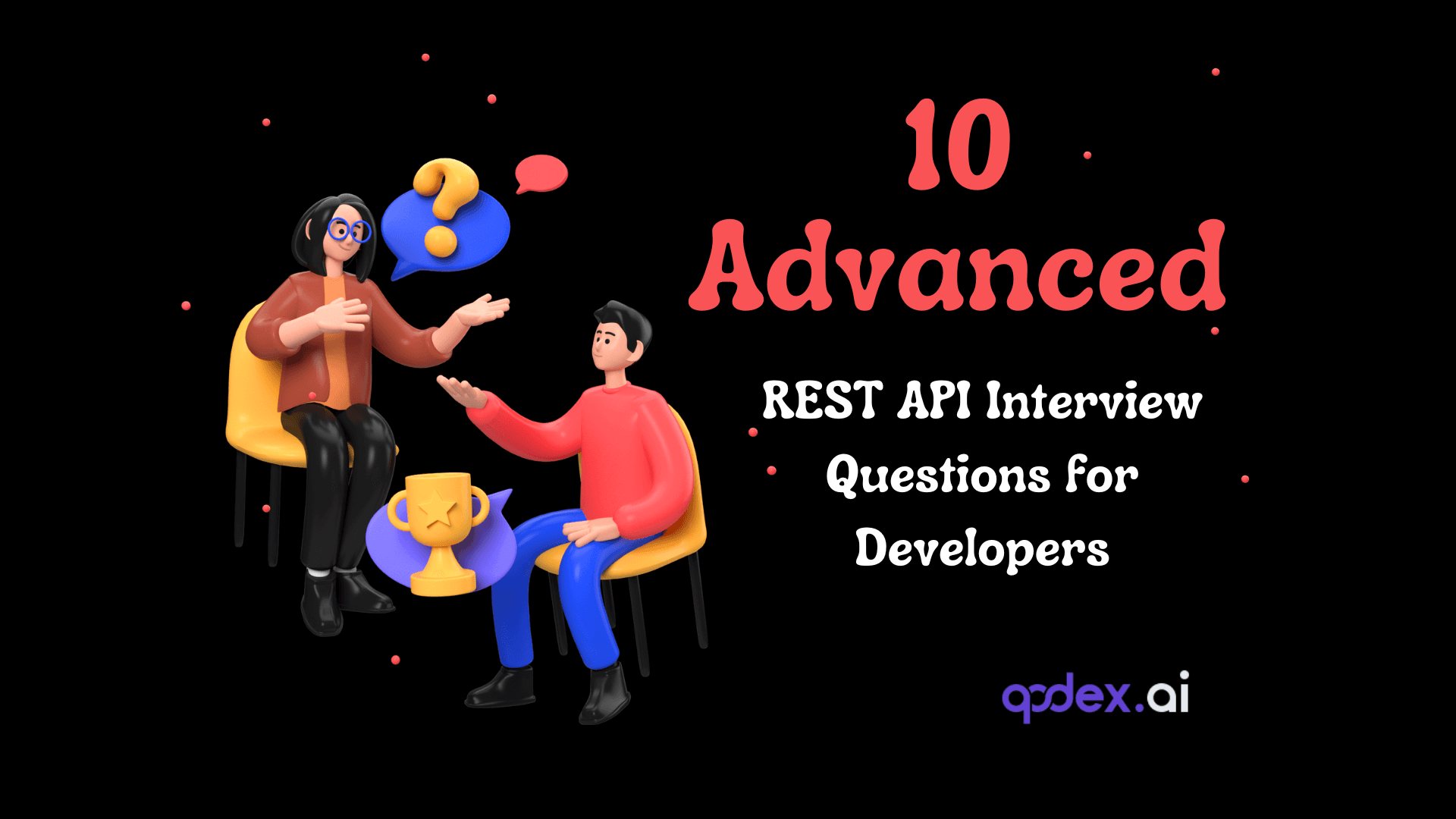
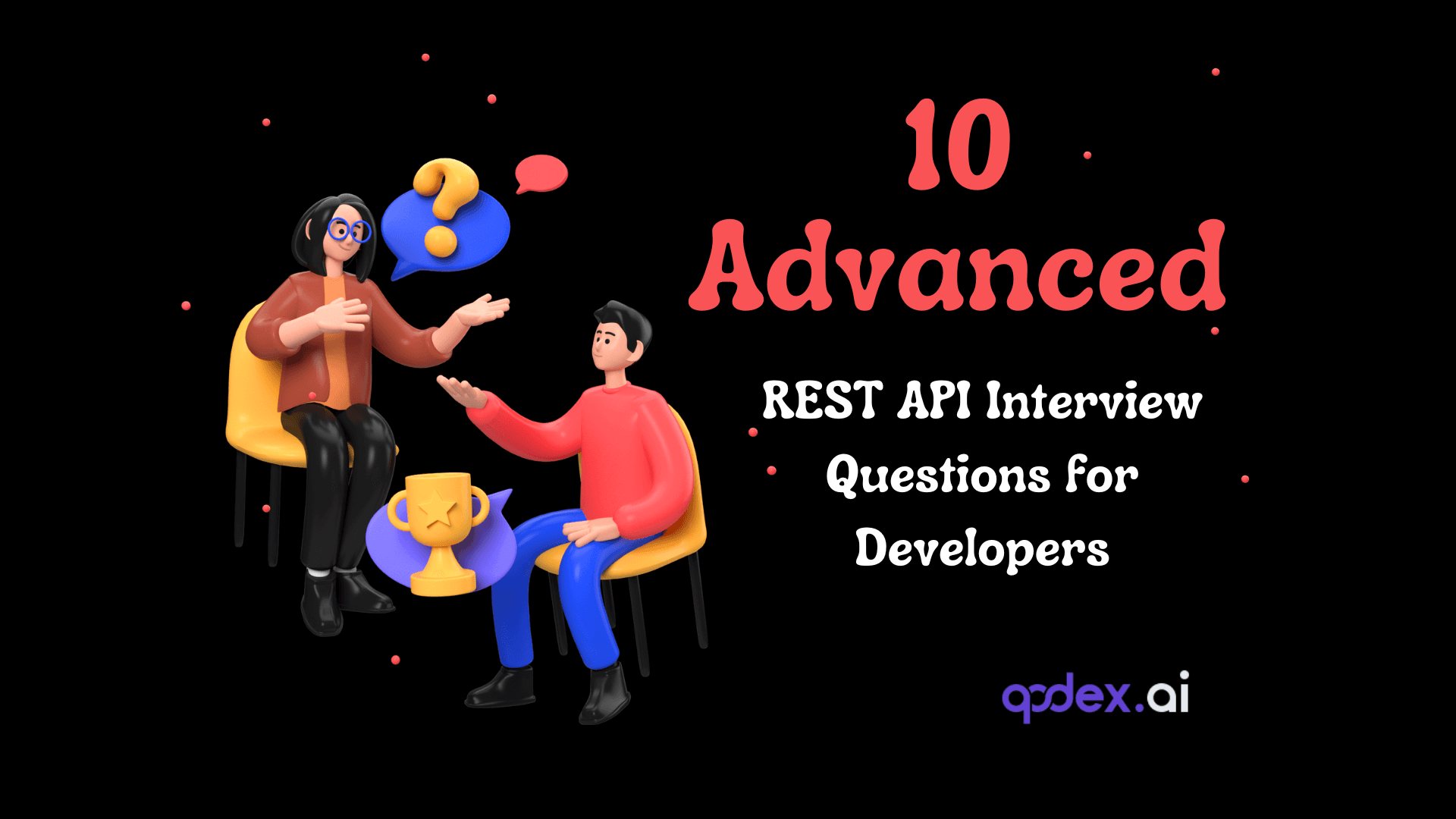
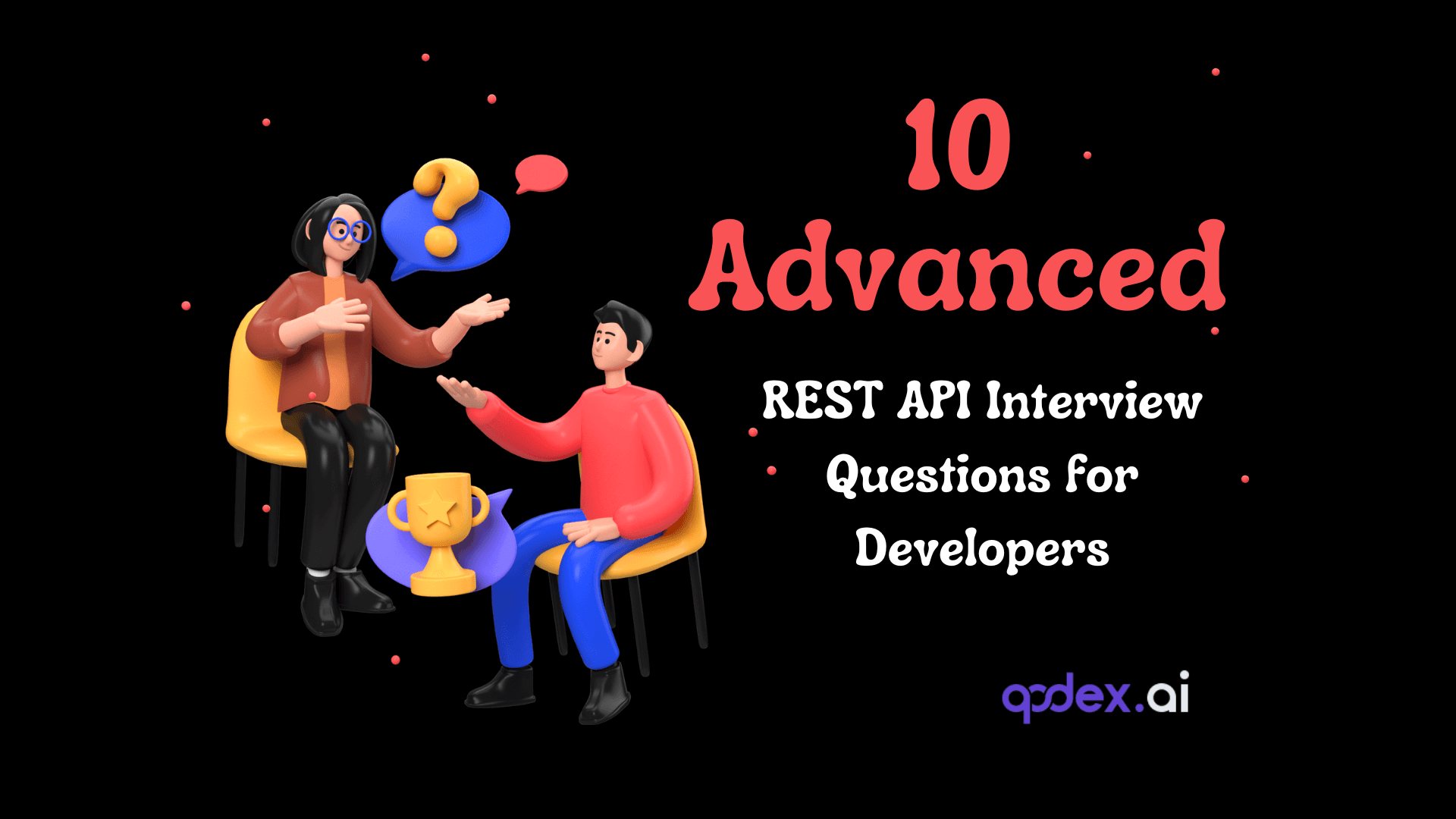
Basic Interview Questions
What do you understand by RESTful Web Services?
RESTful web services follow the REST architecture, using HTTP for communication. They're lightweight, scalable, and facilitate communication between applications in different languages. Clients access server resources via HTTP requests, which include headers, bodies, and receive responses with data and status codes.What is a REST Resource?
A REST Resource in the context of RESTful web services (Representational State Transfer) represents an entity or a collection of entities that can be manipulated through a RESTful API. Each resource is identified by a unique URI (Uniform Resource Identifier) and can be accessed and manipulated using standard HTTP methods.Here are key components of a REST Resource:
URI (Uniform Resource Identifier):
Each resource is accessible via a unique URI. For example, /users might represent a collection of user resources, while /users/1 might represent a specific user.
HTTP Methods:
GET: Retrieve a resource or a collection of resources.
POST: Create a new resource.
PUT: Update an existing resource.
DELETE: Remove a resource.
Representation:
Resources can be represented in various formats, such as JSON, XML, HTML, etc. The client and server negotiate the format through the use of HTTP headers.
Stateless Operations:
Each request from a client to a server must contain all the information the server needs to fulfill the request. The server does not store client context between requests.
CRUD Operations:
REST resources are often manipulated through CRUD operations (Create, Read, Update, Delete).
Example
Consider a REST API for managing a collection of books in a library:GET /books: Retrieve a list of books.
POST /books: Add a new book to the collection.
GET /books/{id}: Retrieve details of a specific book by its ID.
PUT /books/{id}: Update the details of a specific book by its ID.
DELETE /books/{id}: Remove a specific book by its ID.
Each of these endpoints corresponds to a resource (the collection of books or an individual book) and allows for standard HTTP methods to perform operations on those resources.What is URI?
A URI (Uniform Resource Identifier) is a string of characters that identifies a resource on the internet. It provides a way to uniquely identify a resource, such as a web page, a file, or a service.
URIs can take different forms, including URLs (Uniform Resource Locators) and URNs (Uniform Resource Names). URLs specify the location of a resource, while URNs provide a unique name for a resource without specifying its location.
In simpler terms, a URI is like the address of a resource on the internet. Just like a street address helps you find a specific house, a URI helps you locate a specific resource online.What are the features of RESTful Web Services?
Key Features of RESTful Web Services
Statelessness:
Each client request contains all the information needed by the server to fulfill that request. The server does not store any client context between requests. This improves scalability and reliability.
Uniform Interface:
RESTful services follow a consistent, uniform interface, using standard HTTP methods (GET, POST, PUT, DELETE). This simplifies the interaction between clients and servers and ensures a clear separation of concerns.
Resource-Based:
Everything is considered a resource and is identified by a URI (Uniform Resource Identifier). Resources are manipulated using standard HTTP methods, making interactions predictable and consistent.
Representation-Oriented:
Resources are represented in various formats (e.g., JSON, XML, HTML). Clients interact with these representations to perform actions on the resources.
Stateless Operations:
Each operation (request) is stateless, meaning it does not depend on the previous operations. This allows each request to be treated independently, enhancing reliability and scalability.
Cacheability:
Responses from the server are marked as cacheable or non-cacheable, improving efficiency by reducing the need to fetch the same resource multiple times.
Layered System:
RESTful architectures can have multiple layers (e.g., security, load balancing) between the client and the server. Each layer can perform different tasks, improving scalability and manageability.
Code on Demand (Optional):
Servers can temporarily extend or customise client functionality by transferring executable code, such as JavaScript. This is optional and used as needed.
What is the concept of statelessness in REST?
In REST, statelessness means that each client request to the server must contain all the information needed to understand and fulfill the request. The server doesn't store any client state between requests, which simplifies scalability and improves reliability. Each request is independent and self-contained, making it easier to manage and scale the system.What do you understand by JAX-RS?
JAX-RS (Java API for RESTful Web Services) is a Java programming language API that provides support for creating RESTful web services. It simplifies the development of RESTful applications in Java by providing annotations and classes for handling HTTP requests and responses. JAX-RS allows developers to build web services that follow REST principles, making it easier to create scalable and interoperable applications.What are HTTP Status codes?
These are the standard codes that refer to the predefined status of the task at the server. Following are the status codes formats available:1xx - represents informational responses
2xx - represents successful responses
3xx - represents redirects
4xx - represents client errors
5xx - represents server errors
Most commonly used status codes are:200 - success/OK
201 - CREATED - used in POST or PUT methods.
304 - NOT MODIFIED - used in conditional GET requests to reduce the bandwidth use of the network. Here, the body of the response sent should be empty.
400 - BAD REQUEST - This can be due to validation errors or missing input data.
401- UNAUTHORISED - This is returned when there is no valid authentication credentials sent along with the request.
403 - FORBIDDEN - sent when the user does not have access (or is forbidden) to the resource.
404 - NOT FOUND - Resource method is not available.
500 - INTERNAL SERVER ERROR - server threw some exceptions while running the method.
502 - BAD GATEWAY - Server was not able to get the response from another upstream server
What are the HTTP Methods?
The HTTP methods, also known as HTTP verbs, are actions that clients can perform on resources located on a server.
They indicate the desired action to be performed on the specified resource. Here are the common HTTP methods:GET: Retrieves data from the server. It should only retrieve data and should not have any other effect on the server.
POST: Submits data to the server to create a new resource. It often results in a change in state or side effects on the server.
PUT: Updates an existing resource on the server with the provided data. It replaces the entire resource with the new data.
DELETE: Removes the specified resource from the server.
PATCH: Partially updates an existing resource on the server with the provided data. It only updates the specified fields without replacing the entire resource.
HEAD: Retrieves the headers for a resource without the body content. It is often used to check the status of a resource or to retrieve metadata.
OPTIONS: Returns the HTTP methods that the server supports for a specified URL. It is often used for cross-origin resource sharing (CORS) requests.
These HTTP methods allow clients to interact with resources on the server in various ways, enabling a wide range of functionalities in web applications.Advantage and disadvantages of RESTful web services?
Advantages of RESTful Web Services:
Simplicity: Easy to understand and implement with standard HTTP methods.
Scalability: Stateless communication and caching support efficient scaling.
Interoperability: Can be accessed from any platform using standard web protocols.
Flexibility: Support for various data formats, providing flexibility in data representation.
Performance: Lightweight communication and caching mechanisms improve performance.
Disadvantages of RESTful Web Services:
Lack of Standardisation: Variations in implementation may lead to inconsistency.
Security Concerns: Reliance on standard web security mechanisms may not suffice for all needs.
Overhead: Additional HTTP communication may introduce overhead, affecting performance.
Limited Support for Complex Transactions: Not ideal for complex transactions requiring multi-step processes.
Dependency on Network: Susceptible to network latency, failures, and availability issues.
Can you explain the concept of HATEOAS in REST?
HATEOAS (Hypermedia As The Engine Of Application State) is a constraint of the REST application architecture. It means that the client interacts with the application entirely through hypermedia provided dynamically by application servers. In other words, the server provides links to related resources, allowing clients to navigate the API dynamically.
Let's explore how you can establish a comprehensive test infrastructure with Qodex.ai.

With Qodex.ai, you have an AI co-pilot Software Test Engineer at your service. Our autonomous AI Agent assists software development teams in conducting end-to-end testing for both frontend and backend services. This support enables teams to accelerate their release cycles by up to 2 times while reducing their QA budget by one-third.
What do you understand by RESTful Web Services?
RESTful web services follow the REST architecture, using HTTP for communication. They're lightweight, scalable, and facilitate communication between applications in different languages. Clients access server resources via HTTP requests, which include headers, bodies, and receive responses with data and status codes.What is a REST Resource?
A REST Resource in the context of RESTful web services (Representational State Transfer) represents an entity or a collection of entities that can be manipulated through a RESTful API. Each resource is identified by a unique URI (Uniform Resource Identifier) and can be accessed and manipulated using standard HTTP methods.Here are key components of a REST Resource:
URI (Uniform Resource Identifier):
Each resource is accessible via a unique URI. For example, /users might represent a collection of user resources, while /users/1 might represent a specific user.
HTTP Methods:
GET: Retrieve a resource or a collection of resources.
POST: Create a new resource.
PUT: Update an existing resource.
DELETE: Remove a resource.
Representation:
Resources can be represented in various formats, such as JSON, XML, HTML, etc. The client and server negotiate the format through the use of HTTP headers.
Stateless Operations:
Each request from a client to a server must contain all the information the server needs to fulfill the request. The server does not store client context between requests.
CRUD Operations:
REST resources are often manipulated through CRUD operations (Create, Read, Update, Delete).
Example
Consider a REST API for managing a collection of books in a library:GET /books: Retrieve a list of books.
POST /books: Add a new book to the collection.
GET /books/{id}: Retrieve details of a specific book by its ID.
PUT /books/{id}: Update the details of a specific book by its ID.
DELETE /books/{id}: Remove a specific book by its ID.
Each of these endpoints corresponds to a resource (the collection of books or an individual book) and allows for standard HTTP methods to perform operations on those resources.What is URI?
A URI (Uniform Resource Identifier) is a string of characters that identifies a resource on the internet. It provides a way to uniquely identify a resource, such as a web page, a file, or a service.
URIs can take different forms, including URLs (Uniform Resource Locators) and URNs (Uniform Resource Names). URLs specify the location of a resource, while URNs provide a unique name for a resource without specifying its location.
In simpler terms, a URI is like the address of a resource on the internet. Just like a street address helps you find a specific house, a URI helps you locate a specific resource online.What are the features of RESTful Web Services?
Key Features of RESTful Web Services
Statelessness:
Each client request contains all the information needed by the server to fulfill that request. The server does not store any client context between requests. This improves scalability and reliability.
Uniform Interface:
RESTful services follow a consistent, uniform interface, using standard HTTP methods (GET, POST, PUT, DELETE). This simplifies the interaction between clients and servers and ensures a clear separation of concerns.
Resource-Based:
Everything is considered a resource and is identified by a URI (Uniform Resource Identifier). Resources are manipulated using standard HTTP methods, making interactions predictable and consistent.
Representation-Oriented:
Resources are represented in various formats (e.g., JSON, XML, HTML). Clients interact with these representations to perform actions on the resources.
Stateless Operations:
Each operation (request) is stateless, meaning it does not depend on the previous operations. This allows each request to be treated independently, enhancing reliability and scalability.
Cacheability:
Responses from the server are marked as cacheable or non-cacheable, improving efficiency by reducing the need to fetch the same resource multiple times.
Layered System:
RESTful architectures can have multiple layers (e.g., security, load balancing) between the client and the server. Each layer can perform different tasks, improving scalability and manageability.
Code on Demand (Optional):
Servers can temporarily extend or customise client functionality by transferring executable code, such as JavaScript. This is optional and used as needed.
What is the concept of statelessness in REST?
In REST, statelessness means that each client request to the server must contain all the information needed to understand and fulfill the request. The server doesn't store any client state between requests, which simplifies scalability and improves reliability. Each request is independent and self-contained, making it easier to manage and scale the system.What do you understand by JAX-RS?
JAX-RS (Java API for RESTful Web Services) is a Java programming language API that provides support for creating RESTful web services. It simplifies the development of RESTful applications in Java by providing annotations and classes for handling HTTP requests and responses. JAX-RS allows developers to build web services that follow REST principles, making it easier to create scalable and interoperable applications.What are HTTP Status codes?
These are the standard codes that refer to the predefined status of the task at the server. Following are the status codes formats available:1xx - represents informational responses
2xx - represents successful responses
3xx - represents redirects
4xx - represents client errors
5xx - represents server errors
Most commonly used status codes are:200 - success/OK
201 - CREATED - used in POST or PUT methods.
304 - NOT MODIFIED - used in conditional GET requests to reduce the bandwidth use of the network. Here, the body of the response sent should be empty.
400 - BAD REQUEST - This can be due to validation errors or missing input data.
401- UNAUTHORISED - This is returned when there is no valid authentication credentials sent along with the request.
403 - FORBIDDEN - sent when the user does not have access (or is forbidden) to the resource.
404 - NOT FOUND - Resource method is not available.
500 - INTERNAL SERVER ERROR - server threw some exceptions while running the method.
502 - BAD GATEWAY - Server was not able to get the response from another upstream server
What are the HTTP Methods?
The HTTP methods, also known as HTTP verbs, are actions that clients can perform on resources located on a server.
They indicate the desired action to be performed on the specified resource. Here are the common HTTP methods:GET: Retrieves data from the server. It should only retrieve data and should not have any other effect on the server.
POST: Submits data to the server to create a new resource. It often results in a change in state or side effects on the server.
PUT: Updates an existing resource on the server with the provided data. It replaces the entire resource with the new data.
DELETE: Removes the specified resource from the server.
PATCH: Partially updates an existing resource on the server with the provided data. It only updates the specified fields without replacing the entire resource.
HEAD: Retrieves the headers for a resource without the body content. It is often used to check the status of a resource or to retrieve metadata.
OPTIONS: Returns the HTTP methods that the server supports for a specified URL. It is often used for cross-origin resource sharing (CORS) requests.
These HTTP methods allow clients to interact with resources on the server in various ways, enabling a wide range of functionalities in web applications.Advantage and disadvantages of RESTful web services?
Advantages of RESTful Web Services:
Simplicity: Easy to understand and implement with standard HTTP methods.
Scalability: Stateless communication and caching support efficient scaling.
Interoperability: Can be accessed from any platform using standard web protocols.
Flexibility: Support for various data formats, providing flexibility in data representation.
Performance: Lightweight communication and caching mechanisms improve performance.
Disadvantages of RESTful Web Services:
Lack of Standardisation: Variations in implementation may lead to inconsistency.
Security Concerns: Reliance on standard web security mechanisms may not suffice for all needs.
Overhead: Additional HTTP communication may introduce overhead, affecting performance.
Limited Support for Complex Transactions: Not ideal for complex transactions requiring multi-step processes.
Dependency on Network: Susceptible to network latency, failures, and availability issues.
Can you explain the concept of HATEOAS in REST?
HATEOAS (Hypermedia As The Engine Of Application State) is a constraint of the REST application architecture. It means that the client interacts with the application entirely through hypermedia provided dynamically by application servers. In other words, the server provides links to related resources, allowing clients to navigate the API dynamically.
Let's explore how you can establish a comprehensive test infrastructure with Qodex.ai.

With Qodex.ai, you have an AI co-pilot Software Test Engineer at your service. Our autonomous AI Agent assists software development teams in conducting end-to-end testing for both frontend and backend services. This support enables teams to accelerate their release cycles by up to 2 times while reducing their QA budget by one-third.

Ship bug-free software, 200% faster, in 20% testing budget. No coding required

Ship bug-free software, 200% faster, in 20% testing budget. No coding required

Ship bug-free software, 200% faster, in 20% testing budget. No coding required
FAQs
Why should you choose Qodex.ai?
Why should you choose Qodex.ai?
Why should you choose Qodex.ai?
10 Advanced REST API Interview Questions for Developers
Ship bug-free software,
200% faster, in 20% testing budget
Remommended posts

Hire our AI Software Test Engineer
Experience the future of automation software testing.
Copyright © 2024 Qodex
|
All Rights Reserved

Hire our AI Software Test Engineer
Experience the future of automation software testing.
Copyright © 2024 Qodex
All Rights Reserved

Hire our AI Software Test Engineer
Experience the future of automation software testing.
Copyright © 2024 Qodex
|
All Rights Reserved